Fuzz testing, or fuzzing, is a powerful automated testing technique that helps developers discover vulnerabilities and bugs by feeding random, unexpected, or malformed inputs to an application.
This approach excels at finding edge cases and security flaws that traditional testing methods might miss. Whether you're developing a web application, embedded system, or desktop software, understanding and implementing fuzz testing can significantly improve your code's robustness and security.
What is fuzz testing?
Software testing traditionally focuses on verifying that systems work correctly with valid inputs. But what about invalid, unexpected, or malicious inputs? This is where fuzz testing shines. The concept is deceptively simple: bombard your software with random, unexpected, or malformed data and see what breaks.
Fuzzing originated in the late 1980s when Professor Barton Miller at the University of Wisconsin-Madison tasked his students with testing Unix utilities by feeding them random inputs. To everyone's surprise, they managed to crash about a third of the programs tested. Since then, fuzzing has evolved into a sophisticated technique employed by security researchers, software developers, and quality assurance teams worldwide.
Modern software faces an increasingly hostile environment where a single vulnerability can lead to data breaches, system compromises, or service disruptions. Fuzzing helps identify these issues before they reach production, making it an essential part of any comprehensive security strategy.
Fundamentals of fuzz testing
Before diving into practical implementation, let's understand the core concepts that make fuzz testing effective.
At its heart, fuzzing involves:
- Test case generation: Creating inputs that will be fed to the target program
- Test execution: Running the target program with the generated inputs
- Monitoring: Watching for crashes, hangs, memory leaks, or other unexpected behaviors
- Crash analysis: Determining what went wrong when a test fails
A successful fuzzing campaign typically finds numerous edge cases where your code fails to handle inputs properly. These might range from simple crashes due to unhandled exceptions to more serious security vulnerabilities like buffer overflows or code injection opportunities.
Types of fuzzing
Fuzz testing comes in several flavors, each with its strengths and use cases:
1. Mutation-based vs. generation-based fuzzing
Mutation-based fuzzing starts with valid inputs and modifies them to create test cases. This approach works well when you already have examples of valid inputs, like a collection of image files for testing an image parser. The fuzzer randomly flips bits, deletes chunks, or otherwise mutates the sample inputs.
For example, if you're testing a JSON parser, a mutation-based fuzzer might start with a valid JSON document and then:
- Remove closing brackets
- Change string values to extremely long strings
- Replace numbers with special characters
- Duplicate key names
Generation-based fuzzing, on the other hand, creates test inputs from scratch based on a specification or model of the expected input format. This approach typically requires more setup but can achieve better coverage, especially for complex input formats.
2. Black-box, white-box, and gray-box fuzzing
These categories describe how much information the fuzzer has about the target:
Black-box fuzzing treats the application as a complete unknown. The fuzzer only knows what inputs it can provide and what outputs or behaviors result. This is simplest to set up but may miss deeper bugs.
White-box fuzzing uses detailed knowledge of the application's internals, often including source code access and program analysis. This approach can target specific vulnerabilities but requires more sophisticated tooling.
Gray-box fuzzing sits in the middle, using some knowledge of the program's structure without requiring full white-box analysis. Modern fuzzers often use instrumentation to gather execution feedback without needing source code access.
Common targets for fuzzing
Some software components benefit more from fuzzing than others:
- File parsers and format handlers: PDF readers, image processors, multimedia codecs.
- Network protocol implementations: HTTP servers, DNS resolvers, Bluetooth stacks.
- Command-line interfaces: Shell utilities, configuration tools.
- API endpoints: Web services, remote procedure calls.
- Database query processors: SQL or NoSQL query engines.
- Browser engines: JavaScript interpreters, HTML parsers, CSS processors.
Any component that processes external, especially user-supplied, input is a prime candidate for fuzzing.
Getting started with fuzz testing
Let's transition from theory to practice by setting up a basic fuzzing environment.
For beginners, I recommend starting with AFL (American Fuzzy Lop) or libFuzzer, both powerful and widely-used fuzzers. We'll use AFL for our examples because it's relatively easy to get started with and works well for many applications.
