How to Log Js Errors From a Client Into Kibana?
To log JavaScript errors from a client (e.g., a web application) into Kibana, you'll need to set up a process that captures these errors on the client side, sends them to a logging service, and then indexes them into Elasticsearch, which Kibana can then visualize.
Here’s a step-by-step approach to achieve this:
1. Capture JavaScript Errors
First, you need to capture JavaScript errors on the client side. This can be done using a combination of JavaScript error handling and a logging library.
Using window.onerror
and window.addEventListener
You can capture errors using window.onerror
and window.addEventListener
for unhandled promise rejections:
// Capture global errors
window.onerror = function(message, source, lineno, colno, error) {
logErrorToServer({
message: message,
source: source,
lineno: lineno,
colno: colno,
error: error ? error.stack : null
});
};
// Capture unhandled promise rejections
window.addEventListener('unhandledrejection', function(event) {
logErrorToServer({
message: event.reason.message,
source: null,
lineno: null,
colno: null,
error: event.reason.stack
});
});
// Function to send errors to your server
function logErrorToServer(errorData) {
fetch('<https://your-server-endpoint/logs>', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify(errorData)
});
}
2. Send Errors to a Logging Service
You need a server or service that can receive these errors and forward them to Elasticsearch. You can use Logstash, an HTTP endpoint, or a third-party service like Sentry or Loggly.
Using Logstash
Set Up Logstash to Receive HTTP Input
Configure Logstash to accept logs via an HTTP input. Create a Logstash configuration file (e.g.,
logstash.conf
):input { http { port => 5044 codec => json } } output { elasticsearch { hosts => ["<http://localhost:9200>"] index => "client-errors" } }
- **`port`**: The port Logstash will listen to for HTTP input.
- **`codec`**: Set to `json` to process incoming JSON payloads.
Run Logstash
Start Logstash with the configuration file:
bin/logstash -f /path/to/logstash.conf
Using an HTTP Endpoint
If you don't want to use Logstash, you can set up a simple HTTP endpoint (using Node.js, for example) to receive logs and push them to Elasticsearch directly.
3. Index Logs into Elasticsearch
Ensure that your Logstash (or other logging service) is correctly configured to index the logs into Elasticsearch. This step is covered in the Logstash configuration above.
4. Visualize Logs in Kibana
- Open Kibana: Navigate to your Kibana instance.
- Create an Index Pattern:
Go to Management > Index Patterns and create a new index pattern that matches the index name used in your Logstash configuration (e.g.,
client-errors
). - Explore the Logs: Use Discover to explore your logs and ensure they are being indexed correctly.
- Create Visualizations and Dashboards: Create visualizations and dashboards to analyze and monitor JavaScript errors.
Summary
- Capture Errors: Use JavaScript to capture and send errors to your server.
- Send to Logstash: Configure Logstash to receive and forward these logs to Elasticsearch.
- Index and Visualize: Create an index pattern in Kibana to visualize and analyze the JavaScript errors.
By following these steps, you'll be able to capture JavaScript errors from the client side and visualize them in Kibana, helping you monitor and debug client-side issues effectively.
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us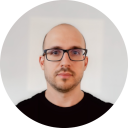
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github