How to remove a key from a Python dictionary?
To remove a key from a dictionary, you can use the del
statement. Here's an example:
my_dict = {'a': 1, 'b': 2, 'c': 3}
del my_dict['b']
print(my_dict) # Output: {'a': 1, 'c': 3}
Alternatively, you can use the pop()
method, which removes the key-value pair from the dictionary and returns the value. If the key is not found in the dictionary, the pop()
method will return a default value (e.g., None
). Here's an example:
my_dict = {'a': 1, 'b': 2, 'c': 3}
value = my_dict.pop('b', None)
print(value) # Output: 2
print(my_dict) # Output: {'a': 1, 'c': 3}
You can also use the pop()
method to remove a key-value pair from the dictionary and return the default value if the key is not found, like this:
my_dict = {'a': 1, 'b': 2, 'c': 3}
value = my_dict.pop('b', 'Key not found')
print(value) # Output: 2
print(my_dict) # Output: {'a': 1, 'c': 3}
-
Understanding Python super() with init() methods
The super() function is used to call a method from a parent class. When used with the __init__ method, it allows you to initialize the attributes of the parent class, in addition to any attributes ...
Questions -
How to manually raising (throwing) an exception in Python?
To manually raise an exception in Python, use the raise statement. Here is an example of how to use it: def calculatepayment(amount, paymenttype): if paymenttype != "Visa" and paymenttype != "M...
Questions -
How do I make a time delay in Python?
There are a few ways to make a time delay in Python. Here are three options: time.sleep(): You can use the sleep() function from the time module to add a delay to your program. The sleep() function...
Questions -
How do I pass a variable by reference in Python?
In Python, variables are passed to functions by reference. This means that when you pass a variable to a function, you are passing a reference to the memory location where the value of the variable...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us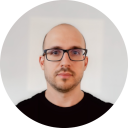
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github