First, install AFL on your system:
sudo apt-get install afl # For Debian/Ubuntu
or
brew install afl-fuzz # For macOS
After installation, you'll need to compile your target program with AFL's instrumentation. This allows AFL to monitor your program's execution paths and make smarter decisions about test case generation.
export CC=afl-gcc
export CXX=afl-g++
./configure # If your project uses configure
make
Choosing the right fuzzing tools
While AFL is excellent for general-purpose fuzzing, several other tools might better fit specific scenarios:
- libFuzzer: Integrated with LLVM, excellent for fuzzing libraries
- Radamsa: Works well when you have a collection of valid inputs
- Peach Fuzzer: Commercial option with protocol awareness
- SPIKE: Specialized for network protocol fuzzing
- jsfuzz: Targets JavaScript code
For web applications, consider tools like OWASP ZAP or Burp Suite's Intruder, which can fuzz HTTP parameters, headers, and form inputs.
Identifying good fuzzing targets
Look for code that:
- Processes external input (files, network data, user input)
- Contains parsing logic
- Has a history of security issues
- Uses low-level memory functions
- Employs complex state machines
Start with a small, self-contained component rather than trying to fuzz an entire application at once. This makes it easier to set up the fuzzing environment and analyze results.
Practical examples
Let's walk through two concrete examples to demonstrate fuzzing in action.
Example 1: Fuzzing a simple file parser
Consider a simple program that parses a custom file format. The program might have vulnerabilities like buffer overflows if it doesn't properly validate input lengths.
Here's our vulnerable C program that we'll target with fuzzing:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// A simple function that parses a custom file format
// The format is: [4-byte length][data]
void parse_file(const char *filename) {
FILE *file = fopen(filename, "rb");
if (!file) {
printf("Could not open file %s\n", filename);
return;
}
// Read the length prefix
unsigned int length;
if (fread(&length, sizeof(length), 1, file) != 1) {
printf("Could not read length prefix\n");
fclose(file);
return;
}
// Unsafe: No validation on length
char *buffer = (char *)malloc(length);
// Vulnerable: No null termination guaranteed
if (fread(buffer, 1, length, file) != length) {
printf("Could not read data\n");
free(buffer);
fclose(file);
return;
}
printf("Successfully parsed file with length %u\n", length);
// Process the data (example: print as string)
printf("Data: %s\n", buffer);
free(buffer);
fclose(file);
}
int main(int argc, char **argv) {
if (argc != 2) {
printf("Usage: %s <filename>\n", argv[0]);
return 1;
}
parse_file(argv[1]);
return 0;
}
This program has several vulnerabilities:
- It doesn't validate the length value before allocating memory.
- It assumes the data is a null-terminated string.
- It doesn't check for integer overflows.
Let's compile this code with AFL instrumentation and prepare it for fuzzing:
afl-gcc -o parser parser.c
Next, we need sample inputs to start our fuzzing campaign. Let's create a valid input file:
mkdir -p testcases
printf "\x04\x00\x00\x00test" > testcases/sample1
This creates a file with a 4-byte length value (4 in little-endian) followed by the string "test".
Now, let's create a directory to store the fuzzing results:
mkdir -p findings
With everything prepared, we can start the fuzzing process:
afl-fuzz -i testcases -o findings -- ./parser @@
The @@
symbol tells AFL to replace it with the name of the input file it
generates for each test case.
After running for a while (possibly hours or days, depending on your code complexity), AFL will likely find several inputs that crash our program. Let's look at what might happen:
When AFL finds crashes, it stores the inputs that caused them in the
findings/crashes
directory. We can examine these files to understand what went
wrong:
xxd findings/crashes/id:000000,sig:11,src:000023,time:12345
00000000: ffff ffff 0a .....
