TypeScript developers now have great options for checking whether their data is valid. Two popular choices are TypeBox and Zod. Both help ensure data accuracy, but they work in different ways.
Zod emerged in 2020 and quickly became popular. It checks your data and automatically creates TypeScript types that match. You write your validation rules once and get type safety for free.
TypeBox takes a different path. It focuses on working with JSON Schema, a standard way to describe data formats. TypeBox lets you write schemas that work with JSON Schema tools while still getting proper TypeScript types.
This guide will compare these libraries to help you pick the right one for your projects.
What is Zod?
Zod is a validation library built from the ground up for TypeScript. It lets you define rules for your data and automatically creates matching TypeScript types.
You create Zod schemas using simple functions like z.string()
or z.number()
. You can add rules by chaining methods like .email()
or .min(5)
. This makes your validation rules easy to read and combine.
The best part of Zod is how it handles types. When you write z.infer<typeof mySchema>
, TypeScript gives you a perfect type that matches your validation rules. You don't need to write your types twice.
Many modern tools like Next.js, tRPC, and React Hook Form work well with Zod. It's excellent for both frontend and backend code, especially when you want to share types.
What is TypeBox?
TypeBox is a tool that bridges TypeScript and JSON Schema. It lets you create JSON Schema-compatible validators while getting TypeScript types that match.
The main idea behind TypeBox is compatibility with standard JSON Schema. This means your schemas work with any tool that supports JSON Schema. At the same time, TypeBox gives you functions to get TypeScript types from these schemas.
You write TypeBox schemas using functions like Type.String()
and Type.Number()
. These look similar to TypeScript's own types, making them feel natural to TypeScript developers.
TypeBox is great when you need to work with systems that expect JSON Schema. It's beneficial for APIs where you need both TypeScript types and standard schema documentation.
TypeBox vs. Zod: a quick comparison
Here's how TypeBox and Zod compare on key features:
Feature | TypeBox | Zod |
---|---|---|
Main focus | JSON Schema compatibility | TypeScript-first validation |
Validation | Needs external validator like Ajv | Built-in validation |
Type creation | Generate types from schema | Automatic type inference |
Schema style | JSON Schema-like declarations | Builder pattern with chaining |
Bundle size | Small core + validator size | Medium size, all-inclusive |
Best use case | APIs needing JSON Schema | Full TypeScript projects |
Learning curve | Steeper with JSON Schema concepts | Simpler for TypeScript devs |
Error handling | Depends on validator choice | Built-in error objects |
Framework fits | Backend frameworks, OpenAPI | Next.js, tRPC, React Hook Form |
Schema definition
The way you write validation rules affects how easy they are to read and maintain. TypeBox and Zod take very different approaches here.
TypeBox looks like JSON Schema with TypeScript flavor. You define your types in a way that creates standard JSON Schema:
import { Type, Static } from '@sinclair/typebox'
const UserSchema = Type.Object({
id: Type.Number(),
email: Type.String({ format: 'email' }),
name: Type.String({ minLength: 2 })
})
// Get TypeScript type
type User = Static<typeof UserSchema>
This style works great when you need to share your schemas with tools that expect JSON Schema. Your schemas can be used for validation, documentation, and generating TypeScript types.
Zod takes a more direct approach. It combines schema definition and validation in one package:
import { z } from 'zod'
const userSchema = z.object({
id: z.number(),
email: z.string().email(),
name: z.string().min(2)
})
// Get TypeScript type
type User = z.infer<typeof userSchema>
Zod's style is shorter and doesn't need any external tools. You get everything in one place - from defining rules to validating data to generating types. This makes it quick to set up and easy to use.
The main difference is what they prioritize. TypeBox puts JSON Schema compatibility first, while Zod focuses on being a complete TypeScript solution. Your choice depends on whether you need to work with JSON Schema or just want simple validation.
Validation patterns
How you actually check data against your schemas affects your code structure. TypeBox and Zod handle this very differently.
TypeBox focuses on defining schemas, not running validation. You need to use another library like Ajv to actually check data:
import { Type } from '@sinclair/typebox'
import Ajv from 'ajv'
const UserSchema = Type.Object({
email: Type.String({ format: 'email' })
})
const ajv = new Ajv()
const validate = ajv.compile(UserSchema)
// Check data
const valid = validate(data)
if (!valid) {
console.log(validate.errors)
}
This separation gives you freedom to pick the best validator for your needs. Ajv is very fast, but you need to set it up separately and handle type assertions yourself.
Zod bundles everything together. It has built-in methods to validate data:
import { z } from 'zod'
const userSchema = z.object({
email: z.string().email()
})
try {
// Validate and get typed data
const user = userSchema.parse(data)
// Use user safely
} catch (error) {
console.log(error.errors)
}
Zod also has a non-throwing option if you prefer:
const result = userSchema.safeParse(data)
if (result.success) {
// Use result.data
} else {
console.log(result.error)
}
The key difference is simplicity versus flexibility. Zod gives you a complete package out of the box. TypeBox lets you pick your validation tools but requires more setup.
Handling complex validation scenarios
Real apps often need more than basic type checking. You might need to check that passwords match or validate against data in your database. Let's see how each library handles these complex cases.
TypeBox works with JSON Schema features and lets you add custom validation through your validator:
import { Type } from '@sinclair/typebox'
import Ajv from 'ajv'
const ajv = new Ajv()
// Add custom password check
ajv.addKeyword({
keyword: 'passwordStrength',
validate: (schema, password) => {
return /[A-Z]/.test(password) &&
/[a-z]/.test(password) &&
/[0-9]/.test(password)
}
})
const PasswordSchema = Type.Object({
password: Type.String({
minLength: 8,
passwordStrength: true
})
})
This approach is powerful for reusable validation rules. You can define custom keywords once and use them in many schemas. The downside is the extra setup and the need to learn both TypeBox and your validator.
