Smoke Testing vs Sanity Testing: Understanding the Key Differences
Software testing is an essential part of the development lifecycle that ensures applications work as expected before they reach users. Among the various testing methodologies, smoke testing and sanity testing are two fundamental approaches that are often confused with each other. Despite their similarities, they serve different purposes and are performed at different stages of development.
This article delves into the definitions, processes, benefits, and limitations of both smoke testing and sanity testing. We'll explore their key differences and how they can work together to create a robust testing strategy.
What is smoke testing?
Smoke testing, also known as "confidence testing," "build verification testing," or "build acceptance testing," is a preliminary testing approach that verifies whether the most critical functions of an application work correctly after a new build.
The main purpose is to identify major issues that would prevent further testing and determine if the build is stable enough to proceed with more comprehensive testing.
The term "smoke testing" originates from hardware testing, where engineers would plug in a circuit board and power it up. If smoke appeared, they would immediately turn off the power, knowing that further testing was unnecessary until the fundamental issue was fixed.
This concept translated well to software testing, where teams needed a quick way to assess whether a build was worth proceeding with further tests.
Smoke testing has become increasingly important with the rise of Agile methodologies and CI/CD pipelines, where frequent builds and deployments are common. It serves as a first line of defense against critical bugs that could waste time and resources on more detailed testing.
How smoke testing works
The smoke testing process typically follows these steps:
- A new feature is developed or an update is made to existing functionality
- A new build is created and deployed to a testing environment
- Smoke tests are executed against the build
- If the tests pass, the build moves to the next testing phase
- If the tests fail, the build is rejected and sent back to development
Smoke tests focus on the core functionalities of an application - the features that are essential for the application to be considered functional. These tests don't go into depth but cover enough breadth to ensure the application isn't fundamentally broken.
Here's an example of what a simple smoke test script might look like for a web application:
import unittest
from selenium import webdriver
class SmokeTest(unittest.TestCase):
def setUp(self):
self.driver = webdriver.Chrome()
self.driver.get("https://example-shop.com")
def test_homepage_loads(self):
# Verify homepage title is correct
self.assertEqual("Example Shop - Home", self.driver.title)
def test_login_form_displayed(self):
# Verify login button exists and can be clicked
login_button = self.driver.find_element_by_id("login-button")
login_button.click()
# Check login form is displayed
login_form = self.driver.find_element_by_id("login-form")
self.assertTrue(login_form.is_displayed())
def test_product_search(self):
# Test basic product search functionality
search_box = self.driver.find_element_by_id("search-input")
search_box.send_keys("laptop")
search_button = self.driver.find_element_by_id("search-button")
search_button.click()
# Verify results are shown
results = self.driver.find_elements_by_class_name("product-item")
self.assertGreater(len(results), 0)
def tearDown(self):
self.driver.quit()
if __name__ == "__main__":
unittest.main()
This simple smoke test script checks three critical functionalities:
- The homepage loads correctly
- The login functionality is accessible
- The product search feature works
For an e-commerce application, these represent essential functions that must work for the application to be considered usable. If any of these tests fail, the build would be rejected, and developers would need to fix the issues before proceeding with more detailed testing.
Benefits and limitations of smoke testing
Benefits
Early detection of critical issues: Smoke testing quickly identifies major problems in a build, allowing teams to address them before investing time in more detailed testing.
Improved development efficiency: By catching fundamental problems early, smoke testing helps development teams focus on fixing critical issues first, rather than wasting time on more detailed testing of an unstable build.
Build quality assurance: Regular smoke testing ensures that each new build meets a minimum level of quality before moving further in the development process.
CI/CD integration: Smoke tests can be easily integrated into CI/CD pipelines, allowing for automated verification of each build.
Time and resource savings: By quickly rejecting unstable builds, smoke testing saves the time and resources that would otherwise be spent on more comprehensive testing of a fundamentally flawed build.
Limitations
Limited coverage: Smoke tests only check the most critical functionalities, potentially missing issues in less essential but still important features.
Maintenance challenges: As an application grows and evolves, smoke tests must be updated to reflect changes in core functionality, which can be time-consuming.
