Docker Compose vs Kubernetes: Choosing the Right Container Orchestration Tool
Containerization has revolutionized how we develop, deploy, and manage applications. The ability to package an application with all its dependencies into a standardized unit has made software deployment more consistent and reliable across different environments. However, as organizations adopt containers, they quickly discover that managing individual containers becomes challenging at scale. This is where container orchestration tools like Docker Compose and Kubernetes come into play.
Both Docker Compose and Kubernetes help manage containerized applications, but they serve different needs and come with different levels of complexity. This article explores these two popular container orchestration solutions, comparing their features, use cases, and helping you decide which tool is right for your specific requirements.
Docker Compose: The simple orchestrator
Docker Compose is a tool for defining and running multi-container Docker applications. It uses a YAML file to configure application services, networks, and volumes, allowing you to launch and manage multiple containers with a single command.
Docker Compose was designed to simplify the process of running applications that require multiple containers to work together. It's particularly helpful during development, testing, and for smaller production deployments that don't need advanced scaling or high availability features.
Key features of Docker Compose
Docker Compose offers several features that make it attractive for developers and small teams:
- Simplicity: With a straightforward YAML configuration, it's easy to define complex applications.
- Service definition: Each container is defined as a service with its own image, ports, volumes, and environment variables.
- Network management: Compose automatically creates networks for your application.
- Volume persistence: Easily define and manage persistent data volumes.
- Environment variable management: Configure applications for different environments using environment variables.
- Local development excellence: Perfect for development workflows on local machines.
The compose.yaml file
At the heart of Docker Compose is the compose.yaml
file, which defines your
application stack. Here's an example of a basic compose.yaml
file for a web
application with a database:
services:
web:
image: nginx:latest
ports:
- "8080:80"
volumes:
- ./website:/usr/share/nginx/html
depends_on:
- app
networks:
- frontend
- backend
app:
build: ./app
environment:
- DATABASE_URL=postgres://postgres:password@db:5432/mydb
depends_on:
- db
networks:
- backend
db:
image: postgres:15
environment:
- POSTGRES_USER=postgres
- POSTGRES_PASSWORD=password
- POSTGRES_DB=mydb
volumes:
- postgres_data:/var/lib/postgresql/data
networks:
- backend
networks:
frontend:
backend:
volumes:
postgres_data:
This file defines three services: a web server (nginx), an application server, and a database (PostgreSQL). It also sets up networks to isolate communication and configures a volume for the database to persist data.
Starting and managing applications with Docker Compose
To start the application defined in your compose.yaml file, simply run:
docker-compose up
You'll see the logs from all containers in your terminal. To run the containers
in the background, add the -d
flag:
docker-compose up -d
To check the status of your services:
docker-compose ps
And to stop all services:
docker-compose down
Docker Compose makes it easy to manage the lifecycle of your application's containers. You can also scale specific services up or down:
docker-compose up -d --scale app=3
This command would start three instances of the app service.
Limitations of Docker Compose
Despite its simplicity and usefulness, Docker Compose has several limitations:
- It's primarily designed for development and testing, not for complex production environments
- Limited load balancing capabilities
- No built-in auto-scaling features
- Basic health checks but no sophisticated self-healing
- No native support for rolling updates
- Runs on a single host by default (though it can be extended with Docker Swarm)
Kubernetes: The enterprise-grade orchestrator
Kubernetes, often abbreviated as K8s, is an open-source platform designed to automate deploying, scaling, and operating application containers. Originally developed by Google and now maintained by the Cloud Native Computing Foundation, Kubernetes provides a framework to run distributed systems resiliently.
Kubernetes is built for production environments at scale. It can manage thousands of containers across multiple hosts, handling networking, storage, and computing resources intelligently.
Key components of Kubernetes
Kubernetes has a more complex architecture than Docker Compose, consisting of several key components:
- Nodes: The physical or virtual machines that run your applications.
- Pods: The smallest deployable units in Kubernetes, containing one or more containers.
