How do I pass a variable by reference in Python?
In Python, variables are passed to functions by reference. This means that when you pass a variable to a function, you are passing a reference to the memory location where the value of the variable is stored, rather than the value itself.
This is different from some other programming languages, which pass variables by value, meaning that a copy of the value is passed to the function.
Here's an example of how you might pass a variable to a function and modify its value inside the function:
def my_function(x):
x = x * 2
print(x)
y = 5
my_function(y)
print(y)
This would output the following:
10
5
As you can see, the value of y
is not modified by the function. This is because the value of x
inside the function is a new reference to a different memory location, and it is not connected to the original y
variable.
If you want to modify the value of a variable that is passed to a function, you can use a mutable data type such as a list or dictionary. Here's an example using a list:
def my_function(x):
x[0] = x[0] * 2
print(x)
y = [5]
my_function(y)
print(y)
This would output the following:
[10]
[10]
In this case, the value of y
is modified by the function because the x
variable refers to the same memory location as the y
variable.
-
How to Copy Files in Python?
To copy a file in Python, you can use the shutil module. Here is an example of how you can use the shutil.copy() function to copy a file: import shutil shutil.copy('/path/to/source/file', '/path/to...
Questions -
How to flatten a list in Python?
You can flatten a list in python using the following one-liner: flat_list = [item for sublist in l for item in sublist] In the example above, l is the list of lists that is to be flattened. The pre...
Questions -
Understanding slicing in Python?
Slicing is a way of extracting a specific part of an array. The syntax is following: mylist[start:end] # items start through end-1 mylist[start:] # items start through the rest of the array ...
Questions -
What are metaclasses in Python?
In Python, a metaclass is the class of a class. It defines how a class behaves, including how it is created and how it manages its instances. A metaclass is defined by inheriting from the built-in ...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us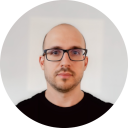
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github