Automating Playwright Checks for User Registration and Login
End-to-end (E2E) testing and continuous monitoring are necessary for maintaining the reliability and performance of key web application processes like user registration and login, email workflows, shopping cart actions, and more.
This approach leverages browser automation techniques to simulate real-world user actions, capturing the full spectrum of interactions a user might have with your application.
By replicating scenarios such as completing a signup form, verifying an email, or navigating through an app, you can preemptively identify and rectify issues that might otherwise disrupt the user experience.
In this guide, we will discuss the role of end-to-end testing in ensuring the reliability of key application workflows, how to set up and execute such tests with Playwright, and how test automation and monitoring with Better Stack supports these goals for the workflows that you are already testing.
Let's get started!
What is Playwright?
Playwright is a powerful end-to-end testing tool designed to work seamlessly across all modern browsers, including Chromium, WebKit, and Firefox. It's built to be cross-platform, allowing tests to run on Windows, Linux, and macOS, whether locally, on CI, in headless mode, or with a UI.
// A simple Playwright test script
import { test, expect } from '@playwright/test';
test('ensure successful user login', async ({ page, browser, context }) => {
const userEmail = 'testmail@example.com'
await page.goto('http://example.com/login');
await page.getByLabel('Email').fill(userEmail);
await page.getByLabel('Password', { exact: true }).fill('123456');
await page.getByRole('button', { name: 'Login' }).click();
await page.waitForLoadState();
await expect(
page.getByRole('heading', { name: 'Welcome to your dashboard!' })
).toBeVisible();
});
Playwright's API is multilingual, supporting TypeScript/JavaScript, Python, .NET, and Java, alongside mobile web emulation for platforms like Android's Google Chrome and iOS's Mobile Safari. It emphasizes test isolation with minimal overhead by utilizing browser contexts and supports state preservation across tests for efficiency.
Key features include an auto-wait functionality that negates the need for manual timeouts, enhancing reliability. It's designed to operate outside of the browser process, facilitating comprehensive testing across multiple tabs, origins, and user scenarios without traditional limitations.
Playwright is also fully equipped with development tools like Codegen for recording actions to generate tests, the Inspector for debugging, and the Trace Viewer for detailed failure diagnostics, making it a comprehensive solution for modern web application testing.
Learn More: Playwright Testing Essentials: A Beginner's Guide
Why E2E is testing important for user registration and login
The registration and login pages are often the first points of interaction between your application and its users. Writing and automating E2E tests for these pages verify that these processes are as seamless and intuitive as possible.
By simulating real-world user interactions, E2E testing uncovers usability problems that could otherwise hinder user engagement or cause frustration. Testing across various browsers and devices can help highlight inconsistencies and browser-specific issues not typically caught in earlier testing stages.
Furthermore, as applications grow and evolve, modifications in one area might unexpectedly impact others. E2E testing guards against such unintended consequences on these essential user pathways, thereby preserving the integrity of the user experience at all times.
User registration and login test cases
A large number of test cases can be written to verify the functionality and reliability of the registration and login processes of an application. Here are a few examples that cover a range of common scenarios:
- Verify the presence and visibility of page elements.
- Test with valid registration and login credentials.
- Test with invalid and empty details.
- Test error handling and displaying of appropriate error messages.
- Test "Remember me" functionality.
- Test password visibility toggle.
- Test login with multi-factor authentication.
- Test email confirmation after successful registration.
- Test social media signup/login integrations
- Test concurrent user sessions.
- Test the effect of multiple login failures.
- Test how accessible your pages are.
- Test the responsiveness of the pages.
- Test login behavior with browser autofill.
- Test CAPTCHA protections against bot signups.
These test cases, when automated with tools like Playwright, provide a comprehensive assessment of the registration and login processes, helping you quickly discover and fix issues when they arise.
Learn More: E2E Testing Signup and Login Workflows with Playwright
Why you should automate your Playwright checks
Automating Playwright tests is crucial for validating the functionality and performance of your application under real-world conditions. By executing these tests in production environments, you can ensure an authentic experience, closely mirroring the user's actual environment, including hardware specifics, network conditions, and third-party integrations.
This approach reveals potential issues that might not surface during development or staging phases, as automated Playwright tests replicate genuine user interactions. Running these tests against production also allows for continuous monitoring of your application's critical workflows, ensuring they operate smoothly for the end-user.
Better Stack lets you run your Playwright checks on a schedule and from multiple locations. You can test your apps every minute, hour, or day and access detailed test statistics and aggregated results.
