How to check if the string is empty in Python?
To check if a string is empty in Python, you can use the len()
function. For example:
string = ""
if len(string) == 0:
print("The string is empty")
You can also use the not
operator to check if the string is not empty:
string = ""
if not string:
print("The string is empty")
Alternatively, you can use the bool()
function to check if the string is empty. An empty string is considered to be False
, and all other strings are considered to be True
:
string = ""
if not bool(string):
print("The string is empty")
Note that all of these approaches treat a string that consists only of whitespace characters (such as spaces, tabs, or newlines) as being non-empty. To check if a string is empty or consists only of whitespace characters, you can use the strip()
function to remove leading and trailing whitespace and then check if the resulting string is empty:
string = " "
if not string.strip():
print("The string is empty or consists only of whitespace characters")
-
Convert bytes to a string in Python and vice versa?
To convert a string to bytes in Python, you can use the bytes function. This function takes two arguments: the string to encode and the encoding to use. The default encoding is utf-8. Here is an ex...
Questions -
How do I get a substring of a string in Python?
To get a substring of a string in Python, you can use the string slicing notation, which is string[start:end], where start is the index of the first character of the substring, and end is the index...
Questions -
How do I pad a string with zeroes in Python?
You can pad a string with zeros in Python by using the zfill() method. This method adds zeros to the left of the string until it reaches the specified length. Here's an example: s = '123' padded = ...
Questions -
How to convert string into datetime in Python?
To convert a string into a datetime object in Python, you can use the datetime.strptime() function. This function allows you to specify the format of the input string, and it will return a datetime...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us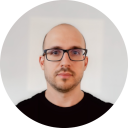
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github