False confidence: Passing smoke tests doesn't guarantee a build is bug-free; it only indicates that the most critical functions appear to work.
Test selection complexity: Determining which functionalities should be included in smoke tests can be challenging, especially for large applications with many features.
What is sanity testing?
Sanity testing, sometimes called "surface level testing," is a focused testing approach that verifies specific functionality or bug fixes in a software build. Unlike smoke testing, which checks the overall stability of a build, sanity testing targets particular areas of an application that have been modified or fixed.
Sanity testing is performed after a build has passed smoke testing and is considered stable enough for more detailed testing. It serves as a quick check to ensure that specific changes work as expected and haven't introduced new issues in the modified components.
How sanity testing works
Sanity testing follows a process similar to smoke testing but with a more targeted focus:
- A build passes smoke testing, indicating overall stability
- Specific changes or bug fixes are identified for verification
- Sanity tests focus only on those specific areas
- If the sanity tests pass, the build moves to more comprehensive testing
- If the sanity tests fail, the build is sent back for further development
Unlike smoke testing, which often involves automated test scripts, sanity testing is typically performed manually by testers or QA professionals. This is because sanity tests are narrowly focused on specific changes and may not justify the effort required to automate them, especially when those changes vary significantly between builds.
Let's consider a sanity testing scenario for our example e-commerce application:
Imagine that developers have fixed a bug in the payment processing system that was causing transactions to fail when users entered certain special characters in the billing address field. A sanity test for this fix might involve:
- Navigating to the checkout page
- Adding items to the cart
- Proceeding to payment
- Entering a billing address with specific special characters
- Completing the transaction
- Verifying that the transaction processes successfully
This test is narrowly focused on the specific issue that was fixed, rather than testing the entire checkout process or other application functionality.
Here's what a manual test script for this sanity test might look like:
Sanity Test: Special Characters in Billing Address
Prerequisites:
- Test account with login credentials
- Test credit card information
Steps:
1. Login to the application using test account
2. Search for "Wireless Headphones" and add to cart
3. Navigate to checkout page
4. Enter shipping information
5. Enter billing address with special characters: "123 Main St., Apt #5, O'Hare District"
6. Complete payment with test credit card
7. Verify order confirmation page is displayed
8. Verify order appears in order history
Expected Result:
- Transaction processes successfully
- No error messages related to special characters
- Order confirmation is displayed with correct billing address
Benefits and limitations of sanity testing
Benefits
Focused verification: Sanity testing concentrates on specific changes or fixes, ensuring they work as expected without spending time on unaffected areas.
Cost-effectiveness: By limiting testing to only the modified components, sanity testing saves time and resources compared to full regression testing.
Increased confidence: Successful sanity tests provide confidence that specific changes have been implemented correctly before proceeding with more comprehensive testing.
Quick feedback: Sanity testing provides rapid feedback on the quality of specific changes, allowing for quick iteration if issues are found.
Limitations
Manual effort: Sanity testing is often performed manually, which can be time-consuming and dependent on tester availability.
Limited automation potential: Because sanity tests focus on specific changes that vary between builds, they can be difficult to automate effectively.
Tester dependency: The effectiveness of sanity testing depends heavily on the tester's understanding of the changes and ability to design appropriate tests.
Narrow focus: By focusing only on specific changes, sanity testing might miss how those changes interact with other parts of the application.
Smoke testing vs sanity testing: key differences
Aspect | Smoke Testing | Sanity Testing |
---|---|---|
Purpose | Verify that critical functionalities of an application work after a new build | Verify that specific changes or bug fixes work as expected in an already validated build |
Goal | Determine if a build is stable enough for further testing | Ensure specific modifications work properly without introducing new issues |
When performed | Immediately after a new build is created | After smoke testing passes, on builds that have shown basic stability |
Scope | Broad but shallow - covers entire application's critical functions | Narrow but deeper - focuses only on specific modified components |
Depth | Surface-level verification of core functionality | More thorough testing of specific areas that changed |
Test execution | Often automated, integrated into CI/CD pipelines | Typically performed manually by testers |
Documentation | Well-documented, structured test cases | Less formal, often created ad-hoc based on specific changes |
Who performs it | Developers, testers, or automated systems | QA professionals or testers with knowledge of specific changes |
Testing approach | Pre-defined test cases covering core functionality | Targeted test cases focused on recent changes |
Test selection | Based on critical functionality of the application | Based on specific changes or bug fixes in the build |
Time requirement | Quick execution to verify basic functionality | Quick but focused on specific components |
Frequency | Performed on every new build | Performed after changes or bug fixes |
Structure | Usually scripted and follows a defined process | Often not scripted, more flexible and specific |
In the testing cycle | First level of testing after build creation | Performed after smoke testing succeeds |
Coverage goal | Essential functionality only | Specific changes only |
While both smoke testing and sanity testing are lightweight testing approaches that help ensure software quality, they differ in several important ways:
Purpose and goals
Smoke testing aims to verify that the entire application is stable enough to proceed with more detailed testing. It checks that all critical functionalities work at a basic level.