Its built-in alerting and incident response tools streamline the process of managing potential issues by automatically notifying you when problems are detected to minimize impact on users and maintain high standards of application reliability and user satisfaction.
How often should you run Playwright checks?
The purpose of automated E2E checks is to ascertain your system's health at specific moments. Determining the interval between these checks is crucial; less frequent checks could mean missing out on detecting critical issues in a timely manner, but too frequent checks could also be quite costly.
The optimal frequency for these checks mostly hinges on how tolerable a failure would be if it occurred. Critical operations such as user registration or checking out necessitate more frequent checks while less critical actions like accessing informational pages, could be monitored less frequently, perhaps once an hour.
Adopting a tiered approach based on the severity of potential failures can guide the scheduling of checks. For instance, identifying a problem within five minutes through ten-minute intervals might be acceptable for some processes, but near-instantaneous detection could be crucial for others, necessitating checks every minute or two.
Ultimately, the decision on check frequency should balance the need for timely issue detection against resource constraints and monitoring costs, with a clear focus on maintaining optimal system performance and availability.
Alerting and incident response
Effective incident response hinges on immediate and customizable alerting mechanisms to shine a spotlight on critical issues without overwhelming team members with too many notifications.
Relevant considerations include choosing who gets alerts and when, how the alerts are sent, prioritization based on the nature of the incident, and further escalation if issue acknowledgement isn't forthcoming.
When investigating a problem, having access to the test reports and logs is necessary for diagnosing its cause and ultimately fixing the issue.
With Better Stack, the entire Playwright log and reporting is included in each incident alert so that you can inspect them directly or download and use Playwright's excellent tools for time-travel debugging or other analysis.
Getting started with Playwright and Better Stack
Getting started with Playwright for end-to-end (E2E) testing and Better Stack for monitoring involves a few straightforward steps:
1. Write your Playwright tests
Begin by scripting tests for the functionalities you aim to evaluate. For example, to test the login functionality on Hacker News, your Playwright test could look like this:
import { test, expect } from '@playwright/test';
test('ensure successful login to Hacker News', async ({
page,
browser,
context,
}) => {
await page.goto('https://news.ycombinator.com/login');
await page
.locator('form')
.filter({ hasText: 'username:password: login' })
.locator('input[name="acct"]')
.click();
await page
.locator('form')
.filter({ hasText: 'username:password: login' })
.locator('input[name="acct"]')
.fill('<your_username>'); // Username
await page
.locator('form')
.filter({ hasText: 'username:password: login' })
.locator('input[name="acct"]')
.press('Tab');
await page
.locator('form')
.filter({ hasText: 'username:password: login' })
.locator('input[name="pw"]')
.fill('<your_password>'); // Password
await page.getByRole('button', { name: 'login' }).click();
await page.waitForLoadState();
await expect(page.getByRole('link', { name: '<your_username>' })).toBeVisible();
await expect(page.getByRole('link', { name: 'logout' })).toBeVisible();
});
2. Run your tests locally and in CI/CD
Run your tests in a local setting and integrate them with your CI/CD pipelines to preemptively detect and fix any potential issues. This proactive approach helps avert bugs or other problems from reaching the production stage.
3. Deploy your Playwright tests and start monitoring
With Better Stack, you can schedule your Playwright checks to run on a schedule from different locations against your production systems to keep tabs on your business-critical workflows.
Incorporate your existing test scripts into Better Stack’s scenario editor, then set up the execution timetable, alert configurations, and escalation procedures.
Once you've saved your changes, your monitor will be shown as "Up":
It will shift to "Down" upon test failure, triggering alerts to designated contacts and channels per your settings.
You can then view the ongoing incident to see the details of the failure such as Playwright's entire log and report files for further debugging.
Utilizing Better Stack for Playwright monitoring in this fashion secures a proactive stance on your application's critical functions, facilitating rapid detection and correction of issues before they affect your users.
Final thoughts
In this article, we explored the use of Playwright for end-to-end testing and Better Stack for continuous monitoring, highlighting how easy it can be to keep tabs on your application's critical workflows.
For a deeper dive into how Better Stack enhances your testing and monitoring strategies, check out our comprehensive documentation. If you haven't yet experienced the capabilities of Better Stack, consider signing up for a free account here.
Thanks for reading!
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us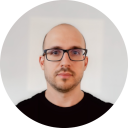
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github