As Kubernetes has become the de facto standard for container orchestration, developers need efficient ways to work with it locally. Local Kubernetes environments allow developers to build, test, and debug applications in a setup similar to production without requiring expensive cloud resources or complex infrastructure.
Two popular tools for running Kubernetes locally are Minikube and Kind (Kubernetes IN Docker). Both tools create local Kubernetes clusters, but they take different approaches to implementation, resource usage, and feature sets.
This article aims to help beginners understand both technologies, their differences, use cases, and how to choose the right one for specific development needs. We'll explore their architectures, benefits, limitations, and provide practical guidance on when to use each tool.
Understanding Minikube
Minikube is an open-source tool that enables running a single-node Kubernetes cluster locally. It was one of the first officially supported ways to run Kubernetes locally and has been part of the Kubernetes ecosystem since 2016.
Minikube's primary purpose is to provide developers with a production-like Kubernetes environment for local development and testing. It creates a virtual machine (or uses a container, depending on the driver) on your local computer, then installs and configures Kubernetes inside that environment.
Minikube solves several key problems for developers:
- Providing an isolated Kubernetes environment without cloud dependencies
- Enabling testing of Kubernetes-specific features locally
- Allowing application testing in a realistic environment before deployment
- Supporting learning and experimentation with Kubernetes
Minikube architecture
Minikube's architecture consists of a few key components:
Drivers: Minikube supports multiple virtualization and container technologies through drivers. These include:
- VirtualBox (cross-platform)
- HyperKit (macOS)
- Hyper-V (Windows)
- KVM (Linux)
- Docker (cross-platform)
- Podman (Linux)
The driver determines how Minikube creates the environment where Kubernetes runs.
Kubernetes Components: Inside the VM or container, Minikube sets up all standard Kubernetes components:
- API Server
- Controller Manager
- Scheduler
- etcd
- kubelet
- Container runtime (usually Docker or containerd)
Add-ons: Minikube includes a system for enabling optional components like:
- Dashboard (web UI)
- Metrics Server
- Ingress controller
- Storage provisioners
- Registry
Here's an example of starting Minikube with specific options:
minikube start --driver=virtualbox --memory=4096 --cpus=2 --kubernetes-version=v1.24.0
This command starts Minikube using VirtualBox as the driver, allocates 4GB of RAM and 2 CPUs, and specifies Kubernetes version 1.24.0.
- Profiles: Minikube supports multiple profiles, allowing you to run several different Kubernetes clusters simultaneously, each with its own configuration:
minikube start -p production-like --memory=8192 --cpus=4
minikube profile dev-cluster # Switch between profiles
Minikube offers several advantages for Kubernetes developers:
Production-like environment: Minikube provides a full-featured Kubernetes implementation that closely resembles production environments. This reduces the "it works on my machine" problem when moving applications to actual clusters.
Rich add-ons ecosystem: Minikube includes numerous add-ons that make it easy to enable common Kubernetes components:
# View available add-ons
minikube addons list
# Enable an add-on
minikube addons enable ingress
# Disable an add-on
minikube addons disable dashboard
Stable and well-documented: As one of the oldest and most widely used local Kubernetes solutions, Minikube has extensive documentation, community support, and proven stability.
Dashboard and observability tools: Minikube makes it easy to access visual tools for monitoring and managing your cluster:
Despite its strengths, Minikube has some limitations:
Resource requirements: Minikube tends to require more resources than alternatives, especially when using VM-based drivers. The default configuration allocates 2GB of RAM, but real-world usage often requires more.
Performance considerations: The virtualization layer in VM-based setups can impact performance, particularly for disk I/O operations.
Multi-node limitations: While Minikube technically supports multi-node clusters as of version 1.10.1, this feature is still experimental and not as robust as purpose-built multi-node solutions like Kind.
Understanding Kind (Kubernetes IN Docker)
Kind (Kubernetes IN Docker) is an open-source tool for running local Kubernetes clusters using Docker containers as "nodes." Originally created to test Kubernetes itself, Kind has evolved into a popular development tool due to its lightweight architecture and multi-node capabilities.
Kind's approach is different from Minikube's: instead of creating VMs, it uses Docker containers to simulate Kubernetes nodes. This makes Kind particularly efficient in terms of resource usage and startup time.
