Need to pick between Knex and Prisma for your next JavaScript project? Let's break down these two popular database tools.
Knex.js gives you a SQL query builder with chainable methods that work across different databases. You get direct control while avoiding raw SQL strings.
Prisma takes a different path with a modern, type-safe ORM approach. It generates code from your schema definition and lets you work with database records as regular JavaScript objects.
I'll compare these tools across key areas so you can make the right choice for your specific needs.
What is Knex?
Knex.js stands out as a mature SQL query builder that works with PostgreSQL, MySQL, SQLite, Oracle, and MSSQL. Since its creation in 2013, developers have relied on its flexibility across different database systems.
You get a practical toolkit with Knex - a chainable API for writing queries, built-in transaction handling, connection pooling, and migration tools. It strikes a balance between abstraction and control, letting you build SQL queries with JavaScript methods without hiding the underlying SQL concepts.
Unlike full ORMs, Knex doesn't try to map your database tables to JavaScript classes. It focuses on translating your JavaScript code into proper SQL queries that work the same way across any supported database.
What is Prisma?
Prisma entered the scene in 2019 as a fresh take on database tools. At its core sits a schema file where you define your data model - Prisma uses this to generate a tailored, type-safe client for your database.
The Prisma ecosystem combines three main tools: Prisma Client for queries, Prisma Migrate for database changes, and Prisma Studio for visual data management. This integrated approach aims to make your database work more straightforward.
Prisma breaks from traditional ORMs by avoiding model instances with methods. Instead, you get a generated client that lets you work with plain JavaScript objects while enforcing your schema's types and relationships automatically.
Knex vs Prisma: a quick comparison
Choosing between Knex and Prisma affects your development experience, application architecture, and how you interact with your database. Each tool approaches database access with different priorities and design philosophies.
The following comparison highlights key differences to consider:
Feature | Knex.js | Prisma |
---|---|---|
Primary paradigm | SQL query builder | Schema-first ORM |
Learning curve | Moderate, SQL knowledge required | Gentle, minimal SQL knowledge needed |
Type safety | Limited, relies on external types | Strong, auto-generated TypeScript types |
Query building | Chainable methods for SQL construction | High-level API with relation handling |
Schema definition | Code-first with migrations | Schema-first with Prisma Schema Language |
Migration support | Built-in migrations system | Prisma Migrate with declarative schema |
Relationship handling | Manual join queries | Automatic relation loading and nested queries |
Transaction management | Explicit transaction blocks | Nested writes with automatic transactions |
Performance | Lightweight with minimal overhead | Higher abstraction with some performance cost |
Database support | Wide support including PostgreSQL, MySQL, SQLite | PostgreSQL, MySQL, SQLite, SQL Server, MongoDB |
Raw SQL support | First-class raw query support | Support for raw queries when needed |
Type hints | Via third-party types | Auto-generated TypeScript types |
Ecosystem | Mature with established patterns | Modern, growing ecosystem with dedicated tools |
Data inspection | No built-in tools | Prisma Studio for visual data management |
Maintenance approach | Stable, conservative changes | Rapid development, frequent improvements |
Installation and setup
Getting started reveals key differences between these tools. Each takes its own path to connecting your app with your database.
Knex keeps things traditional - you install the package and its database driver, then set up your connections with JavaScript:
// Install packages
npm install knex pg
// knexfile.js - your configuration
module.exports = {
development: {
client: 'postgresql',
connection: {
database: 'my_db',
user: 'username',
password: 'password'
},
migrations: {
directory: './migrations'
}
}
};
// In your app
const knex = require('knex')(require('./knexfile').development);
You handle database settings directly with Knex, giving you control but requiring more initial setup. You decide exactly how connections work.
Prisma takes over more of this process with its schema-first approach:
# Install packages
npm install prisma --save-dev @prisma/client
npx prisma init
This creates a schema file where you define your data model:
// prisma/schema.prisma (simplified)
datasource db {
provider = "postgresql"
url = env("DATABASE_URL")
}
generator client {
provider = "prisma-client-js"
}
model User {
id Int @id @default(autoincrement())
email String @unique
name String?
posts Post[]
}
model Post {
id Int @id @default(autoincrement())
title String
author User @relation(fields: [authorId], references: [id])
authorId Int
}
After defining your schema, generate your client and use it:
// Generate client first with: npx prisma generate
// In your app
const { PrismaClient } = require('@prisma/client');
const prisma = new PrismaClient();
// Now use prisma for database operations
const users = await prisma.user.findMany();
Prisma guides you along a more structured path. This means less configuration work but requires following Prisma's way of doing things.
