Mastering Exponential Backoff in Distributed Systems
Imagine you're at a crowded coffee shop. You approach the counter to place an order, but the barista is overwhelmed and asks you to come back in a minute.
A reasonable approach would be to wait a minute, then try again. If they're still busy, you might wait two minutes before your next attempt, then four minutes, and so on. This natural human behavior is the essence of exponential backoff.
In computing, exponential backoff is a retry strategy where each failed attempt triggers a delay that increases exponentially before the next retry. It's a fundamental technique used to improve system resilience, respect rate limits, and handle transient failures gracefully.
When services communicate over networks, failures are inevitable. Servers go down for maintenance, networks experience congestion, and APIs enforce rate limits.
By implementing exponential backoff, your application becomes more courteous to external services and more resilient against temporary disruptions.
This article explores exponential backoff from first principles, providing practical implementations, and explaining when and how to apply this powerful technique in your applications.
Understanding the core concepts
Before diving into implementation details, let's understand the three core concepts that make exponential backoff work: retry mechanisms, backoff strategies, and jitter.
1. Retry mechanism
A retry mechanism is a simple concept: when an operation fails, try it again. However, effective retry mechanisms require careful consideration of several factors:
Identifying retryable failures: Not all failures should trigger retries. For example, a 404 (Not Found) error likely won't resolve with retries, but a 503 status code often will.
Timeout management: Each retry attempt should have a reasonable timeout to prevent hanging indefinitely.
Retry budgeting: Systems need a maximum number of retry attempts to avoid infinite loops.
Here's a simple JavaScript function that implements a basic retry mechanism:
async function retryOperation(operation, maxRetries) {
let retries = 0;
while (retries <= maxRetries) {
try {
return await operation();
} catch (error) {
if (retries >= maxRetries) {
throw error; // Rethrow if we've exhausted retries
}
console.log(`Operation failed, retrying (${retries + 1}/${maxRetries})...`);
retries++;
// We'll add a delay strategy here later
}
}
}
While this function provides the structure for retries, it's missing a crucial element: the delay between retries. That's where backoff strategies come in.
2. Backoff strategy: Timing is everything
A backoff strategy determines how long to wait between retry attempts. There are several common approaches:
Constant backoff: Wait the same amount of time between each retry. This is a simple but ineffective for many scenarios.
Linear backoff: Increase the wait time linearly (e.g., wait 1 second, then 2 seconds, then 3 seconds).
Exponential backoff: Increase the wait time exponentially (e.g., wait 1 second, then 2 seconds, then 4 seconds, then 8 seconds).
Exponential backoff is particularly effective because it starts with quick retries for potentially brief disruptions, then rapidly backs off to give overwhelmed systems time to recover.
The mathematical formula for exponential backoff is:
delay = initialDelay * (factor ^ retryNumber)
Where factor
is typically 2, resulting in a doubling of delay with each retry.
Here's how you can implement exponential backoff in JavaScript:
function calculateExponentialBackoff(retry, initialDelay, factor) {
return initialDelay * Math.pow(factor, retry);
}
async function retryWithExponentialBackoff(operation, maxRetries, initialDelay = 1000, factor = 2) {
let retries = 0;
while (retries <= maxRetries) {
try {
return await operation();
} catch (error) {
if (retries >= maxRetries) {
throw error; // Rethrow if we've exhausted retries
}
const delay = calculateExponentialBackoff(retries, initialDelay, factor);
console.log(`Operation failed, retrying in ${delay}ms...`);
await new Promise(resolve => setTimeout(resolve, delay));
retries++;
}
}
}
This implementation introduces increasingly longer delays between retries. Starting with an initial delay of 1 second, it will wait 2 seconds before the second retry, 4 seconds before the third, and so on.
3. Jitter: Avoiding the thundering herd
While exponential backoff is effective, it has a potential flaw: if multiple clients experience failures simultaneously, they might all retry at the same time, creating synchronized waves of traffic. This thundering herd problem can overwhelm services just as they're trying to recover.
Jitter solves this by adding randomness to the delay. Instead of all clients retrying after exactly 2 seconds, some might wait 1.7 seconds, others 2.3 seconds, spreading out the load.
