How do I get the current time in Python?
There are two ways you can get the current time in python.
- using the
datetime
object - using the
time
module
Using the datetime
object
First, you need to import the datetime
module. Then by calling the now
method, you can create a datetime
object containing the current date and time.
from datetime import datetime
now = datetime.now()
current_time = now.strftime("%H:%M:%S")
print(current_time)
This will output:
11:26:11
Using the strftime
you can convert the datetime
to a string by specifying the format.
Using the time
module
First, import the time
module, then call the localtime
method to create a time object.
import time
t = time.localtime()
current_time = time.strftime("%H:%M:%S", t)
print(current_time)
11:26:11
Using the strftime
you can convert the time
to a string by specifying the format.
-
Difference between static and class methods in Python?
Class method To create a class method, use the @classmethod decorator. Class methods receive the class as an implicit first argument, just like an instance method receives the instance. The class m...
Questions -
Understanding slicing in Python?
Slicing is a way of extracting a specific part of an array. The syntax is following: mylist[start:end] # items start through end-1 mylist[start:] # items start through the rest of the array ...
Questions -
How to access the index in for loops in Python?
In python, if you are enumerating over a list using the for loop, you can access the index of the current value by using enumerate function. my_list = [1,2,3,4,5,6,7,8,9,10] for index, value in enu...
Questions -
How to flatten a list in Python?
You can flatten a list in python using the following one-liner: flat_list = [item for sublist in l for item in sublist] In the example above, l is the list of lists that is to be flattened. The pre...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us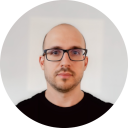
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github