- Services: Abstractions that define a logical set of pods and policies to access them.
- Deployments: Declarative descriptions of the desired state for pods and replica sets.
- ConfigMaps and Secrets: Resources for handling configuration and sensitive data.
- Namespaces: Virtual clusters within a physical cluster for resource isolation.
- Persistent Volumes: Storage resources that persist beyond the lifetime of pods.
Kubernetes architecture
A Kubernetes cluster consists of at least one control plane (master) and multiple worker nodes. The control plane manages the cluster, while worker nodes run the actual application workloads.
Defining applications in Kubernetes
In Kubernetes, applications are defined using YAML manifests. Here's an example of a simple web application deployment:
apiVersion: apps/v1
kind: Deployment
metadata:
name: web-app
labels:
app: web
spec:
replicas: 3
selector:
matchLabels:
app: web
template:
metadata:
labels:
app: web
spec:
containers:
- name: nginx
image: nginx:latest
ports:
- containerPort: 80
resources:
limits:
memory: "128Mi"
cpu: "500m"
This deployment will create three replicas of an nginx container. To expose this deployment as a service:
apiVersion: v1
kind: Service
metadata:
name: web-service
spec:
selector:
app: web
ports:
- port: 80
targetPort: 80
type: LoadBalancer
Deploying and managing applications with Kubernetes
To deploy an application to Kubernetes, you typically use the kubectl
command-line tool:
kubectl apply -f deployment.yaml
kubectl apply -f service.yaml
To check the status of your deployment:
kubectl get deployments
NAME READY UP-TO-DATE AVAILABLE AGE
web-app 3/3 3 3 45s
And to view your services:
kubectl get services
NAME TYPE CLUSTER-IP EXTERNAL-IP PORT(S) AGE
kubernetes ClusterIP 10.96.0.1 <none> 443/TCP 1h
web-service LoadBalancer 10.96.45.123 203.0.113.10 80:30123/TCP 30s
Kubernetes provides powerful tools for monitoring and managing your applications:
kubectl logs -l app=web
kubectl exec -it pod/web-app-54d9b6775f-abcd1 -- /bin/bash
kubectl describe deployment web-app
Advanced Kubernetes features
Kubernetes offers numerous advanced features that set it apart from simpler orchestration tools:
Auto-scaling
Kubernetes can automatically scale your applications based on resource usage:
apiVersion: autoscaling/v2
kind: HorizontalPodAutoscaler
metadata:
name: web-app-hpa
spec:
scaleTargetRef:
apiVersion: apps/v1
kind: Deployment
name: web-app
minReplicas: 3
maxReplicas: 10
metrics:
- type: Resource
resource:
name: cpu
target:
type: Utilization
averageUtilization: 70
Apply this configuration to automatically scale your deployment based on CPU usage:
kubectl apply -f hpa.yaml
Self-healing
Kubernetes continuously monitors the health of your applications and automatically replaces failed containers:
apiVersion: apps/v1
kind: Deployment
metadata:
name: web-app
spec:
replicas: 3
selector:
matchLabels:
app: web
template:
metadata:
labels:
app: web
spec:
containers:
- name: nginx
image: nginx:latest
livenessProbe:
httpGet:
path: /
port: 80
initialDelaySeconds: 30
periodSeconds: 10
Rolling updates
Kubernetes supports zero-downtime deployments with rolling updates:
apiVersion: apps/v1
kind: Deployment
metadata:
name: web-app
spec:
replicas: 5
strategy:
type: RollingUpdate
rollingUpdate:
maxSurge: 1
maxUnavailable: 1
selector:
matchLabels:
app: web
template:
metadata:
labels:
app: web
spec:
containers:
- name: nginx
image: nginx:1.21
To update the application:
kubectl set image deployment/web-app nginx=nginx:1.22 --record
Comparison: Complexity and learning curve
One of the most significant differences between Docker Compose and Kubernetes is the learning curve.
