When you're working with data, bad data can lead to bugs, security issues, and unhappy users. To avoid that, you need to make sure your data is correct. Two popular tools for this are Joi and TypeBox. They both help you validate your data, but they do it in different ways.
Joi has been around for a long time. It's reliable, well-tested, and used in many JavaScript projects. TypeBox is newer and built with TypeScript in mind. It helps you check your data at runtime while working well with TypeScript’s static types.
In this guide, you'll see how Joi and TypeBox compare so you can decide which one fits your project best.
What is Joi?
Joi started as part of the Hapi.js framework back when Node.js was gaining popularity. Developers loved it because it made data validation intuitive and powerful.
You validate data in Joi by creating schemas - blueprints that describe what your data should look like. Even though Joi predates TypeScript, it works well in TypeScript projects thanks to type definitions.
It's widely used in Express apps, REST APIs, and many Node.js projects where reliable validation matters.
What is TypeBox?
TypeBox is a newer tool explicitly built for TypeScript developers. It creates JSON Schemas that work well with TypeScript's type system.
The big idea behind TypeBox is simple: define your validation schema once, and get both runtime checks and TypeScript types from the same definition.
TypeBox generates standard JSON Schema, which you can use with fast validators like Ajv. It's lightweight and fits naturally into TypeScript workflows.
TypeBox vs. Joi: a quick comparison
Here's how these two libraries compare at a glance:
Feature | Joi | TypeBox |
---|---|---|
Focus | JavaScript-first with TypeScript support | TypeScript-first design |
Validation style | All-in-one validation library | JSON Schema generator (needs Ajv) |
Schema syntax | Fluent, chainable API | Object composition style |
TypeScript integration | Separate type definitions | Automatic type inference |
Learning curve | Easy for JavaScript developers | Easy for TypeScript developers |
Bundle size | Larger (~70KB minified) | Smaller (~5KB minified) |
Maturity | Mature, stable API | Newer but rapidly maturing |
Community | Large, established community | Growing TypeScript community |
Framework support | Most Node.js frameworks | TypeScript-focused frameworks |
Schema definition
When working with data, the first step is defining what "valid" looks like. Joi and TypeBox let you create schemas for this, but they take different paths.
Joi uses chains of methods that build on each other. This creates readable rules that flow naturally:
const productSchema = Joi.object({
name: Joi.string().min(2).required(),
price: Joi.number().positive().precision(2),
tags: Joi.array().items(Joi.string())
});
const result = productSchema.validate(someData);
This style feels natural, especially for JavaScript developers. The downside? In TypeScript projects, you need to define your types separately from your validation logic.
TypeBox takes a more structured approach. It builds schemas as nested objects that mirror TypeScript types:
const Product = Type.Object({
name: Type.String({ minLength: 2 }),
price: Type.Number({ minimum: 0, multipleOf: 0.01 }),
tags: Type.Array(Type.String())
});
const validate = ajv.compile(Product);
The real magic happens when you extract TypeScript types from your schema:
type ProductType = Static<typeof Product>;
// Now you have both validation and types!
This tight integration prevents mismatches between your runtime checks and compile-time types. You define your rules once, and both systems stay in sync.
Validation patterns
Once you've defined your schema, the next step is actually validating your data. Joi and TypeBox handle this in different ways, each with its own strengths.
Joi keeps things self-contained. When you validate data, you get back both the results and any errors:
const { error, value } = userSchema.validate(input);
if (error) {
console.log('Invalid data:', error.message);
} else {
saveUser(value); // Data is valid and normalized
}
This approach makes error handling straightforward. You don't need try/catch blocks, and you can easily format error messages for users.
TypeBox works differently. It generates JSON Schema, but you need a validator like Ajv to actually check data:
import Ajv from 'ajv';
const ajv = new Ajv();
const validate = ajv.compile(UserSchema);
if (validate(input)) {
saveUser(input); // Data is valid
} else {
console.log('Invalid data:', validate.errors);
}
This separation of concerns keeps TypeBox focused on schema generation, while letting Ajv handle the validation details. The upside? Ajv is incredibly fast and standards-compliant.
Both approaches work well, but they fit into different development styles. Joi's all-in-one approach feels more cohesive, while TypeBox's modular design aligns better with TypeScript projects and JSON Schema standards.
Handling complex validation scenarios
Not all validation is simple. Sometimes you need rules that depend on other fields, custom checks, or different logic based on the data. Both Joi and TypeBox can handle these cases.