This crash likely occurred because our program tried to allocate a huge amount of memory (0xffffffff bytes) without validation. Let's fix some of the vulnerabilities in our code:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <stdint.h>
void parse_file(const char *filename) {
FILE *file = fopen(filename, "rb");
if (!file) {
printf("Could not open file %s\n", filename);
return;
}
// Read the length prefix
uint32_t length;
if (fread(&length, sizeof(length), 1, file) != 1) {
printf("Could not read length prefix\n");
fclose(file);
return;
}
// Safety check: Validate length
if (length > 1024 * 1024) { // 1MB max
printf("Invalid length: %u\n", length);
fclose(file);
return;
}
// Allocate buffer with extra byte for null termination
char *buffer = (char *)malloc(length + 1);
if (!buffer) {
printf("Memory allocation failed\n");
fclose(file);
return;
}
// Read data
size_t read_bytes = fread(buffer, 1, length, file);
if (read_bytes != length) {
printf("Could not read data (expected %u, got %zu bytes)\n",
length, read_bytes);
free(buffer);
fclose(file);
return;
}
// Ensure null termination for string operations
buffer[length] = '\0';
printf("Successfully parsed file with length %u\n", length);
// Process the data
printf("Data: %s\n", buffer);
free(buffer);
fclose(file);
}
int main(int argc, char **argv) {
if (argc != 2) {
printf("Usage: %s <filename>\n", argv[0]);
return 1;
}
parse_file(argv[1]);
return 0;
}
Recompile with AFL instrumentation and run the fuzzer again to see if our fixes resolved the issues:
afl-gcc -o parser_fixed parser_fixed.c
afl-fuzz -i testcases -o findings_fixed -- ./parser_fixed @@
Example 2: API fuzzing for a web application
Let's look at a different example: fuzzing a REST API. For this, we'll use a simple Node.js Express API and a JavaScript-based fuzzer.
Here's a simple Express API with potential vulnerabilities:
const express = require('express');
const app = express();
const port = 3000;
app.use(express.json());
// Database of users (in-memory for this example)
const users = [
{ id: 1, username: "admin", email: "admin@example.com" },
{ id: 2, username: "user", email: "user@example.com" }
];
// Vulnerable search endpoint
app.get('/api/users/search', (req, res) => {
const query = req.query.q;
// Vulnerable: no input validation
if (!query) {
return res.status(400).json({ error: 'Search query required' });
}
try {
// Vulnerable: using regex without validation could lead to ReDoS
const regex = new RegExp(query);
const results = users.filter(user =>
regex.test(user.username) || regex.test(user.email)
);
return res.json(results);
} catch (error) {
return res.status(500).json({ error: 'Server error' });
}
});
// User creation endpoint
app.post('/api/users', (req, res) => {
const { username, email } = req.body;
// Vulnerable: insufficient validation
if (!username || !email) {
return res.status(400).json({ error: 'Username and email required' });
}
// Create new user
const newId = users.length > 0 ? Math.max(...users.map(u => u.id)) + 1 : 1;
const newUser = { id: newId, username, email };
users.push(newUser);
return res.status(201).json(newUser);
});
app.listen(port, () => {
console.log(`API server running on port ${port}`);
});
For this API, we'll use a custom JavaScript fuzzer that targets both endpoints. Let's create a simple fuzzer using Node.js:
const axios = require('axios');
const fs = require('fs');
const BASE_URL = 'http://localhost:3000';
const NUM_TESTS = 1000;
const LOG_FILE = 'fuzzing_results.txt';
// Payload generators
function generateRandomString(length) {
const chars = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789!@#$%^&*()_+-=[]{}|;:,.<>?';
let result = '';
for (let i = 0; i < length; i++) {
result += chars.charAt(Math.floor(Math.random() * chars.length));
}
return result;
}
function generateRegexPayloads() {
return [
'.*',
'(a+)+b', // ReDoS payload
'[a-zA-Z0-9]{100}',
'\\w+@\\w+\\.\\w+',
generateRandomString(50),
'(x+x+)+y', // Another ReDoS payload
'\\d{1000}',
JSON.stringify({ key: 'value' }), // JSON in a string
'\\', // Unescaped backslash
'(', // Unclosed parenthesis
')' // Unopened parenthesis
];
}
function generateUserPayloads() {
return [
{},
{ username: null, email: null },
{ username: '', email: '' },
{ username: generateRandomString(10000), email: 'test@example.com' },
{ username: 'valid', email: generateRandomString(10000) },
{ username: '<script>alert(1)</script>', email: 'test@example.com' },
{ username: 'valid', email: '<script>alert(1)</script>' },
{ username: { nested: 'object' }, email: 'test@example.com' },
{ username: 'valid', email: { nested: 'object' } },
{ username: 'valid', email: 'test@example.com', extraField: 'should be ignored' },
{ username: 'valid', email: 'test@example.com'.repeat(100) }
];
}
// Fuzz the search endpoint
async function fuzzSearchEndpoint() {
const payloads = generateRegexPayloads();
const results = [];
for (const payload of payloads) {
try {
console.log(`Testing search with: ${payload}`);
const startTime = Date.now();
const response = await axios.get(`${BASE_URL}/api/users/search`, {
params: { q: payload },
