How to find the current directory and file's parent directory in Python?
You can use the os
module in Python to find the current directory and the parent directory of a file.
To get the current directory, you can use os.getcwd()
.
To get the parent directory of a file, you can use os.path.dirname(file_path)
.
For example:
import os
# Get current directory
current_dir = os.getcwd()
print(current_dir)
# Get parent directory of a file
file_path = '/path/to/file.txt'
parent_dir = os.path.dirname(file_path)
print(parent_dir)
Note that the os.path.dirname()
function takes a file path as an argument and returns the parent directory of that file.
-
How do I access environment variables in Python?
You can access environment variables in Python using the os module. Here's an example: import os Access an environment variable value = os.environ['VARIABLE_NAME'] Set an environment variable os.en...
Questions -
How to Copy Files in Python?
To copy a file in Python, you can use the shutil module. Here is an example of how you can use the shutil.copy() function to copy a file: import shutil shutil.copy('/path/to/source/file', '/path/to...
Questions -
How do I pass a variable by reference in Python?
In Python, variables are passed to functions by reference. This means that when you pass a variable to a function, you are passing a reference to the memory location where the value of the variable...
Questions -
How do I make a time delay in Python?
There are a few ways to make a time delay in Python. Here are three options: time.sleep(): You can use the sleep() function from the time module to add a delay to your program. The sleep() function...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us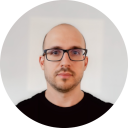
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github