Model definition
Database modeling reveals fundamental differences between these tools. Your choice here affects how you'll work with your data throughout your project.
Knex doesn't have traditional models - instead, you define your database structure through migrations:
// migrations/create_users_table.js
exports.up = function(knex) {
return knex.schema.createTable('users', table => {
table.increments('id').primary();
table.string('email').notNullable().unique();
table.string('name');
table.timestamp('created_at').defaultTo(knex.fn.now());
});
};
exports.down = function(knex) {
return knex.schema.dropTable('users');
};
Run these migrations with npx knex migrate:latest
to set up your database. Since Knex doesn't create models for you, many developers build their own wrappers:
// A simple DIY model with Knex
class User {
static async findById(id) {
return knex('users').where({ id }).first();
}
static async create(data) {
return knex('users').insert(data).returning('*');
}
}
This DIY approach gives you freedom but means writing and maintaining your own data access patterns.
Prisma works completely differently with its schema-first approach:
// prisma/schema.prisma
model User {
id Int @id @default(autoincrement())
email String @unique
name String?
posts Post[]
}
model Post {
id Int @id @default(autoincrement())
title String
author User @relation(fields: [authorId], references: [id])
authorId Int @map("author_id")
}
From this schema, Prisma generates a complete client with type-safe operations:
// Using the generated client
import { PrismaClient } from '@prisma/client';
const prisma = new PrismaClient();
// Create a user with posts in one operation
const user = await prisma.user.create({
data: {
email: 'alice@example.com',
name: 'Alice',
posts: {
create: [{ title: 'Hello World' }]
}
},
include: { posts: true }
});
Prisma's approach gives you a central schema that drives everything else. Your editor can provide autocompletion, and TypeScript can catch errors before runtime.
Query building
Writing database queries shows the core philosophy of each tool. Your daily coding experience will be shaped by these different approaches.
Knex gives you a chainable API that feels like writing SQL in JavaScript:
// Basic query
const users = await knex('users')
.select('id', 'name')
.where('active', true)
.orderBy('name');
// Join example
const userPosts = await knex('users')
.join('posts', 'users.id', 'posts.author_id')
.select('users.name', 'posts.title')
.where('posts.published', true);
// Aggregation
const postCounts = await knex('posts')
.select('author_id')
.count('* as post_count')
.groupBy('author_id')
.having('count(*)', '>', 5);
This approach keeps you close to SQL concepts while avoiding string concatenation. You can build exactly the query you need, making it perfect for complex or performance-critical operations.
Prisma offers a higher-level API focused on your data models:
// Basic query
const users = await prisma.user.findMany({
select: { id: true, name: true },
where: { active: true },
orderBy: { name: 'asc' }
});
// Relation query (join)
const userPosts = await prisma.post.findMany({
where: { published: true },
select: {
title: true,
author: { select: { name: true } }
}
});
// Aggregation
const postCounts = await prisma.post.groupBy({
by: ['authorId'],
_count: { _all: true },
having: { _count: { _all: { gt: 5 } } }
});
Prisma abstracts away SQL details in favor of an object-based approach. This makes common operations more intuitive but requires learning Prisma's specific patterns for complex queries.
Transaction management
Transactions keep your data consistent when multiple operations need to succeed or fail together. The approaches to transaction handling reveal key differences between these tools.
Knex puts you in direct control of transactions with a callback or Promise-based API:
// Using the callback approach
await knex.transaction(async trx => {
// Create a user and get their ID
const [userId] = await trx('users')
.insert({ name: 'Alice', email: 'alice@example.com' })
.returning('id');
// Create a post for this user
await trx('posts').insert({
title: 'First Post',
author_id: userId
});
// Auto-commits if successful, rolls back on error
});
// Or the manual approach
const trx = await knex.transaction();
try {
const [userId] = await trx('users')
.insert({ name: 'Bob', email: 'bob@example.com' })
.returning('id');
await trx('posts').insert({ title: 'Bob\'s Post', author_id: userId });
await trx.commit();
} catch (error) {
await trx.rollback();
throw error;
}
With Knex, you decide exactly how transactions work and when they commit or roll back.