Docker Compose: Simple and straightforward
Docker Compose is designed for simplicity. If you already understand Docker containers, you can pick up Docker Compose in a day or two. The configuration is intuitive, and the commands are easy to learn and use.
A simple multi-container application might look like this:
version: '3'
services:
web:
image: nginx:latest
ports:
- "80:80"
redis:
image: redis:latest
Kubernetes: Steep learning curve
Kubernetes, on the other hand, has a much steeper learning curve. It introduces many new concepts and abstractions that take time to understand fully.
The same simple application in Kubernetes would require multiple YAML files:
apiVersion: apps/v1
kind: Deployment
metadata:
name: web
spec:
replicas: 1
selector:
matchLabels:
app: web
template:
metadata:
labels:
app: web
spec:
containers:
- name: nginx
image: nginx:latest
ports:
- containerPort: 80
apiVersion: v1
kind: Service
metadata:
name: web
spec:
selector:
app: web
ports:
- port: 80
targetPort: 80
type: ClusterIP
apiVersion: apps/v1
kind: Deployment
metadata:
name: redis
spec:
replicas: 1
selector:
matchLabels:
app: redis
template:
metadata:
labels:
app: redis
spec:
containers:
- name: redis
image: redis:latest
Comparison: Scalability
Scalability is another area where these tools differ significantly.
Docker Compose: Limited scalability
Docker Compose offers basic scaling capabilities. You can scale services within a single host using:
docker-compose up -d --scale web=3
However, this approach has limitations:
- All containers run on a single host
- No cross-host networking (without Docker Swarm)
- Manual load balancing required
- No intelligent scheduling
Kubernetes: Built for scale
Kubernetes excels at scalability:
- Runs across multiple hosts
- Automatically distributes workloads
- Intelligent scheduling based on resource requirements
- Built-in load balancing
- Horizontal scaling with HorizontalPodAutoscaler
- Vertical scaling with VerticalPodAutoscaler
- Cluster autoscaling capabilities
Scaling an application in Kubernetes is straightforward:
kubectl scale deployment web-app --replicas=10
Or using an autoscaler, as shown earlier.
Comparison: High availability and fault tolerance
High availability is critical for production applications, and this is another area where the two tools differ.
Docker Compose: Basic fault tolerance
Docker Compose offers restart policies, which is a form of basic fault tolerance:
version: '3'
services:
web:
image: nginx:latest
restart: always
However, it lacks advanced high availability features:
- No automatic rescheduling across hosts
- No self-healing capabilities beyond container restarts
- Limited monitoring and health checking
Kubernetes: Advanced high availability
Kubernetes is designed with high availability in mind:
- Self-healing: automatically restarts failed containers
- Replica sets ensure the desired number of pods are running
- Pod disruption budgets prevent too many pods from going down simultaneously
- Node affinity and anti-affinity rules distribute workloads
- Advanced health checks with liveness and readiness probes
Example of a readiness probe:
apiVersion: apps/v1
kind: Deployment
metadata:
name: web-app
spec:
replicas: 3
selector:
matchLabels:
app: web
template:
metadata:
labels:
app: web
spec:
containers:
- name: nginx
image: nginx:latest
readinessProbe:
httpGet:
path: /healthz
port: 80
initialDelaySeconds: 5
periodSeconds: 5
Comparison: Deployment and management
Both tools offer different approaches to deployment and management.
Docker Compose workflow
Docker Compose offers a simple workflow:
- Define your application in compose.yaml
- Start your application with
docker-compose up
- Update services by editing the YAML file and rerunning
docker-compose up
- View logs with
docker-compose logs
- Stop and remove containers with
docker-compose down
Example of environment-specific configurations:
version: '3'
services:
web:
environment:
- DEBUG=true
docker-compose -f compose.yaml -f docker-compose.override.yml up
Kubernetes deployment process
Kubernetes deployment is more complex:
- Define resources in multiple YAML files
- Apply manifests using
kubectl apply
- Wait for resources to be provisioned
- Monitor rollout status
- Debug issues with
kubectl describe
andkubectl logs
Kubernetes introduces the concept of declarative configuration management. You describe the desired state, and Kubernetes works to maintain that state.