There are several jitter strategies:
- Full jitter: Completely random delay up to the calculated exponential backoff value:
actualDelay = random(0, exponentialDelay)
- Equal jitter: Half fixed delay, half random:
actualDelay = (exponentialDelay / 2) + random(0, exponentialDelay / 2)
- Decorrelated jitter: Builds on the previous delay with some randomness:
actualDelay = min(maxDelay, random(baseDelay, 3 * baseDelay))
Full jitter typically performs best in practice. Here's an implementation:
function calculateBackoffWithJitter(retry, initialDelay, factor, cap) {
// Calculate basic exponential backoff
const exponentialDelay = initialDelay * Math.pow(factor, retry);
// Apply cap if provided
const cappedDelay = cap ? Math.min(exponentialDelay, cap) : exponentialDelay;
// Apply full jitter: random delay between 0 and the calculated delay
return Math.random() * cappedDelay;
}
async function retryWithBackoffAndJitter(
operation,
maxRetries,
initialDelay = 1000,
factor = 2,
maxDelay = 30000
) {
let retries = 0;
while (retries <= maxRetries) {
try {
return await operation();
} catch (error) {
if (retries >= maxRetries) {
throw error; // Rethrow if we've exhausted retries
}
const delay = calculateBackoffWithJitter(retries, initialDelay, factor, maxDelay);
console.log(`Operation failed, retrying in ${Math.round(delay)}ms...`);
await new Promise(resolve => setTimeout(resolve, delay));
retries++;
}
}
}
Notice we've also introduced a maxDelay
parameter. This caps the maximum wait
time to prevent extremely long delays after multiple retries, which is important
for user-facing applications where responsiveness matters.
Implementing exponential backoff
Now that we understand the core concepts, let's build a more complete implementation. A robust exponential backoff should:
- Distinguish between retryable and non-retryable errors.
- Include configurable parameters.
- Provide logging for debugging.
- Respect maximum retry limits.
Here's a more comprehensive implementation:
class ExponentialBackoff {
constructor({
initialDelay = 1000,
maxDelay = 30000,
factor = 2,
jitter = true,
maxRetries = 5,
retryableErrors = [
'ECONNRESET', 'ETIMEDOUT', 'ECONNREFUSED', 'EPIPE',
429, 503, 504 // Status codes
]
} = {}) {
this.initialDelay = initialDelay;
this.maxDelay = maxDelay;
this.factor = factor;
this.jitter = jitter;
this.maxRetries = maxRetries;
this.retryableErrors = retryableErrors;
}
isRetryable(error) {
if (error.status && this.retryableErrors.includes(error.status)) {
return true;
}
if (error.code && this.retryableErrors.includes(error.code)) {
return true;
}
return false;
}
calculateDelay(retry) {
// Calculate basic exponential delay
const exponentialDelay = this.initialDelay * Math.pow(this.factor, retry);
// Apply cap
const cappedDelay = Math.min(exponentialDelay, this.maxDelay);
// Apply jitter if enabled
if (this.jitter) {
return Math.random() * cappedDelay;
}
return cappedDelay;
}
async execute(operation) {
let retries = 0;
while (true) {
try {
return await operation();
} catch (error) {
if (
retries >= this.maxRetries ||
!this.isRetryable(error)
) {
throw error;
}
const delay = this.calculateDelay(retries);
console.log(
`Operation failed with error ${error.message || error}. ` +
`Retrying in ${Math.round(delay)}ms (${retries + 1}/${this.maxRetries})...`
);
await new Promise(resolve => setTimeout(resolve, delay));
retries++;
}
}
}
}
This implementation encapsulates exponential backoff logic in a reusable class with configurable parameters. Here's how you might use it:
// Create the backoff instance with custom parameters
const backoff = new ExponentialBackoff({
initialDelay: 500, // Start with 500ms delay
maxDelay: 60000, // Maximum delay of 1 minute
factor: 3, // Triple the delay each time
maxRetries: 8, // Try up to 8 times
jitter: true // Apply randomization
});
// Define the operation that might fail
async function fetchData() {
const response = await fetch('https://api.example.com/data');
if (!response.ok) {
const error = new Error('Request failed');
error.status = response.status;
throw error;
}
return response.json();
}
// Execute with backoff
backoff.execute(fetchData)
.then(data => console.log('Data retrieved successfully:', data))
.catch(error => console.error('All retries failed:', error));
When to use exponential backoff (and when not to)
Exponential backoff is a powerful strategy for managing retries, but like any technique, it's not appropriate for every situation. Understanding when to apply it and when to use alternative approaches is key to building truly resilient systems.
Ideal scenarios for exponential backoff
Exponential backoff shines in scenarios involving transient failure. When services experience momentary outages for whatever reason, your application needs a way to "ride out" these disruptions.
Similarly, network congestion issues where packet loss, delayed transmissions, and connection resets cause temporary failures tend to clear up naturally as traffic patterns shift.
In both cases, exponential backoff prevents your application from bombarding recovering systems with a flood of retry attempts that could delay their recovery.
Rate limiting presents another perfect use case. When you receive a 429 response, it is an explicit signal that you need to back off and try again later.
Distributed synchronization scenarios also benefit greatly from this approach. When multiple clients compete for resources like distributed locks or database rows, exponential backoff with jitter helps spread out retry attempts, reducing contention and increasing the likelihood of eventual success without constant collisions.
The randomness introduced by jitter is particularly valuable here, as it prevents clients from falling into lockstep patterns of repeated conflicts.
The common thread among these situations is their ephemeral nature, where patience and a measured approach to retries can turn failure into eventual success.
When exponential backoff falls short
Despite its utility, exponential backoff isn't a universal solution. There are several categories of failures where continued retries (regardless of the backoff strategy) is unlikely to help and may even make things worse:
Client-side errors (4xx status codes) generally indicate problems that won't resolve through retrying as these responses represent fundamental issues with the request itself, not temporary service conditions.
