How do I concatenate two lists in Python?
You can concatenate two lists in Python by using the +
operator or the extend()
method.
Here is an example using the +
operator:
list1 = [1, 2, 3]
list2 = [4, 5, 6]
list3 = list1 + list2
print(list3) # Output: [1, 2, 3, 4, 5, 6]
Here is an example using the extend()
method:
list1 = [1, 2, 3]
list2 = [4, 5, 6]
list1.extend(list2)
print(list1) # Output: [1, 2, 3, 4, 5, 6]
Note that the extend()
method modifies the list in place, whereas the +
operator creates a new list.
-
Finding the index of an item in a Python list?
To find an index of the first occurrence of an element in a given list, you can use index method of List class with the element passed as an argument. The syntax is the following: my_list = [1,2,0,...
Questions -
How to Copy Files in Python?
To copy a file in Python, you can use the shutil module. Here is an example of how you can use the shutil.copy() function to copy a file: import shutil shutil.copy('/path/to/source/file', '/path/to...
Questions -
How do I list all files in a directory using Python?
To list all files in a directory in Python, you can use the os module and its listdir() function. This function returns a list of all the files and directories in the specified directory. Here's an...
Questions -
What Does if name == “main”: Do in Python?
The if __name__ == "main" is a guarding block that is used to contain the code that should only run went the file in which this block is defined is run as a script. What it means is that if you run...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us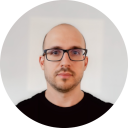
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github