Joi excels at expressing complex rules. It has built-in methods for conditions, cross-field validation, and custom logic:
const orderSchema = Joi.object({
type: Joi.string().valid('standard', 'express').required(),
items: Joi.array().min(1).required(),
insurance: Joi.when('type', {
is: 'express',
then: Joi.boolean().required(),
otherwise: Joi.boolean().optional()
})
});
The .when()
method makes conditional validation clear and concise. Joi also lets you add custom validation with the .custom()
method:
const schema = Joi.string().custom((value, helpers) => {
if (isBlacklisted(value)) {
return helpers.error('string.forbidden');
}
return value;
});
TypeBox approaches complex validation through JSON Schema features and composition. For conditional validation, you might use type unions:
const StandardOrder = Type.Object({
type: Type.Literal('standard'),
items: Type.Array(Type.Object({}), { minItems: 1 }),
insurance: Type.Optional(Type.Boolean())
});
const ExpressOrder = Type.Object({
type: Type.Literal('express'),
items: Type.Array(Type.Object({}), { minItems: 1 }),
insurance: Type.Boolean()
});
const Order = Type.Union([StandardOrder, ExpressOrder]);
For custom validation, TypeBox works with Ajv keywords and formats:
ajv.addKeyword({
keyword: 'notBlacklisted',
validate: (schema, data) => !isBlacklisted(data)
});
const SafeString = Type.String({ notBlacklisted: true });
Joi offers a more compact syntax for complex rules, while TypeBox provides a more composable system that aligns with TypeScript's type composition.
Type safety and integration
Type safety is a big deal in modern development, especially if you use TypeScript. Joi and TypeBox take very different approaches here.
Joi was built before TypeScript became popular. It supports TypeScript through type definitions, but you must manually keep your schemas and types in sync:
import Joi from 'joi';
// Validation schema
const userSchema = Joi.object({
name: Joi.string().required(),
email: Joi.string().email(),
age: Joi.number().min(18)
});
// Separate TypeScript interface
interface User {
name: string;
email: string;
age: number;
}
function processUser(data: unknown): User {
const { error, value } = userSchema.validate(data);
if (error) throw error;
return value as User;
}
This works, but it creates two sources of truth. If you change the schema, you must remember to update the TypeScript interface too.
TypeBox was designed specifically to solve this problem. It generates both validation schemas and TypeScript types from a single definition:
import { Type, Static } from '@sinclair/typebox';
const UserSchema = Type.Object({
name: Type.String(),
email: Type.String({ format: 'email' }),
age: Type.Number({ minimum: 18 })
});
// TypeScript type automatically derived from schema
type User = Static<typeof UserSchema>;
function processUser(data: unknown): User {
const validate = ajv.compile(UserSchema);
if (!validate(data)) throw new Error('Invalid data');
return data as User;
}
This approach eliminates type mismatches. Your validation logic and TypeScript types always stay in sync because they come from the same source.
TypeBox also preserves TypeScript's literal types, unions, and other advanced features:
const Role = Type.Union([
Type.Literal('admin'),
Type.Literal('user'),
Type.Literal('guest')
]);
// Becomes the type: 'admin' | 'user' | 'guest'
type UserRole = Static<typeof Role>;
If type safety matters to your project, TypeBox's integrated approach offers clear advantages. For teams less focused on TypeScript, Joi's separation of concerns might feel more flexible.
Ecosystem and maturity
When choosing a validation library, it’s not just about the code—it’s also about how well it fits into your stack and how much support it has around it.
Joi has been around since 2012 and has a mature ecosystem. It works with most Node.js frameworks out of the box:
// Express + Joi using celebrate middleware
app.post('/users', celebrate({
body: userSchema
}), createUser);
Many plugins extend Joi's functionality. You'll find extensions for dates, phone numbers, credit cards, and more. Its API has remained stable for years, making upgrades painless.
TypeBox is newer but growing quickly in the TypeScript community. It works especially well with TypeScript-focused frameworks:
// Fastify with TypeBox
app.post<{ Body: UserType }>('/users', {
schema: { body: UserSchema },
handler: createUser
});
TypeBox benefits from the broader JSON Schema ecosystem. Any tool that works with JSON Schema works with TypeBox schemas. This includes documentation generators, API validators, and client libraries.
Joi makes sense for established Node.js applications, especially JavaScript-first projects. TypeBox fits better in TypeScript environments where type safety is a priority, particularly for full-stack applications that share types between frontend and backend.
Performance considerations
Performance can be a deciding factor, especially if you're building high-traffic APIs or processing large volumes of data.
Joi offers solid performance thanks to years of optimization. It compiles schemas internally and caches results:
// Optimize validation performance
const schema = Joi.object({
// schema definition
}).options({ abortEarly: true });
TypeBox itself is extremely lightweight. The heavy lifting happens in Ajv, which is known for its blazing speed:
// Configure Ajv for maximum performance
const ajv = new Ajv({ allErrors: false });
const validate = ajv.compile(UserSchema);
In benchmarks, TypeBox with Ajv typically outperforms Joi, especially for simpler schemas and large data sets. The performance gap narrows for complex validation rules with many conditions.
For most web applications, either library is fast enough. Choose based on your other requirements like TypeScript integration or ecosystem support. For high-traffic APIs or performance-sensitive applications, TypeBox with Ajv offers better raw speed.
Final thoughts
Joi and TypeBox both validate data but with different focuses. Joi is a mature, all-in-one tool that suits JavaScript and traditional Node.js projects, though it doesn’t fully integrate with TypeScript types.
TypeBox is built for TypeScript, keeping validation and types in sync while supporting fast tools like Ajv. It’s ideal for modern TypeScript apps that share types across the stack.
Choose TypeBox for stronger type safety, or stick with Joi if you're working mainly in JavaScript or already using it.
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us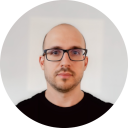
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github