How do I split Python list into equally-sized chunks?
To split a list into equally sized chunks, you can use the grouper
function from the itertools
module.
Here's an example of how you can use it:
from itertools import zip_longest
def grouper(iterable, n, fillvalue=None):
"Collect data into fixed-length chunks or blocks"
# grouper('ABCDEFG', 3, 'x') --> ABC DEF Gxx
args = [iter(iterable)] * n
return zip_longest(*args, fillvalue=fillvalue)
# Example usage
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
n = 3
chunks = list(grouper(my_list, n))
print(chunks)
This will output the following list of chunks:
[(1, 2, 3), (4, 5, 6), (7, 8, 9), (10, None, None)]
You can also use list slicing to achieve the same result:
def split_list(my_list, chunk_size):
return [my_list[i:i + chunk_size] for i in range(0, len(my_list), chunk_size)]
# Example usage
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
chunk_size = 3
chunks = split_list(my_list, chunk_size)
print(chunks)
This will output the following list of chunks:
[[1, 2, 3], [4, 5, 6], [7, 8, 9], [10]]
You can choose the method that best fits your needs.
-
How to Copy Files in Python?
To copy a file in Python, you can use the shutil module. Here is an example of how you can use the shutil.copy() function to copy a file: import shutil shutil.copy('/path/to/source/file', '/path/to...
Questions -
How to flatten a list in Python?
You can flatten a list in python using the following one-liner: flat_list = [item for sublist in l for item in sublist] In the example above, l is the list of lists that is to be flattened. The pre...
Questions -
Understanding Python super() with init() methods
The super() function is used to call a method from a parent class. When used with the __init__ method, it allows you to initialize the attributes of the parent class, in addition to any attributes ...
Questions -
Understanding slicing in Python?
Slicing is a way of extracting a specific part of an array. The syntax is following: mylist[start:end] # items start through end-1 mylist[start:] # items start through the rest of the array ...
Questions -
What are metaclasses in Python?
In Python, a metaclass is the class of a class. It defines how a class behaves, including how it is created and how it manages its instances. A metaclass is defined by inheriting from the built-in ...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us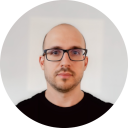
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github