Prisma handles many transaction scenarios automatically, especially when working with related records:
// Automatic transaction for related records
const result = await prisma.user.create({
data: {
name: 'Carol',
email: 'carol@example.com',
posts: {
create: [
{ title: 'Carol\'s First Post' },
{ title: 'Carol\'s Second Post' }
]
}
},
include: { posts: true }
});
// This happens in a transaction automatically
// Explicit transaction for custom flows
const [user, post] = await prisma.$transaction(async (tx) => {
const user = await tx.user.create({
data: { name: 'Dave', email: 'dave@example.com' }
});
const post = await tx.post.create({
data: { title: 'Dave\'s Post', authorId: user.id }
});
return [user, post];
});
Prisma aims to handle the common cases automatically while still giving you a way to define custom transaction flows when needed.
Relationship handling
Relationships between tables make databases powerful. The way you work with these connections shows another major difference between these tools.
Knex makes you manage relationships yourself using SQL concepts like joins:
// Getting a user with their posts
const rows = await knex('users')
.where('users.id', userId)
.join('posts', 'users.id', 'posts.author_id')
.select('users.*', 'posts.id as post_id', 'posts.title');
// You need to restructure the flat results yourself
const user = {
id: rows[0].id,
name: rows[0].name,
posts: rows.map(row => ({
id: row.post_id,
title: row.title
}))
};
// Or make separate queries
const user = await knex('users').where('id', userId).first();
const posts = await knex('posts').where('author_id', userId);
user.posts = posts;
This approach gives you control but means writing code to organize your data into a proper structure. You're responsible for handling all relationship logic.
Prisma treats relationships as a core feature in both schema and queries:
// Define relationships in the schema
model User {
id Int @id @default(autoincrement())
name String
posts Post[] // One-to-many relationship
}
model Post {
id Int @id @default(autoincrement())
title String
author User @relation(fields: [authorId], references: [id])
authorId Int
}
// Query with related data
const user = await prisma.user.findUnique({
where: { id: userId },
include: { posts: true }
});
// Result already has user.posts as an array
// Filter by relationship
const usersWithPosts = await prisma.user.findMany({
where: {
posts: { some: { published: true } }
},
include: {
posts: { where: { published: true } }
}
});
// Create related records together
const newUser = await prisma.user.create({
data: {
name: 'Alice',
posts: {
create: [{ title: 'First Post' }]
}
},
include: { posts: true }
});
Prisma handles the complex work of managing relationships automatically. This makes your code cleaner but means following Prisma's way of defining and querying relationships.
Migration support
Database schemas evolve as your application grows. Both tools offer ways to manage these changes, but with fundamentally different approaches.
Knex comes with a traditional migration system using JavaScript files:
// Create a migration
npx knex migrate:make add_user_settings
// In the generated file:
exports.up = function(knex) {
return knex.schema.table('users', table => {
table.jsonb('settings').defaultTo('{}');
table.boolean('email_notifications').defaultTo(true);
});
};
exports.down = function(knex) {
return knex.schema.table('users', table => {
table.dropColumn('settings');
table.dropColumn('email_notifications');
});
};
// Apply migrations
npx knex migrate:latest
With Knex, you write exactly what should change in each migration. You define both how to apply and reverse each change, giving you precise control over the process. Your migrations directly use Knex's schema builder, letting you leverage JavaScript for complex migration logic.
Prisma Migrate generates migrations automatically from changes to your schema:
// Just modify your schema.prisma file
model User {
id Int @id @default(autoincrement())
name String
settings Json? @default("{}")
emailNotifications Boolean @default(true) @map("email_notifications")
posts Post[]
}
// Then generate and apply a migration
npx prisma migrate dev --name add_user_settings
This creates an SQL migration file:
-- prisma/migrations/20230415123456_add_user_settings/migration.sql
ALTER TABLE "users" ADD COLUMN "settings" JSONB NOT NULL DEFAULT '{}';
ALTER TABLE "users" ADD COLUMN "email_notifications" BOOLEAN NOT NULL DEFAULT true;
Prisma's approach treats your schema file as the source of truth. You focus on defining what your database should look like, and Prisma figures out how to get there. This makes migrations simpler but less flexible - Prisma generates the SQL for you based on schema changes.
