Random string generation with upper case letters and digits in Python?
You can use the random
module and the string
module to generate a random string of a specified length that includes upper case letters and digits. Here's an example function that generates a random string of length n
:
import random
import string
def generate_random_string(n):
return ''.join(random.choices(string.ascii_uppercase + string.digits, k=n))
print(generate_random_string(10))
This function first imports the random
and string
modules, then defines a function called generate_random_string
that takes an argument n
for the length of the string to be generated. The function uses the random.choices()
method to randomly select characters from the string.ascii_uppercase
(uppercase letters) and string.digits
(digits) strings, concatenates them, and join them to form a string of length n
.
-
How do I pad a string with zeroes in Python?
You can pad a string with zeros in Python by using the zfill() method. This method adds zeros to the left of the string until it reaches the specified length. Here's an example: s = '123' padded = ...
Questions -
How to check if the string is empty in Python?
To check if a string is empty in Python, you can use the len() function. For example: string = "" if len(string) == 0: print("The string is empty") You can also use the not operator to check if...
Questions -
How do I get a substring of a string in Python?
To get a substring of a string in Python, you can use the string slicing notation, which is string[start:end], where start is the index of the first character of the substring, and end is the index...
Questions -
How to convert string into datetime in Python?
To convert a string into a datetime object in Python, you can use the datetime.strptime() function. This function allows you to specify the format of the input string, and it will return a datetime...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us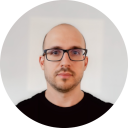
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github