Docker vs Kubernetes: A Comprehensive Comparison
Containerization has revolutionized how we develop, deploy, and scale applications. It provides a way to package software with all its dependencies into standardized units that can run consistently across different environments.
At the forefront of this revolution are Docker and Kubernetes, two technologies that have become fundamental to modern application development and deployment.
Docker emerged first, offering a simple way to create, package, and run containers. It made containerization accessible to developers by providing tools that were relatively easy to use. Kubernetes came later, addressing the challenges of managing multiple containers at scale, particularly in production environments.
This article aims to help you understand both technologies, their differences, how they complement each other, and when to use each one. We'll explore their architectures, benefits, limitations, and how they work together to power modern applications.
Understanding Docker
Docker is an open-source platform that automates the deployment of applications inside lightweight, portable containers. A container is a standalone executable package that includes everything needed to run an application: code, runtime, system tools, libraries, and settings.
Unlike virtual machines which virtualize an entire operating system, Docker containers share the host system's OS kernel but run in isolated user spaces. This makes containers much lighter and faster to start than virtual machines.
Docker's architecture consists of several core components that work together to create, manage, and run containers. Let's examine each of these components:
Docker Engine
The Docker Engine is the core technology that powers Docker. It's a client-server application with:
- A server component with a long-running daemon process (
dockerd
) that manages Docker objects. - REST API that programs can use to communicate with the daemon.
- Background processes that handle container execution, networking, and storage.
The Engine is responsible for building and running containers, managing container images, and providing the foundation for all Docker functionality.
Docker CLI
The Docker Command Line Interface (CLI) is the primary way users interact with Docker. The CLI uses the Docker REST API to control or interact with the Docker daemon through scripting or direct CLI commands.
Common Docker CLI commands include:
# Build an image from a Dockerfile
docker build -t myapp .
# Run a container from an image
docker run -d -p 8080:80 myapp
# List running containers
docker ps
# Stop a running container
docker stop container_id
The CLI makes Docker accessible to you without requiring an understanding of the underlying API details, allowing for straightforward container management.
Docker images
A Docker image is a read-only template with instructions for creating a Docker container. It contains the application code, libraries, dependencies, tools, and other files needed for an application to run.
Images are built from instructions written in a Dockerfile
. Here's an example
of a simple Dockerfile
for a Node.js application:
FROM node:22
WORKDIR /app
COPY package*.json ./
RUN npm install
COPY . .
EXPOSE 3000
CMD ["node", "app.js"]
This Dockerfile
does the following:
- Starts with a base Node.js 22 image.
- Sets the working directory inside the container to
/app
. - Copies package files and installs dependencies.
- Copies the rest of the application code.
- Exposes port 3000.
- Specifies the command to run when the container starts.
To build an image from this Dockerfile
, you would run:
docker build -t my-node-app .
Docker containers
A container is a runnable instance of an image. You can create, start, stop, move, or delete containers using the Docker API or CLI.
To run a container from the image we created:
docker run -p 3000:3000 my-node-app
This command runs a container from the my-node-app
image and maps port 3000
from the container to port 3000 on the host machine, making the application
accessible through http://localhost:3000
.
Docker Compose
Docker Compose is a tool for defining and running multi-container Docker applications. With a YAML file, you configure your application's services, networks, and volumes, then create and start all services with a single command.
Here's an example compose.yaml
file for a web application with a Node.js
backend and MongoDB database:
services:
web:
build: .
ports:
- "3000:3000"
depends_on:
- mongo
environment:
- MONGO_URI=mongodb://mongo:27017/myapp
mongo:
image: mongo
ports:
- "27017:27017"
volumes:
- mongo-data:/data/db
volumes:
mongo-data:
To start this application:
docker compose up
This command builds the web service image if needed, pulls the MongoDB image, creates the necessary networks and volumes, and starts both services.
Benefits of Docker
Docker offers several advantages for developers and operations teams:
Consistency across environments: Docker ensures that applications run the same way regardless of where they're deployed. This eliminates the "it works on my machine" problem by packaging all dependencies within the container.
Lightweight resource usage: Containers share the host OS kernel, making them much more efficient than virtual machines. A server can run many more containers than VMs with the same hardware.
Quick startup time: Containers can start in seconds, compared to minutes for virtual machines. This rapid startup makes containers ideal for dynamic scaling and microservices architectures.
Isolation of applications: Each container runs in its own isolated environment, preventing conflicts between applications with different requirements.
