Understanding Python super() with init() methods
The super()
function is used to call a method from a parent class. When used with the __init__
method, it allows you to initialize the attributes of the parent class, in addition to any attributes defined in the child class.
For example, if you have a parent class Person
with an __init__
method that sets the name attribute, and a child class Student
that inherits from Person
and has its own __init__
method that sets the student_id
attribute, you can use super()
to call the __init__
method of the parent class and set the name attribute while also setting the student_id attribute in the child class:
class Person:
def __init__(self, name):
self.name = name
class Student(Person):
def __init__(self, name, student_id):
super().__init__(name)
self.student_id = student_id
When you create a new Student
object, the __init__
method of the parent class Person
is called first to set the name attribute, and then the __init__
method of the child class Student
is called to set the student_id attribute.
It is important to note that the super()
call must be made after any attribute assignments in the child class, so that the attributes from the parent class are initialized before the child class modifies them.
-
How to Copy Files in Python?
To copy a file in Python, you can use the shutil module. Here is an example of how you can use the shutil.copy() function to copy a file: import shutil shutil.copy('/path/to/source/file', '/path/to...
Questions -
How to flatten a list in Python?
You can flatten a list in python using the following one-liner: flat_list = [item for sublist in l for item in sublist] In the example above, l is the list of lists that is to be flattened. The pre...
Questions -
How do I get the current time in Python?
There are two ways you can get the current time in python. using the datetime object using the time module Using the datetime object First, you need to import the datetime module. Then by calling t...
Questions -
What are metaclasses in Python?
In Python, a metaclass is the class of a class. It defines how a class behaves, including how it is created and how it manages its instances. A metaclass is defined by inheriting from the built-in ...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us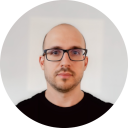
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github