The key difference: Knex migrations are imperative (you specify how to change things), while Prisma migrations are declarative (you specify the end result). Prisma simplifies the common cases but gives you less control over the exact SQL.
Raw SQL support
Even with great tools, sometimes you need direct SQL access for performance or database-specific features. Both tools let you write raw SQL, but with different approaches.
Knex makes raw SQL feel natural and integrated:
// Simple raw query
const users = await knex.raw('SELECT * FROM users WHERE email = ?',
['alice@example.com']);
// Mix raw expressions with the query builder
const activeUsers = await knex('users')
.select(knex.raw('COUNT(*) as user_count'))
.whereRaw('last_login > now() - interval ?', ['7 days']);
// Named parameters
const posts = await knex.raw(`
SELECT p.*, u.name as author_name
FROM posts p JOIN users u ON p.author_id = u.id
WHERE p.published = :published LIMIT :limit
`, { published: true, limit: 10 });
Raw SQL in Knex feels like an extension of its main API. You can mix raw expressions with builder methods or write complete custom queries with safe parameter binding.
Prisma also supports raw SQL, but with a more structured approach:
// Tagged template queries
const users = await prisma.$queryRaw`
SELECT * FROM users WHERE email = ${email}
`;
// For data modification
const updated = await prisma.$executeRaw`
UPDATE users SET last_login = NOW()
WHERE id = ${userId}
`;
// Dynamic queries need a different method
const orderBy = 'created_at DESC';
const users = await prisma.$queryRawUnsafe(
`SELECT * FROM users ORDER BY ${orderBy} LIMIT $1`,
10
);
Prisma separates $queryRaw
for fetching data and $executeRaw
for changing data. Both use tagged templates for safe parameter handling. For dynamic queries, you need the special $queryRawUnsafe
method.
Knex's approach to raw SQL feels more seamless with its query builder nature. Prisma's support is comprehensive but feels more like a separate feature from its main API.
Type safety and validation
TypeScript has become essential for JavaScript development. The tools differ dramatically in how they support type safety.
Knex was created before TypeScript became popular, so it doesn't automatically create types for your database schema. You need to define and maintain them yourself:
// Manual type definitions with Knex
interface User {
id: number;
name: string;
email: string;
}
// Using your types with queries
const getUser = async (id: number): Promise<User | undefined> => {
return knex<User>('users').where({ id }).first();
};
const createUser = async (user: Omit<User, 'id'>): Promise<User> => {
const [newUser] = await knex<User>('posts')
.insert(user)
.returning('*');
return newUser;
};
This works, but you must keep your TypeScript interfaces in sync with your database schema manually. When your schema changes, you need to update your types too.
Prisma was built with TypeScript from the start. It automatically generates types from your schema:
// Prisma generates these types for you
import { User, Prisma } from '@prisma/client';
// Fully typed operations
const getUser = async (id: number): Promise<User | null> => {
return prisma.user.findUnique({
where: { id }
});
};
// Input types are also generated
const createUser = async (data: Prisma.UserCreateInput): Promise<User> => {
return prisma.user.create({ data });
};
// Complex queries stay type-safe
const result = await prisma.user.findUnique({
where: { id },
include: { posts: true }
});
// TypeScript knows result has a posts array
Prisma's type system goes beyond basic model types to include input types, filters, and nested query structures. When your schema changes, just run prisma generate
to update all your TypeScript definitions automatically.
This type safety difference is one of Prisma's biggest advantages. While you can add types to Knex, Prisma builds them in as a core feature that requires almost no extra work.
Testing support
Effective testing of database interactions is crucial for application reliability. Knex and Prisma offer different approaches to testing database code.
