Finding the Index of an Item in a Python List?
To find an index of the first occurrence of an element in a given list, you can use index
method of List
class with the element passed as an argument.
The syntax is the following:
my_list = [1,2,0,4,5,6,7,0,9]
my_list.index(0) # returns 2
You can also specify the start
and end
of the search area:
my_list.index(0, 3, 8) # returns 7
In the first example, we are looking for the first occurrence of 0 in the entire list from the start of the list to the end of the list. The first occurrence of 0 is at index 2, therefore 2 is returned.
In the second example, we specify that we want to start at index 3 and search up to index 8. Therefore the index 7 of the second 0 is returned because the first 0 is outside of the search area.
-
Difference between static and class methods in Python?
Class method To create a class method, use the @classmethod decorator. Class methods receive the class as an implicit first argument, just like an instance method receives the instance. The class m...
Questions -
How to flatten a list in Python?
You can flatten a list in python using the following one-liner: flat_list = [item for sublist in l for item in sublist] In the example above, l is the list of lists that is to be flattened. The pre...
Questions -
How can I safely create a nested directory in Python?
The most common way to safely create a nested directory in Python is using the pathlib or os modules. Using pathlib You can create a nested directory in python 3.5 or later using the Path and mkdir...
Questions -
What Does the “yield” Keyword Do in Python?
To better understand what yield does, you need first to understand what generator and iterable are. What is iterable When you use a list or list-like structure, you can read the values from the lis...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us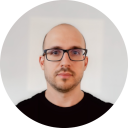
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github