timeout: 5000 // 5 second timeout
});
const endTime = Date.now();
results.push({
payload,
statusCode: response.status,
responseTime: endTime - startTime,
responseSize: JSON.stringify(response.data).length,
error: null
});
} catch (error) {
results.push({
payload,
statusCode: error.response?.status || 0,
responseTime: 0,
responseSize: 0,
error: error.message
});
}
}
return results;
}
// Fuzz the user creation endpoint
async function fuzzUserCreationEndpoint() {
const payloads = generateUserPayloads();
const results = [];
for (const payload of payloads) {
try {
console.log(`Testing user creation with: ${JSON.stringify(payload)}`);
const startTime = Date.now();
const response = await axios.post(`${BASE_URL}/api/users`, payload, {
timeout: 5000 // 5 second timeout
});
const endTime = Date.now();
results.push({
payload: JSON.stringify(payload),
statusCode: response.status,
responseTime: endTime - startTime,
responseSize: JSON.stringify(response.data).length,
error: null
});
} catch (error) {
results.push({
payload: JSON.stringify(payload),
statusCode: error.response?.status || 0,
responseTime: 0,
responseSize: 0,
error: error.message
});
}
}
return results;
}
// Main fuzzing function
async function runFuzzing() {
console.log('Starting API fuzzing...');
// Fuzz both endpoints
const searchResults = await fuzzSearchEndpoint();
const userResults = await fuzzUserCreationEndpoint();
// Log results
const allResults = [...searchResults, ...userResults];
fs.writeFileSync(LOG_FILE, JSON.stringify(allResults, null, 2));
// Print summary
console.log('\nFuzzing completed!');
console.log(`Total tests: ${allResults.length}`);
const errors = allResults.filter(r => r.error !== null);
console.log(`Tests with errors: ${errors.length}`);
const slowResponses = allResults.filter(r => r.responseTime > 1000);
console.log(`Slow responses (>1s): ${slowResponses.length}`);
if (errors.length > 0) {
console.log('\nSample errors:');
errors.slice(0, 5).forEach(e => {
console.log(`- Payload: ${e.payload}, Error: ${e.error}`);
});
}
console.log(`\nDetailed results saved to ${LOG_FILE}`);
}
// Run the fuzzer
runFuzzing().catch(console.error);
To use this fuzzer:
- Start the Express API server:
node app.js
- In a separate terminal, run the fuzzer:
node api_fuzzer.js
The fuzzer will generate various payloads to test both endpoints and record the results. After running, we'll analyze the findings to identify vulnerabilities.
Based on the fuzzing results, we might discover:
- The search endpoint is vulnerable to Regular Expression Denial of Service (ReDoS) attacks.
- The API doesn't properly validate input types.
- The server might crash with certain malformed inputs.
Let's fix the API code to address these issues:
const express = require('express');
const app = express();
const port = 3000;
app.use(express.json({ limit: '100kb' })); // Limit payload size
// Database of users (in-memory for this example)
const users = [
{ id: 1, username: "admin", email: "admin@example.com" },
{ id: 2, username: "user", email: "user@example.com" }
];
// Fixed search endpoint
app.get('/api/users/search', (req, res) => {
const query = req.query.q;
// Improved validation
if (!query || typeof query !== 'string') {
return res.status(400).json({ error: 'Valid search query string required' });
}
// Limit query length
if (query.length > 100) {
return res.status(400).json({ error: 'Search query too long' });
}
try {
// Safe approach: Use string includes instead of regex for basic search
const results = users.filter(user =>
user.username.includes(query) || user.email.includes(query)
);
return res.json(results);
} catch (error) {
console.error('Search error:', error);
return res.status(500).json({ error: 'Server error' });
}
});
// Fixed user creation endpoint
app.post('/api/users', (req, res) => {
const { username, email } = req.body;
// Improved validation
if (!username || typeof username !== 'string' || username.length > 50) {
return res.status(400).json({ error: 'Valid username required (string, max 50 chars)' });
}
if (!email || typeof email !== 'string' || email.length > 100 || !email.includes('@')) {
return res.status(400).json({ error: 'Valid email required' });
}
// Sanitize inputs
const sanitizedUser = {
username: username.trim(),
email: email.trim()
};
// Create new user
const newId = users.length > 0 ? Math.max(...users.map(u => u.id)) + 1 : 1;
const newUser = { id: newId, ...sanitizedUser };
users.push(newUser);
return res.status(201).json(newUser);
});
// Add error handling middleware
app.use((err, req, res, next) => {
console.error('Unhandled error:', err);
res.status(500).json({ error: 'Internal server error' });
});
app.listen(port, () => {
console.log(`API server running on port ${port}`);
});
The fixed version:
- Adds proper input validation and sanitization
- Limits input lengths to prevent DoS attacks
- Uses safer alternatives to regular expressions
- Adds global error handling middleware
- Limits request body size
Common challenges and solutions
As you incorporate fuzzing into your development process, you'll likely encounter several common challenges.