Sanity testing focuses on verifying that specific changes or bug fixes work as expected. It ensures that particular modifications haven't introduced new issues in the affected components.
Timing in the testing cycle
Smoke testing is performed immediately after a new build is created, before any other testing takes place.
Sanity testing is performed after smoke testing has passed and is focused on builds that have already demonstrated basic stability.
Scope and coverage
Smoke testing covers the entire application but only checks the most critical functionalities at a surface level.
Sanity testing covers only specific components or features that have been modified, but may test them more thoroughly.
Execution approach
Smoke testing is often automated and integrated into CI/CD pipelines, allowing for consistent verification of each build.
Sanity testing is typically performed manually, as it focuses on specific changes that may vary significantly between builds.
Documentation and structure
Smoke testing is usually well-documented and structured, with defined test cases that are executed consistently for each build.
Sanity testing is often less formal and structured, with test cases that are created or modified based on the specific changes being verified.
Who performs the tests
Smoke testing can be performed by developers, testers, or automated systems as part of the build process.
Sanity testing is typically performed by QA professionals or testers who have a good understanding of the specific changes being verified.
Can they work together?
Smoke testing and sanity testing can and should work together as complementary approaches in a comprehensive testing strategy. Here's how they typically integrate in a software development lifecycle:
- A new build is created.
- Automated smoke tests verify basic functionality and stability.
- If smoke tests pass, sanity tests verify specific changes or fixes.
- If both smoke and sanity tests pass, more comprehensive testing (regression, performance, etc.) is performed.
- If any tests fail, the build is rejected and returned for fixes.
Let's illustrate this with a practical example in a CI/CD pipeline:
stages:
- build
- smoke_test
- sanity_test
- regression_test
- deploy
build_job:
stage: build
script:
- npm install
- npm run build
artifacts:
paths:
- dist/
smoke_test_job:
stage: smoke_test
script:
- npm run smoke-tests
dependencies:
- build_job
sanity_test_job:
stage: sanity_test
script:
- npm run sanity-tests
dependencies:
- build_job
only:
- when: manual
allow_failure: false
regression_test_job:
stage: regression_test
script:
- npm run regression-tests
dependencies:
- build_job
only:
- when: on_success
deploy_job:
stage: deploy
script:
- npm run deploy
dependencies:
- build_job
only:
- when: on_success
In this pipeline configuration:
- The application is built.
- Smoke tests are automatically run to verify basic functionality.
- If smoke tests pass, sanity tests are manually triggered to verify specific changes.
- If both pass, regression tests are automatically run.
- Finally, if all tests pass, the application is deployed.
This integration ensures that each build is verified at multiple levels before proceeding to more comprehensive testing or deployment.
Final thoughts
Smoke testing and sanity testing both play crucial roles in ensuring software quality, despite their different focuses and approaches.
Smoke testing provides a rapid assessment of build stability by verifying critical functionalities, while sanity testing offers targeted verification of specific changes or fixes.
By implementing both in a well-integrated testing strategy, development teams can catch issues early, focus testing efforts effectively, and maintain high software quality throughout the development lifecycle.
Remember that the key to success lies not in choosing one over the other, but in understanding how they complement each other and implementing both appropriately based on your project's specific needs.
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us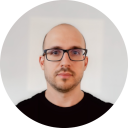
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github