The exception is 429, which specifically indicates a temporary rate limiting situation where backing off is precisely the right approach.
Permanent server errors can be trickier to identify, as they often use the same 5xx status codes as temporary failures. This is why limiting the number of retry attempts is necessary as it prevents your system from endlessly pursuing what might be an unresolvable issue.
More sophisticated implementations might also analyze error patterns across multiple requests or timeframes, using contextual clues from error messages or system behavior to distinguish between momentary hiccups and systemic failures.
In these cases, a more appropriate response involves error handling, fallback strategies, or human intervention rather than automated retries with increasingly longer delays.
Beyond simple retries: Advanced resilience patterns
Implementing exponential backoff is just the beginning of building truly resilient systems. The most robust applications combine multiple complementary strategies to handle different failure modes intelligently.
Let's explore how these advanced patterns work together to create systems that can weather a wide range of disruptions.
The circuit breaker pattern
Circuit breakers complement exponential backoff by temporarily suspending all requests when a service appears to be experiencing prolonged issues.
Just as an electrical circuit breaker prevents damage by cutting power during overloads, this pattern prevents your application from wasting resources on requests that are unlikely to succeed.
Here's how a basic circuit breaker works alongside exponential backoff:
class CircuitBreaker {
constructor(options = {}) {
this.failureThreshold = options.failureThreshold || 5;
this.resetTimeout = options.resetTimeout || 30000;
this.state = 'CLOSED'; // CLOSED = allow requests, OPEN = block requests
this.failureCount = 0;
this.lastFailureTime = null;
}
// Additional methods omitted for brevity
async execute(operation) {
if (this.isOpen()) {
throw new Error('Circuit breaker is open, request rejected');
}
try {
const result = await operation();
this.recordSuccess();
return result;
} catch (error) {
this.recordFailure();
throw error;
}
}
}
The circuit breaker transitions between three states:
- Closed: Requests flow normally, but failures are counted.
- Open: Requests are immediately rejected without attempting execution.
- Half-Open: After a timeout period, a test request is allowed through to check if the service has recovered.
Unlike exponential backoff, which still attempts each request (with delay), the circuit breaker prevents requests entirely when the failure threshold is reached.
This gives overwhelmed services complete relief from your application's requests while they recover.
Bulkhead pattern
Named after the compartments that prevent ships from sinking when one section is breached, the bulkhead pattern isolates components so that failures in one area don't cascade through your entire system. In practice, this means:
- Using separate connection pools for different services.
- Implementing distinct thread pools or request quotas for critical vs. non-critical operations.
- Maintaining independent circuit breakers for different endpoints or services.
- Isolating retry mechanisms by service domain.
This isolation ensures that if one service becomes problematic, its issues won't consume all your application's resources through excessive retries or hanging connections.
Telemetry and adaptive strategies
The most sophisticated resilience systems adapt their behavior based on observed patterns. By collecting telemetry about failure rates, response times, and retry outcomes, your application can dynamically adjust its resilience parameters.
For example, if a particular endpoint consistently requires 3-4 retries before succeeding, your backoff strategy might start with a higher initial delay for that specific service.
Similarly, if failures cluster around certain times of day, your circuit breaker might become more sensitive during those periods.
This adaptive approach requires:
- Comprehensive logging of retry attempts, failures, and successes.
- Metrics collection to identify patterns across requests.
- Dynamic configuration to adjust parameters based on observed behavior.
- Service health checks to proactively test availability before critical operations.
Best practices for implementation
When implementing these resilience patterns:
Cap maximum delays to ensure your application remains responsive. Even with exponential backoff, setting an upper limit (typically 30-60 seconds) prevents extremely long waits after multiple retries.
Distinguish between user-facing and background operations. User-facing operations might prioritize responsiveness with more aggressive timeout values, while background jobs can afford more patience with longer delays and more retry attempts.
Log all retry attempts with sufficient context for debugging and pattern analysis. Include information about the operation, attempt number, delay used, and specific error encountered.
Implement global retry budgets to prevent retry storms across your application. For example, you might limit your system to no more than 1000 retries per minute across all operations.
Consider retry fairness when serving multiple users or operations. Ensure that retries from one user or component don't starve resources needed for other operations.
By thoughtfully combining these resilience patterns and following these best practices, your applications can gracefully handle an impressive range of failure scenarios, from momentary network hiccups to prolonged service outages, all while remaining responsive and conserving resources.
Final thoughts
The most resilient systems don't just react to failures. They anticipate them, classify them appropriately, and respond with measured strategies tailored to each scenario.
Exponential backoff, when thoughtfully implemented and intelligently combined with other patterns, forms the cornerstone of this resilience strategy, ensuring your applications remain available and responsive even in challenging conditions.
Thanks for reading!
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us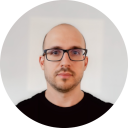
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github