How do I make a time delay in Python?
There are a few ways to make a time delay in Python. Here are three options:
time.sleep()
: You can use thesleep()
function from thetime
module to add a delay to your program. Thesleep()
function takes a single argument, which is the number of seconds to delay the program. For example:
import time
print("Hello")
time.sleep(2)
print("World")
This will print "Hello", delay for 2 seconds, and then print "World".
-
datetime.timedelta()
: You can use thetimedelta
class from thedatetime
module to represent a duration of time, and then add that duration to a specific point in time to calculate a future point in time. For example:
import datetime
current_time = datetime.datetime.now()
delay = datetime.timedelta(seconds=2)
future_time = current_time + delay
print("Hello")
while datetime.datetime.now() < future_time:
# Do nothing
print("World")
This will print "Hello", delay for 2 seconds, and then print "World".
- Loop and
time.perf_counter()
: You can use a loop and theperf_counter()
function from thetime
module to create a delay by repeatedly checking the current time and comparing it to a future point in time. For example:
import time
print("Hello")
end_time = time.perf_counter() + 2
while time.perf_counter() < end_time:
# Do nothing
print("World")
This will print "Hello", delay for 2 seconds, and then print "World".
Note that all of these options will cause the program to "block", meaning that it will not be able to do anything else while the delay is happening. If you need to be able to do other things while the delay is happening, you might want to consider using a timer or a separate thread to perform the delay.
Also, the first option is recommended if you don’t want to waste CPU time by busy waiting. The sleep function will block the current thread while it is sleeping and leaves the waking up to the scheduler.
-
How to flatten a list in Python?
You can flatten a list in python using the following one-liner: flat_list = [item for sublist in l for item in sublist] In the example above, l is the list of lists that is to be flattened. The pre...
Questions -
What are metaclasses in Python?
In Python, a metaclass is the class of a class. It defines how a class behaves, including how it is created and how it manages its instances. A metaclass is defined by inheriting from the built-in ...
Questions -
How do I concatenate two lists in Python?
You can concatenate two lists in Python by using the + operator or the extend() method. Here is an example using the + operator: list1 = [1, 2, 3] list2 = [4, 5, 6] list3 = list1 + list2 print(list...
Questions -
How to Copy Files in Python?
To copy a file in Python, you can use the shutil module. Here is an example of how you can use the shutil.copy() function to copy a file: import shutil shutil.copy('/path/to/source/file', '/path/to...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us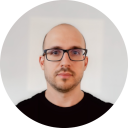
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github