Questions
Find answers to frequently asked development questions. For information about Better Stack products, explore our docs.
How can I access Docker environment variables from a cron job?
To access Docker environment variables from a cron job, you can use the -e option when running the docker run command to pass the environment variables to the container, and then use them in your c...
Run a CRON job on the last day of the month?
To run a cron job on the last day of the month, you can use the following format in your crontab file: 0 0 28-31 * * [ $(date -d "+1 day" +%d) = "01" ] && /path/to/command This cron job will run at...
How to run a Cron job weekly?
To run a cron job weekly, you can use the following format in your crontab file: 0 0 * * 0 /path/to/command This cron job will run at midnight (0 hour and 0 minute) on Sunday (day 0). Replace /path...
How to run a cron job inside a Docker Container?
To run a cron job inside a Docker container, you can follow these steps: Step 1 - Create a Dockerfile First, you need to create a Dockerfile that defines the image for your container. In the Docker...
How to log cron jobs?
To log cron jobs, you can use the following steps: Redirect the output You can redirect the output of the cron job to a log file by adding the following line at the end of the cron job command: >> ...
How to view the contents of docker images?
To view the contents of a Docker image, you can use the docker run command to start a container from the image and then use commands such as ls or cat to view the contents of the container. If you ...
What is the (best) way to manage permissions for Docker shared volumes?
When sharing volumes between a Docker container and the host or between multiple containers, it is important to manage permissions carefully to ensure that the correct users and groups have the nec...
What is the difference between links and depends_on in docker_compose.yml?
Both links and depends_on are used in a Docker Compose file (docker-compose.yml) to define relationships between containers. However, they differ in the way they establish these relationships. Link...
How to Debug a Failed Docker Build Command
When a Docker build command fails, there are several steps you can take to debug the issue
How to detach from a container without stopping it?
To detach from a container without stopping it, you can use the CTRL + P followed by CTRL + Q key sequence while attached to the container using the docker attach command or by running the containe...
What's the difference between Docker Compose and Kubernetes?
Docker Compose and Kubernetes are both container orchestration tools, but they differ in several ways: Scope and complexity Docker Compose is a simpler tool designed to manage a single Docker host ...
How to preserve data when the docker container exits?
When a Docker container exits, any data that was not persisted to a persistent storage will be lost. To preserve data when a Docker container exits, you can use one or more of the following methods...
What is the difference between Running and Starting a Docker container?
In Docker, the difference between running and starting a container is as follows: Running a container means that the container is already started and is currently executing its main process. This c...
How to use sudo inside a docker container?
By default, the root user inside a Docker container does not require sudo to perform privileged operations. This is because Docker containers run as a non-root user by default, and this user has fu...
How to locate the Docker daemon log?
The location of the Docker daemon log file can vary depending on your operating system and the configuration of your Docker installation. Here are the common locations: Linux (systemd): /var/log/sy...
How to use SSH keys inside docker container ?
To use SSH keys inside a Docker container, you can follow these steps: If you haven't already done so, you need to generate an SSH key pair. You can do this using the ssh-keygen command on your loc...
How to clear the logs properly for a Docker container?
To clear the logs for a Docker container, you can use the logrotate command, which is included in most Linux distributions. Here are the steps: First, you need to access the logs for the container ...
How to fix permission denied error when connecting to Docker?
If you are encountering a "permission denied" error when trying to connect to Docker, it is likely that you are not running the Docker commands with sufficient privileges. Here are a few steps you ...
How to connect to PostgreSQL running in a docker container from outside?
To connect to PostgreSQL running in a Docker container from outside the container, you need to expose the PostgreSQL port and provide the necessary authentication credentials. Here are the steps: W...
How to expose a port on a live Docker container?
To expose a port on a live Docker container, you can use the docker container port command. Here are the steps: To expose a port on a live Docker container, you need to first find the container ID ...
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us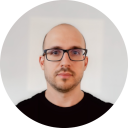
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github
Thank you to everyone who
Here is to all the fantastic people that are contributing and sharing their amazing projects: Thank you!