How to check if a given key already exists in a dictionary in Python?
You can use the in
keyword to check if a key exists in a dictionary. For example:
my_dict = {'a': 1, 'b': 2, 'c': 3}
if 'a' in my_dict:
print("Key 'a' exists in the dictionary")
if 'd' in my_dict:
print("Key 'd' exists in the dictionary")
The first if
statement will print "Key 'a' exists in the dictionary", but the second if
statement will not execute because the key 'd' does not exist in the dictionary.
Alternatively, you can use the dict.get()
method to check if a key exists in a dictionary. This method returns the value for the given key if it exists in the dictionary, and returns a default value (which you can specify) if the key does not exist. For example:
my_dict = {'a': 1, 'b': 2, 'c': 3}
value = my_dict.get('a') # value is 1
value = my_dict.get('d') # value is None
value = my_dict.get('d', 'default') # value is 'default'
-
How do I check if a list is empty in Python?
You can check if a list is empty by using the len() function to check the length of the list. If the length of the list is 0, then it is empty. Here's an example: my_list = [] if len(my_list) == 0:...
Questions -
How do I pass a variable by reference in Python?
In Python, variables are passed to functions by reference. This means that when you pass a variable to a function, you are passing a reference to the memory location where the value of the variable...
Questions -
How to remove a key from a Python dictionary?
To remove a key from a dictionary, you can use the del statement. Here's an example: my_dict = {'a': 1, 'b': 2, 'c': 3} del my_dict['b'] print(my_dict) # Output: {'a': 1, 'c': 3} Alternatively, yo...
Questions -
What are metaclasses in Python?
In Python, a metaclass is the class of a class. It defines how a class behaves, including how it is created and how it manages its instances. A metaclass is defined by inheriting from the built-in ...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us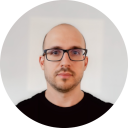
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github