Limitations of Docker
While Docker is powerful, it has some limitations:
Limited orchestration capabilities: Docker alone is great for running containers on a single host but lacks robust tools for managing containers across multiple servers.
Challenges with scaling: As your application grows to dozens or hundreds of containers, managing them manually becomes increasingly difficult without additional tools.
Single server focus: Docker's native tools are primarily designed for managing containers on a single server, not for coordinating containers across a cluster of machines.
These limitations led to the development of container orchestration platforms like Kubernetes.
Understanding Kubernetes
Kubernetes, often abbreviated as K8s, is an open-source container orchestration platform originally developed by Google. It automates the deployment, scaling, and management of containerized applications across clusters of hosts.
Kubernetes provides a framework to run distributed systems resiliently. It takes care of scaling and failover for your application, provides deployment patterns, and helps manage the lifecycle of containerized applications.
Its architecture consists of two main components: the control plane and nodes.
The control plane manages the cluster and includes several components:
- API Server: The front end of the Kubernetes control plane, exposing the Kubernetes API.
- etcd: A consistent and highly-available key-value store used as Kubernetes' backing store for all cluster data.
- Scheduler: Watches for newly created pods with no assigned node, and selects a node for them to run on.
- Controller Manager: Runs controller processes such as node controller and replication controller.
- Cloud Controller Manager: Links your cluster with your cloud provider's API.
Nodes are the worker machines that run containerized applications and include:
- Kubelet: An agent that ensures containers are running in a pod.
- Kube-proxy: A network proxy that maintains network rules on nodes.
- Container Runtime: The software responsible for running containers (e.g., Docker).
Kubernetes uses several basic objects to represent the state of your cluster:
- Pods: The smallest deployable units in Kubernetes, representing a group of one or more containers with shared storage and network resources.
apiVersion: v1
kind: Pod
metadata:
name: nginx-pod
spec:
containers:
- name: nginx
image: nginx:latest
ports:
- containerPort: 80
- Services: An abstract way to expose an application running on a set of Pods as a network service.
apiVersion: v1
kind: Service
metadata:
name: nginx-service
spec:
selector:
app: nginx
ports:
- port: 80
targetPort: 80
type: ClusterIP
- Deployments: Provide declarative updates for Pods and ReplicaSets, allowing for easy scaling and rolling updates.
apiVersion: apps/v1
kind: Deployment
metadata:
name: nginx-deployment
spec:
replicas: 3
selector:
matchLabels:
app: nginx
template:
metadata:
labels:
app: nginx
spec:
containers:
- name: nginx
image: nginx:latest
ports:
- containerPort: 80
To apply these configurations to your Kubernetes cluster:
kubectl apply -f deployment.yaml
Benefits of Kubernetes
Kubernetes provides several advantages for managing containerized applications:
- Scalability: Kubernetes makes it easy to scale your application horizontally by adding or removing instances of your containers based on demand. You can manually scale your application or set up autoscaling based on metrics like CPU usage.
kubectl scale deployment nginx-deployment --replicas=5
This command scales the nginx-deployment
to 5 replicas.
High availability: Kubernetes is designed to ensure your applications stay running even when individual nodes fail. It automatically reschedules pods from failed nodes to healthy ones, maintaining the desired state of your application.
Rolling updates and rollbacks: Kubernetes allows you to update your application with zero downtime through rolling updates, replacing old pods with new ones gradually. If something goes wrong, you can easily roll back to the previous version.
kubectl rollout undo deployment/nginx-deployment
This command rolls back a deployment to its previous version.
Self-healing capabilities: Kubernetes continuously monitors the health of your application and infrastructure. If a container fails, Kubernetes automatically restarts it. If a node dies, Kubernetes reschedules all the pods running on it to other available nodes.
Load balancing: Kubernetes automatically distributes network traffic to ensure stability and prevent any single instance from becoming overwhelmed. Services in Kubernetes provide built-in load balancing for pod instances.
Challenges with Kubernetes
While Kubernetes offers powerful capabilities, it comes with its own challenges:
Steep learning curve: Kubernetes has a complex architecture with many concepts and components to understand. This makes it difficult for beginners to grasp and use effectively.
Complex setup and maintenance: Setting up and maintaining a Kubernetes cluster requires significant expertise and resources. Even with managed Kubernetes services from cloud providers, understanding how to configure and troubleshoot the system requires specialized knowledge.
Resource requirements: Kubernetes has substantial overhead and is generally not suitable for small applications or limited resources. The control plane components themselves require computing resources that might be overkill for simple applications.
