How do I measure elapsed time in Python?
There are several ways to measure elapsed time in Python. Here are a few options:
- You can use the
time
module to get the current time in seconds since the epoch (the epoch is a predefined point in time, usually the beginning of the year 1970). To measure elapsed time, you can get the current time at the start and end of the section of code you want to measure, and then subtract the start time from the end time. Here's an example:
import time
# Code you want to measure goes here
start_time = time.time()
# some code
time.sleep(2)
end_time = time.time()
elapsed_time = end_time - start_time
print(f"Elapsed time: {elapsed_time} seconds")
- Another option is to use the
perf_counter
function from thetimeit
module. This function returns the current performance counter value, which is a measure of elapsed time in seconds with a high precision. Here's an example:
import timeit
start_time = timeit.perf_counter()
# some code
time.sleep(2)
end_time = timeit.perf_counter()
elapsed_time = end_time - start_time
print(f"Elapsed time: {elapsed_time} seconds")
- If you want to measure the time it takes for a specific function to run, you can use the
timeit
module to run the function a certain number of times and return the average time it takes to run. Here's an example:
import timeit
def function_to_time():
# code to be measured goes here
time.sleep(2)
elapsed_time = timeit.timeit(function_to_time, number=1)
print(f"Elapsed time: {elapsed_time} seconds")
-
How do I get the current time in Python?
There are two ways you can get the current time in python. using the datetime object using the time module Using the datetime object First, you need to import the datetime module. Then by calling t...
Questions -
How to convert string into datetime in Python?
To convert a string into a datetime object in Python, you can use the datetime.strptime() function. This function allows you to specify the format of the input string, and it will return a datetime...
Questions -
How do I concatenate two lists in Python?
You can concatenate two lists in Python by using the + operator or the extend() method. Here is an example using the + operator: list1 = [1, 2, 3] list2 = [4, 5, 6] list3 = list1 + list2 print(list...
Questions -
How do I make a time delay in Python?
There are a few ways to make a time delay in Python. Here are three options: time.sleep(): You can use the sleep() function from the time module to add a delay to your program. The sleep() function...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us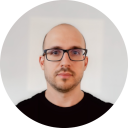
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github