How to start an HTTP server in Python?
You can create an HTTP server in python using the http.server
module.
Starting server from the command line
To start a server from a command line, navigate to your projects directory
cd /dev/myproject
Then start an HTTP server using the following command:
python3 -m http.server
By default, this will run the contents of the directory on a local web server, on port 8000. You can go to this server by going to the URL localhost:8000
in your web browser.
Starting server programmatically
To start an HTTP server from within the python script, you can use socketerserver
along with http.server
. The SimpleHTTPRequestHandler
class can be used in the following manner in order to create a very basic webserver serving files relative to the current directory:
import http.server
import socketserver
PORT = 8000
Handler = http.server.SimpleHTTPRequestHandler
with socketserver.TCPServer(("", PORT), Handler) as httpd:
print("serving at port", PORT)
httpd.serve_forever()
This will run the contents of the directory on a local web server, on port 8000. You can go to this server by going to the URL localhost:8000
in your web browser.
-
Understanding Python super() with init() methods
The super() function is used to call a method from a parent class. When used with the __init__ method, it allows you to initialize the attributes of the parent class, in addition to any attributes ...
Questions -
Importing files from different folder in Python?
In Python, you can use the import statement to import modules or files from different folders. If the file you want to import is in a different folder than the script you are running, you will need...
Questions -
Getting the class name of an instance in Python?
You can use the built-in type() function to get the class name of an instance in Python. For example: class MyClass: pass myinstance = MyClass() print(type(myinstance).name) This will output My...
Questions -
How to remove a key from a Python dictionary?
To remove a key from a dictionary, you can use the del statement. Here's an example: my_dict = {'a': 1, 'b': 2, 'c': 3} del my_dict['b'] print(my_dict) # Output: {'a': 1, 'c': 3} Alternatively, yo...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us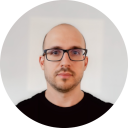
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github