How do I write JSON data to a file in Python?
You can use the json
module in Python to write JSON data to a file. The module has a dump()
function that can be used to write JSON data to a file-like object. Here's an example of how you can use it:
import json
data = {
"name": "John Smith",
"age": 35,
"city": "New York"
}
with open("data.json", "w") as file:
json.dump(data, file)
In this example, data
is a Python dictionary that contains the JSON data. The dump()
function takes two arguments: the JSON data and the file-like object to which the data should be written. The with open
statement opens the file "data.json" in "w" mode, which means it will be opened for writing. The file will be created if it does not exist, and truncated if it does exist. The json.dump()
writes the JSON data to the file and file.close()
is called automatically when the block inside the with statement is exited.
-
How do I append to a file in Python?
To append to a file in Python, you can use the "a" mode in the open() function. This will open the file in append mode, which means that you can write new data at the end of the file. Here is an ex...
Questions -
How to Copy Files in Python?
To copy a file in Python, you can use the shutil module. Here is an example of how you can use the shutil.copy() function to copy a file: import shutil shutil.copy('/path/to/source/file', '/path/to...
Questions -
How to read a file line-by-line in Python?
To read a file line-by-line in Python, you can use the following approach: with open('file.txt') as f: for line in f: print(line) This will open the file, read each line in the file, an...
Questions -
How To Write Logs To A File With Python?
If you are new to logging in Python, please feel free to start with our Introduction to Python logging to get started smoothly. Otherwise, here is how to write logs to a file in Python: Using Basic...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us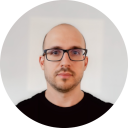
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github