Knex provides a flexible foundation for testing but requires more manual setup:
// Setting up a test database with Knex
const knex = require('knex')({
client: 'sqlite3',
connection: {
filename: ':memory:' // In-memory SQLite for tests
},
useNullAsDefault: true
});
// Jest example
beforeAll(async () => {
// Run migrations on test database
await knex.migrate.latest();
});
afterAll(async () => {
await knex.destroy();
});
beforeEach(async () => {
// Clear data between tests
await knex('posts').truncate();
await knex('users').truncate();
});
test('creates a user', async () => {
const [userId] = await knex('users').insert({
name: 'Test User',
email: 'test@example.com'
}).returning('id');
const user = await knex('users').where({ id: userId }).first();
expect(user).toMatchObject({
name: 'Test User',
email: 'test@example.com'
});
});
For more complex applications, you might create a test helper that manages transactions to isolate tests:
const testWithTransaction = async (callback) => {
const trx = await knex.transaction();
try {
await callback(trx);
} finally {
await trx.rollback();
}
};
test('creates related records', async () => {
await testWithTransaction(async (trx) => {
const [userId] = await trx('users')
.insert({ name: 'Test User', email: 'test@example.com' })
.returning('id');
await trx('posts').insert({
title: 'Test Post',
author_id: userId
});
const posts = await trx('posts')
.join('users', 'posts.author_id', 'users.id')
.where('users.email', 'test@example.com')
.select('posts.*');
expect(posts).toHaveLength(1);
expect(posts[0].title).toBe('Test Post');
});
});
Prisma offers more integrated testing tools with its Jest preset and utilities for managing test environments:
// Setting up Prisma for testing
// jest.setup.js
import { PrismaClient } from '@prisma/client';
import { mockDeep, mockReset } from 'jest-mock-extended';
jest.mock('@prisma/client', () => ({
PrismaClient: jest.fn()
}));
beforeEach(() => {
mockReset(prismaMock);
});
export const prismaMock = mockDeep<PrismaClient>();
(PrismaClient as jest.Mock).mockImplementation(() => prismaMock);
// In your test file
import { prismaMock } from '../jest.setup';
import { UserService } from './user-service';
test('creates a user', async () => {
const user = {
id: 1,
name: 'Test User',
email: 'test@example.com'
};
prismaMock.user.create.mockResolvedValue(user);
const userService = new UserService(prismaMock);
const result = await userService.createUser({ name: 'Test User', email: 'test@example.com' });
expect(result).toEqual(user);
});
For integration tests with a real database, Prisma provides tools for creating isolated test environments:
// Integration testing with a real database
import { PrismaClient } from '@prisma/client';
import { execSync } from 'child_process';
import { join } from 'path';
import { v4 as uuid } from 'uuid';
const generateDatabaseURL = (schema: string) => {
// Generate a unique schema name for test isolation
return `postgresql://user:password@localhost:5432/testdb?schema=${schema}`;
};
jest.setTimeout(60000);
describe('User integration tests', () => {
let prisma: PrismaClient;
let schema: string;
beforeAll(async () => {
schema = `test_${uuid()}`;
process.env.DATABASE_URL = generateDatabaseURL(schema);
// Create the test schema and run migrations
execSync(`npx prisma migrate deploy`, {
env: {
...process.env,
DATABASE_URL: process.env.DATABASE_URL,
},
});
prisma = new PrismaClient();
});
afterAll(async () => {
await prisma.$executeRaw`DROP SCHEMA IF EXISTS "${schema}" CASCADE`;
await prisma.$disconnect();
});
test('creates and retrieves a user', async () => {
const user = await prisma.user.create({
data: {
name: 'Integration Test User',
email: 'integration@example.com'
}
});
const retrieved = await prisma.user.findUnique({
where: { id: user.id }
});
expect(retrieved).toMatchObject({
name: 'Integration Test User',
email: 'integration@example.com'
});
});
});
Prisma's testing approach benefits from its type system and structured API, making mocking and assertions more straightforward. However, both tools require thoughtful test design to ensure database tests are reliable and isolated.
Final thoughts
Choosing between Knex and Prisma depends on your project needs.
Pick Knex if you want direct SQL control, flexible query building, and easier integration into existing projects. It’s ideal for performance tuning and complex queries.
Choose Prisma if you value type safety, faster development, and a smoother developer experience. Its schema-first design and automatic types make it perfect for modern TypeScript apps.
Both are great tools: Knex offers control and flexibility, while Prisma focuses on productivity and reliability.
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us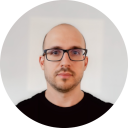
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github