Zod takes a more direct approach with built-in methods:
import { z } from 'zod'
const passwordSchema = z.string()
.min(8)
.refine(password => {
const hasUpper = /[A-Z]/.test(password)
const hasLower = /[a-z]/.test(password)
const hasNumber = /[0-9]/.test(password)
return hasUpper && hasLower && hasNumber
}, { message: "Password too weak" })
For async validation, like checking if a username is taken:
const usernameSchema = z.string().refineAsync(
async (name) => {
const isTaken = await checkDatabase(name)
return !isTaken
},
{ message: "Username already taken" }
)
Zod's approach puts all validation logic in one place. This makes it easier to understand at a glance, but might be less reusable between schemas.
Your choice depends on what you need. If you have complex rules used across many schemas, TypeBox and custom keywords might work better. For simpler project-specific validation, Zod's direct approach is often easier.
Type safety and integration
Both libraries help you get accurate TypeScript types from your validation rules, but they approach it differently.
TypeBox creates a bridge between JSON Schema and TypeScript with its Static<>
helper:
import { Type, Static } from '@sinclair/typebox'
const UserSchema = Type.Object({
id: Type.Number(),
email: Type.String(),
age: Type.Optional(Type.Number())
})
// Generate TypeScript type
type User = Static<typeof UserSchema>
TypeBox carefully maps JSON Schema concepts to TypeScript types. This ensures your generated types accurately reflect the validation rules in your schema.
One strength of TypeBox is handling complex type patterns:
const ResponseSchema = Type.Union([
Type.Object({
success: Type.Literal(true),
data: UserSchema
}),
Type.Object({
success: Type.Literal(false),
error: Type.String()
})
])
type Response = Static<typeof ResponseSchema>
Zod has its own .infer<>
helper that pulls types directly from schemas:
import { z } from 'zod'
const userSchema = z.object({
id: z.number(),
email: z.string(),
age: z.number().optional()
})
// Generate TypeScript type
type User = z.infer<typeof userSchema>
The big advantage of Zod is that validation automatically narrows types:
function processUser(data: unknown) {
const user = userSchema.parse(data)
// TypeScript knows user is User type here
return user.id
}
Both libraries work well with TypeScript, but Zod's approach is more seamless. With TypeBox, you need to manually tell TypeScript about types after validation. With Zod, this happens automatically when you validate data.
Ecosystem and maturity
The tools and community around a library affect how useful it is in real projects. TypeBox and Zod fit into different parts of the TypeScript ecosystem.
TypeBox works best with JSON Schema tools. It's a great choice for APIs that need to generate documentation or work with OpenAPI specs. TypeBox fits well with backend frameworks like Fastify and NestJS:
import fastify from 'fastify'
import { Type } from '@sinclair/typebox'
const app = fastify()
app.post('/users', {
schema: {
body: Type.Object({
username: Type.String(),
email: Type.String({ format: 'email' })
})
}
}, (request, reply) => {
// Handler with typed request body
})
TypeBox benefits from the wide adoption of JSON Schema. You can use TypeBox schemas with API docs tools, form generators, and other JSON Schema validators.
Zod thrives in full-stack TypeScript projects. It's popular with modern frameworks like tRPC, Next.js, and React Hook Form:
import { initTRPC } from '@trpc/server'
import { z } from 'zod'
const t = initTRPC.create()
const appRouter = t.router({
createUser: t.procedure
.input(z.object({
username: z.string(),
email: z.string().email()
}))
.mutation(({ input }) => {
// Create user with typed input
})
})
Zod is great when you want to share types between frontend and backend. It helps create end-to-end type safety in your applications.
Both libraries are actively maintained, but they serve different needs. TypeBox is best for APIs that need to work with JSON Schema. Zod is ideal for TypeScript applications where you want simple validation and shared types.
Performance considerations
How quickly validation runs matters, especially in busy APIs or complex apps. TypeBox and Zod have different performance profiles.
TypeBox itself doesn't handle validation. When paired with Ajv, it's extremely fast:
import { Type } from '@sinclair/typebox'
import Ajv from 'ajv'
const ajv = new Ajv()
const schema = Type.Object({ /* fields */ })
const validate = ajv.compile(schema)
// Ajv is very fast
function validateItem(item) {
return validate(item)
}
Ajv compiles schemas to optimized JavaScript functions. This makes TypeBox + Ajv much faster than other validators, sometimes 10x faster for complex schemas.
Zod handles validation internally:
import { z } from 'zod'
const schema = z.object({ /* fields */ })
function validateItem(item) {
return schema.safeParse(item)
}
Zod focuses on developer experience over raw speed. It's fast enough for most apps, but not as fast as Ajv for high-volume validation.
Bundle size also differs. TypeBox is small, but you need to add your validator. Zod includes everything in one package, making it bigger. For web apps, this might matter.
For most projects, both are fast enough. Performance should only be a deciding factor if you handle lots of validation or have complex schemas.
Final thoughts
TypeBox and Zod are both great tools for validating data in TypeScript, but they’re built for different jobs. TypeBox is a solid choice if you need to work with JSON Schema, especially when building APIs, generating OpenAPI docs, or using tools that rely on standard schemas.
It also offers top-notch performance. On the other hand, Zod is easier to use and works well for full-stack apps. It’s a go-to if you use frameworks like Next.js, tRPC, or React Hook Form. Both help catch data issues early, but the right pick depends on your project and the tools you’re using.
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us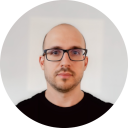
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github