Kind's architecture is centered around its innovative Docker-in-Docker approach:
Node containers: Kind creates special Docker containers that function as Kubernetes "nodes." These containers run systemd and all the necessary Kubernetes components.
Cluster networking: Kind sets up an internal Docker network for communication between node containers.
Multi-node support: Kind excels at creating multi-node clusters, allowing you to test more complex Kubernetes features like node affinity, pod distribution, and high availability configurations.
Creating a cluster with Kind is straightforward:
kind create cluster --name my-cluster
To create a multi-node cluster, you can use a configuration file:
kind: Cluster
apiVersion: kind.x-k8s.io/v1alpha4
nodes:
- role: control-plane
- role: worker
- role: worker
- role: worker
kind create cluster --config kind-config.yaml --name multi-node
Benefits of Kind
Kind offers several advantages, particularly for specific use cases:
Lightweight resource requirements: Kind typically uses fewer resources than Minikube, making it suitable for development on less powerful machines.
Fast startup time: Creating a Kind cluster is usually faster than a Minikube cluster, especially when using VM-based drivers in Minikube.
Multi-node cluster support: Kind's ability to create multi-node clusters easily makes it excellent for testing features that require multiple nodes:
apiVersion: apps/v1
kind: Deployment
metadata:
name: node-distribution-test
spec:
replicas: 9
selector:
matchLabels:
app: distribution-test
template:
metadata:
labels:
app: distribution-test
spec:
affinity:
podAntiAffinity:
preferredDuringSchedulingIgnoredDuringExecution:
- weight: 100
podAffinityTerm:
labelSelector:
matchExpressions:
- key: app
operator: In
values:
- distribution-test
topologyKey: "kubernetes.io/hostname"
containers:
- name: pause
image: k8s.gcr.io/pause:3.5
This deployment demonstrates pod anti-affinity, which requires multiple nodes to test properly.
- CI/CD integration: Kind was built for testing Kubernetes itself, making it ideal for integration into continuous integration pipelines:
name: Test with Kind
on: [push, pull_request]
jobs:
test:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- uses: engineerd/setup-kind@v0.5.0
with:
version: v0.11.1
- name: Test
run: |
kubectl cluster-info
kubectl apply -f ./kubernetes/
./test-scripts/run-e2e-tests.sh
Challenges with Kind
While Kind offers many benefits, it has its own set of challenges:
Limited add-ons compared to Minikube: Kind doesn't have the built-in add-on system that Minikube provides, so you'll need to manually install components like an ingress controller or metrics server.
Networking differences: Since Kind runs within Docker, there are some networking differences compared to VM-based solutions, particularly around port forwarding and service exposure:
kind: Cluster
apiVersion: kind.x-k8s.io/v1alpha4
nodes:
- role: control-plane
extraPortMappings:
- containerPort: 30000
hostPort: 8080
protocol: TCP
Storage persistence complexities: Persistent volumes in Kind require additional configuration, and data doesn't persist by default when you delete and recreate clusters.
Comparing Minikube and Kind
Prerequisites
- Minikube requires a hypervisor (like VirtualBox) when using VM-based drivers, or Docker for the Docker driver.
- Kind requires only Docker to be installed.
Installation process
For Minikube:
# macOS with Homebrew
brew install minikube
# Linux
curl -LO https://storage.googleapis.com/minikube/releases/latest/minikube-linux-amd64
sudo install minikube-linux-amd64 /usr/local/bin/minikube
# Windows with Chocolatey
choco install minikube
For Kind:
# macOS with Homebrew
brew install kind
# Linux
curl -Lo ./kind https://kind.sigs.k8s.io/dl/v0.12.0/kind-linux-amd64
chmod +x ./kind
sudo mv ./kind /usr/local/bin/kind
# Windows with Chocolatey
choco install kind
Initial configuration
- Minikube offers more configuration options at startup, including memory, CPU, Kubernetes version, and add-ons
- Kind has a simpler initial setup but requires config files for advanced configurations
Resource utilization
Memory and CPU usage:
- Minikube typically requires more resources, with defaults around 2GB RAM
- Kind is generally more lightweight, especially for single-node clusters
Disk space requirements:
- Minikube requires more disk space, especially for VM-based drivers
- Kind's container-based approach uses less disk space overall
Startup and shutdown performance
In most environments, Kind will start up faster than Minikube, especially when using VM-based drivers for Minikube.