How Docker and Kubernetes work together
Docker and Kubernetes have a complementary relationship where Docker provides the container technology, and Kubernetes provides the orchestration platform for those containers.
Docker is focused on creating, distributing, and running containers on individual machines. It provides the tooling to build container images, push them to registries, and run them as containers.
Kubernetes, on the other hand, focuses on orchestrating large numbers of containers across multiple machines. It handles scheduling, scaling, service discovery, load balancing, rolling updates, and more.
Container runtime interface
Kubernetes uses a Container Runtime Interface (CRI) to interact with container runtimes. For many years, Docker was the default container runtime for Kubernetes, but this has changed with the deprecation of dockershim in Kubernetes 1.24.
Today, Kubernetes can work with any container runtime that implements the CRI, including:
- containerd: A lightweight container runtime that's actually used under the hood by Docker itself
- CRI-O: A container runtime specifically designed for Kubernetes
- Docker (via the cri-dockerd adapter): Still usable but requires an external adapter
When using Docker with Kubernetes, the workflow generally looks like this:
- Developers build container images using Docker.
- Images are pushed to a container registry.
- Kubernetes pulls these images and schedules them as containers within pods.
A practical workflow
Let's look at a practical workflow for deploying a containerized application to Kubernetes:
- Build an image with Docker:
docker build -t myapp:1.0 .
- Tag and push the image to a registry:
docker tag myapp:1.0 myregistry.com/myapp:1.0
docker push myregistry.com/myapp:1.0
- Define a Kubernetes deployment that uses the image:
apiVersion: apps/v1
kind: Deployment
metadata:
name: myapp
spec:
replicas: 3
selector:
matchLabels:
app: myapp
template:
metadata:
labels:
app: myapp
spec:
containers:
- name: myapp
image: myregistry.com/myapp:1.0
ports:
- containerPort: 8080
- Apply the deployment to your Kubernetes cluster:
kubectl apply -f deployment.yaml
- Create a service to expose your application:
apiVersion: v1
kind: Service
metadata:
name: myapp-service
spec:
selector:
app: myapp
ports:
- port: 80
targetPort: 8080
type: LoadBalancer
- Apply the service*:
kubectl apply -f service.yaml
This workflow demonstrates how Docker and Kubernetes work together: Docker handles the containerization of your application, while Kubernetes handles the deployment, scaling, and management of those containers in a cluster environment.
When to use Docker and Kubernetes
Docker by itself is well-suited for several scenarios:
Local development environments: Docker provides a consistent development environment across team members. Using Docker Compose, developers can run multi-container applications locally with a single command.
Simple applications with few containers: If your application consists of only a few containers and doesn't need advanced orchestration features, Docker with Docker Compose might be sufficient.
CI/CD pipelines: Docker containers are perfect for continuous integration and continuous delivery pipelines. They provide isolated, reproducible environments for building and testing applications.
Testing environments: Docker makes it easy to spin up isolated testing environments and tear them down afterward, ensuring tests run in a clean state each time.
On the other hand, Kubernetes shines in more complex scenarios such as:
Production environments with multiple services: When you have many microservices that need to communicate with each other, Kubernetes provides service discovery, load balancing, and networking capabilities to manage these interactions.
Applications requiring high availability: Kubernetes' self-healing capabilities ensure your application remains available even when individual containers or nodes fail.
Microservices architectures: Kubernetes provides the tools needed to manage complex microservices architectures, including service discovery, ingress controllers, and secret management.
Dynamic scaling: When your application needs to scale dynamically based on demand, Kubernetes' horizontal pod autoscaler can automatically adjust the number of running instances.
When choosing between the two, a practical approach could be:
- Start with Docker for local development and simple deployments.
- Use Docker Compose for multi-container applications.
- Consider managed Kubernetes services (like EKS, GKE, or AKS) when you need advanced orchestration.
- Gradually migrate production workloads to Kubernetes as your team's expertise grows.
Final thoughts
The decision between Docker alone or with Kubernetes isn't binary—many organizations use both in different contexts. Docker remains excellent for development environments and simple deployments, while Kubernetes excels at managing production workloads at scale. Understanding the strengths and limitations of each technology allows you to make informed decisions about your container strategy.
The container ecosystem continues to evolve rapidly, with new tools and approaches emerging regularly. By building a solid foundation in Docker and Kubernetes, you'll be well-prepared to adapt to these changes and leverage containers effectively in your software development lifecycle.
Thanks for reading!
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us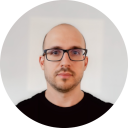
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github