Example of managing different environments:
apiVersion: kustomize.config.k8s.io/v1beta1
kind: Kustomization
resources:
- deployment.yaml
- service.yaml
patchesStrategicMerge:
- dev-patch.yaml
apiVersion: apps/v1
kind: Deployment
metadata:
name: web-app
spec:
template:
spec:
containers:
- name: nginx
env:
- name: DEBUG
value: "true"
kubectl apply -k ./
Decision factors: When to choose which
The decision between Docker Compose and Kubernetes depends on several factors.
Consider Docker Compose when:
- You're developing locally or in a small team
- Your application has a simple architecture with a few containers
- You need a quick setup for testing or development
- You don't require advanced scaling or high availability
- Your team is new to containerization
- You're running in a single-server environment
- You need a lightweight solution with minimal overhead
Consider Kubernetes when:
- You're deploying to production at scale
- Your application has a complex architecture with many services
- You need robust high availability and failover capabilities
- Auto-scaling is a requirement
- You're running across multiple servers or cloud environments
- You need sophisticated deployment strategies (blue/green, canary)
- You require fine-grained resource control and isolation
- Your organization has dedicated DevOps resources
Project size considerations
For small projects (1-5 containers), Docker Compose often provides the best balance of features versus complexity.
For medium projects (5-20 containers), Docker Compose may still work, but you'll start feeling its limitations, especially in production environments.
For large projects (20+ containers), Kubernetes is typically the better choice, despite its added complexity.
Team expertise and resources
Consider your team's expertise:
- Docker Compose requires basic Docker knowledge
- Kubernetes requires understanding of containers, networking, distributed systems, and specific Kubernetes concepts
In terms of resources, running Kubernetes requires:
- More CPU and memory overhead
- Dedicated operations knowledge
- Monitoring and management tools
Migration path
Many organizations start with Docker Compose for development and then migrate to Kubernetes for production. This staged approach allows teams to gain experience with containerization before tackling the complexity of Kubernetes.
A typical evolution might look like:
- Single containers with Docker
- Multi-container applications with Docker Compose
- Simple Kubernetes deployments for production
- Advanced Kubernetes features as needs grow
Integration possibilities
Docker Compose and Kubernetes don't have to be mutually exclusive choices.
Using Docker Compose with Kubernetes
You can use Docker Compose for local development while using Kubernetes in production. Tools like Kompose help with the transition:
kompose convert -f compose.yaml
This converts your compose.yaml file into Kubernetes manifests.
Another approach is to use Docker Desktop, which includes Kubernetes support. This allows you to run both Docker Compose and Kubernetes locally.
Hybrid approaches
Some teams use:
- Docker Compose for development
- Kubernetes for staging and production
- CI/CD pipelines to bridge the environments
Example CI/CD workflow:
- Developers use Docker Compose locally
- CI system builds images and runs tests using Docker Compose
- CI system converts configs to Kubernetes manifests
- CD system deploys to Kubernetes clusters
Final thoughts
Docker Compose and Kubernetes serve different needs in the container orchestration ecosystem. Docker Compose excels in simplicity and rapid development, making it ideal for local development, testing, and small-scale deployments. Kubernetes, while more complex, provides the robust features needed for enterprise-grade, scalable applications in production.
The choice between these tools should be based on your specific requirements, team expertise, and the scale of your operations. Many organizations find value in using both: Docker Compose for development simplicity and Kubernetes for production reliability.
As containerization continues to evolve, both tools are likely to improve and perhaps even converge in some areas. However, their fundamental differences in design philosophy—simplicity versus comprehensive features—will likely keep them as complementary rather than competing solutions.
Remember that the best tool is the one that solves your specific problems with the least amount of overhead. Start with the simplest solution that meets your needs, and be ready to evolve as those needs grow and change.
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us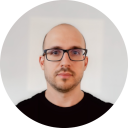
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github