You can time them with:
# Time Minikube startup
time minikube start
# Time Kind startup
time kind create cluster
Feature comparison
Add-ons and plugins:
- Minikube has a built-in add-ons system with many pre-packaged components
- Kind requires manual installation of most components
# List Minikube add-ons
minikube addons list
# With Kind, you need to install components manually
kubectl apply -f https://raw.githubusercontent.com/kubernetes/ingress-nginx/main/deploy/static/provider/kind/deploy.yaml
Ingress support:
- Minikube has built-in ingress support via its add-on.
- Kind requires manual setup of ingress controllers with special configuration.
Storage options:
- Minikube provides built-in storage provisioners
- Kind requires more configuration for persistent storage
kind: Cluster
apiVersion: kind.x-k8s.io/v1alpha4
nodes:
- role: control-plane
extraMounts:
- hostPath: /tmp/data
containerPath: /data
Multi-cluster support:
- Minikube supports multiple clusters through profiles
- Kind supports multiple clusters by name
# Create multiple Minikube clusters
minikube start -p cluster1
minikube start -p cluster2
# Create multiple Kind clusters
kind create cluster --name cluster1
kind create cluster --name cluster2
When to use Minikube
Minikube is particularly well-suited for:
Production-like development: When you need a local environment that closely resembles your production Kubernetes setup, Minikube provides a more complete experience.
Need for add-ons and dashboards: If you want easy access to tools like the Kubernetes dashboard, metrics server, or ingress controllers without manual configuration.
Stable environment for demos: Minikube's maturity and stability make it reliable for demonstrations and workshops.
Learning Kubernetes fundamentals: For beginners learning Kubernetes, Minikube's comprehensive feature set and documentation make it a good learning platform.
apiVersion: apps/v1
kind: Deployment
metadata:
name: hello-minikube
spec:
selector:
matchLabels:
app: hello-minikube
replicas: 2
template:
metadata:
labels:
app: hello-minikube
spec:
containers:
- name: hello-minikube
image: k8s.gcr.io/echoserver:1.4
ports:
- containerPort: 8080
---
apiVersion: v1
kind: Service
metadata:
name: hello-minikube
spec:
type: NodePort
ports:
- port: 8080
nodePort: 30000
selector:
app: hello-minikube
When to use Kind
Kind shines in these scenarios:
- CI/CD pipelines and testing: Kind's lightweight nature and quick startup make it ideal for automated testing environments.
#!/bin/bash
# Create a Kind cluster for testing
kind create cluster
# Deploy the application
kubectl apply -f kubernetes/
# Run tests
go test ./e2e/...
# Clean up
kind delete cluster
Low-resource environments: On systems with limited RAM or CPU, Kind's container-based approach uses fewer resources.
Multi-node cluster testing: When you need to test features that depend on having multiple Kubernetes nodes.
kind: Cluster
apiVersion: kind.x-k8s.io/v1alpha4
nodes:
- role: control-plane
- role: control-plane
- role: control-plane
- role: worker
- role: worker
- role: worker
This configuration creates a highly available cluster with three control plane nodes and three workers.
- Quick ephemeral clusters: When you need to create and delete clusters frequently, Kind's speed is advantageous.
Final thoughts
Minikube and Kind represent different approaches to local Kubernetes development, each with its own strengths and ideal use cases. Minikube provides a more comprehensive, production-like environment with numerous built-in features, while Kind offers a lightweight, fast solution particularly well-suited for multi-node testing and CI/CD pipelines.
For beginners just starting with Kubernetes, Minikube's rich feature set and extensive documentation make it an excellent learning platform. Its built-in dashboard and add-ons system provide a smoother introduction to Kubernetes concepts without requiring manual setup of components.
For developers with resource constraints or those who need to frequently create and destroy clusters, Kind's efficiency and speed make it the better choice. Its multi-node capabilities also make it valuable for testing distributed applications or Kubernetes features that rely on node distribution.
As container orchestration continues to evolve, both Minikube and Kind remain essential tools in the Kubernetes developer's toolkit, providing accessible ways to work with Kubernetes locally and build container-based applications with confidence.
Thanks for reading!
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us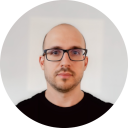
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github