Dealing with false positives
Fuzzers can generate many results that aren't actual bugs or security issues. To manage this:
- Prioritize by severity: Focus first on crashes, hangs, and memory corruption issues.
- Deduplicate crashes: Many different inputs might trigger the same underlying issue.
- Verify findings manually: Confirm that reported issues are genuine vulnerabilities before investing in fixes.
- Use minimization tools: Most fuzzers have tools to reduce test cases to their minimal form, making issues easier to understand.
For example, with AFL, you can use the afl-tmin
tool to minimize a crashing
input:
afl-tmin -i findings/crashes/crash_file -o minimized_crash -- ./target @@
Handling crashes and timeouts
When your fuzzer finds crashes or hangs:
- Collect debug information: Use tools like ASAN (Address Sanitizer) to get detailed information about memory issues.
- Set appropriate timeouts: Too short, and you'll miss slow-path bugs; too long, and your fuzzing campaign will be inefficient.
- Use checkpoints: For long-running applications, consider adding checkpointing to speed up testing.
For example, compiling with ASAN to catch memory errors:
AFL_USE_ASAN=1 afl-gcc -o target_asan target.c
Improving fuzzing coverage
To maximize the effectiveness of your fuzzing:
- Use coverage-guided fuzzers: Tools like AFL and libFuzzer use program instrumentation to track which code paths have been executed.
- Start with diverse seed inputs: Provide a variety of valid inputs that exercise different program behaviors.
- Combine fuzzing with other techniques: Use symbolic execution or concolic testing alongside fuzzing for better results.
- Focus on input handling code: Concentrate fuzzing efforts on code that processes external inputs.
You can view coverage information from your AFL campaign:
afl-cov -d findings/ --coverage-cmd "./target @@" --code-dir ./src/
Integrating fuzzing into CI/CD pipelines
For continuous fuzzing:
- Set up automated fuzzing jobs: Run short fuzzing sessions on each commit or pull request.
- Maintain a corpus of test cases: Save and reuse interesting inputs to avoid rediscovering the same paths.
- Establish failure criteria: Define when a fuzzing finding should block a release.
- Schedule longer fuzzing runs: Run comprehensive fuzzing campaigns weekly or monthly.
Here's an example GitHub Actions workflow for continuous fuzzing:
name: Continuous Fuzzing
on:
push:
branches: [ main ]
pull_request:
branches: [ main ]
jobs:
fuzz:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Install dependencies
run: |
sudo apt-get update
sudo apt-get install -y afl++
- name: Build with instrumentation
run: |
export CC=afl-gcc
export CXX=afl-g++
make
- name: Run fuzzer (short session)
run: |
mkdir -p findings
timeout 10m afl-fuzz -i testcases -o findings -- ./target @@
- name: Check for crashes
run: |
if [ -n "$(ls -A findings/crashes 2>/dev/null)" ]; then
echo "Fuzzing found crashes:"
ls -la findings/crashes/
exit 1
else
echo "No crashes found during fuzzing"
fi
Final thoughts
Fuzz testing represents one of the most effective techniques for discovering hidden bugs and security vulnerabilities in your code. By embracing randomness and the unexpected, you gain insights into edge cases that deterministic testing might miss. Starting with simple tools like AFL or libFuzzer, even beginners can quickly set up effective fuzzing campaigns that yield valuable results.
Remember that fuzzing isn't a replacement for other testing approaches but a powerful complement. Combine it with unit tests, integration tests, and manual code reviews for comprehensive quality assurance. As you gain experience, you'll develop intuition about which components benefit most from fuzzing and how to optimize your fuzzing strategy for maximum effectiveness.
The investment in learning fuzzing pays dividends in more robust, secure code – and might just save you from that dreaded 3 AM production emergency call.
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us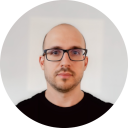
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github