How do I check if a list is empty in Python?
You can check if a list is empty by using the len()
function to check the length of the list. If the length of the list is 0, then it is empty.
Here's an example:
my_list = []
if len(my_list) == 0:
print("The list is empty")
else:
print("The list is not empty")
You can also use the following concise syntax to check if a list is empty:
if not my_list:
print("The list is empty")
else:
print("The list is not empty")
Both of these methods will work to check if a list is empty.
-
How can I safely create a nested directory in Python?
The most common way to safely create a nested directory in Python is using the pathlib or os modules. Using pathlib You can create a nested directory in python 3.5 or later using the Path and mkdir...
Questions -
How do I get the current time in Python?
There are two ways you can get the current time in python. using the datetime object using the time module Using the datetime object First, you need to import the datetime module. Then by calling t...
Questions -
Understanding slicing in Python?
Slicing is a way of extracting a specific part of an array. The syntax is following: mylist[start:end] # items start through end-1 mylist[start:] # items start through the rest of the array ...
Questions -
What are metaclasses in Python?
In Python, a metaclass is the class of a class. It defines how a class behaves, including how it is created and how it manages its instances. A metaclass is defined by inheriting from the built-in ...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us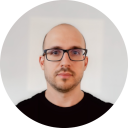
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github