Conda vs Pip: Choosing the Right Python Package Manager
Two main tools are commonly used for managing Python packages: Pip and Conda. Both help with managing dependencies, but they work in different ways.
Pip is Python's default package installer, focusing specifically on Python packages from the Python Package Index (PyPI). It's lightweight, bundled with Python, and excels at managing pure Python dependencies.
Conda takes a broader approach as a cross-platform package manager that handles packages beyond just Python. Created by Anaconda, Inc., it manages virtual environments and packages for multiple languages, making it particularly valuable for scientific computing and data science.
This article compares their approaches, strengths, and ideal use cases to help you decide which package manager best suits your project needs.
What is Pip?
Pip is Python's official package installer, providing a straightforward way to install packages from PyPI (Python Package Index). It focuses solely on Python packages and comes pre-installed with Python distributions.
Developed by the Python Packaging Authority (PyPA), Pip has been the standard way to install Python packages since 2008. It uses a simple command-line interface to install, upgrade, and remove Python packages, resolving dependencies as needed.
Pip maintains a focused approach on Python packages unlike more complex package managers. It handles wheel and source distribution installation, supports reproducibility requirements files, and integrates with virtual environments for project isolation.
What is Conda?
Conda is a cross-platform package manager and environment management system created by Anaconda, Inc. Unlike Pip's Python-specific focus, Conda handles packages for multiple languages and provides integrated environment management.
Initially developed for scientific computing needs, Conda addresses complex dependency challenges by treating Python itself as a package. This approach allows Conda to manage non-Python libraries and binaries that many scientific packages depend on, such as C libraries, R packages, and system-level dependencies.
With environment management built in, Conda simplifies creating isolated spaces for different projects, each with their own dependencies and even Python versions. Its robust dependency resolver ensures compatibility across the entire environment, not just among Python packages.
Conda vs Pip: a quick comparison
Your choice between these package managers impacts development workflow, dependency management, and deployment. Each is designed with distinct principles, making them suitable for different scenarios.
The following comparison highlights key differences to consider:
Feature | Conda | Pip |
---|---|---|
Primary focus | Language-agnostic package management | Python-specific package installation |
Package source | Anaconda repository and custom channels | Python Package Index (PyPI) |
Environment management | Built-in (conda create/activate) | Requires separate tools (virtualenv/venv) |
Dependency resolution | SAT solver for complex constraint solving | Simpler resolution, improving in newer versions |
Binary package handling | Pre-built binaries for many platforms | Relies on wheels when available |
Non-Python dependencies | Handles C libraries and system dependencies | Limited to Python packages |
Installation scope | Requires separate installation | Comes bundled with Python |
Upgrade handling | Updates entire environment consistently | Updates packages individually |
Configuration | Environment YAML files | Requirements.txt files |
Storage efficiency | Larger disk footprint | Smaller disk footprint |
Corporate/enterprise use | Commercial support available | Community support |
Data science integration | Optimized for scientific packages | General Python package management |
Learning curve | Steeper with more concepts | Gentler, more focused commands |
Package creation | Conda recipe system | Standard setuptools/packaging |
Installation and setup
The initial experience with a package manager shapes your workflow. Conda and Pip have different installation processes that reflect their different scopes and philosophies.
Pip comes bundled with Python, immediately available after a Python installation. This built-in availability means you can start installing packages right away without additional setup:
# Check pip version
pip --version
# Install a package
pip install numpy
# Upgrade pip itself
python -m pip install --upgrade pip
For environment isolation, Pip is typically paired with Python's built-in venv module or the third-party virtualenv tool:
# Create a virtual environment with venv
python -m venv myproject_env
# Activate the environment (Windows)
myproject_env\Scripts\activate
# Activate the environment (macOS/Linux)
source myproject_env/bin/activate
# Install packages in the isolated environment
pip install pandas matplotlib
Pip's approach keeps things lightweight but requires combining multiple tools for a complete workflow.
Conda requires a separate installation, typically through the Anaconda distribution (which includes many pre-installed scientific packages) or the lighter-weight Miniconda (which includes just Conda and its dependencies):
# Check conda version
conda --version
# Update conda itself
conda update conda
# Create an environment
conda create --name myproject_env
# Activate the environment
conda activate myproject_env
# Install packages
conda install numpy pandas matplotlib
Conda's installation is more involved initially, but it provides an integrated approach to both package and environment management. You get a consistent interface for creating environments, activating them, and installing packages—all through a single tool.
Environment management
Managing isolated environments for projects is essential for reproducibility and avoiding dependency conflicts. Conda and Pip take fundamentally different approaches to this challenge.
Pip doesn't include built-in environment management, instead relying on Python's virtual environment capabilities. The typical workflow involves creating a virtual environment, activating it, and then using Pip within that environment:
# Create a virtual environment
python -m venv projectA_env
# Activate the environment
source projectA_env/bin/activate # Unix/macOS
projectA_env\Scripts\activate # Windows
# Install packages
pip install tensorflow
# Save environment packages
pip freeze > requirements.txt
# Recreate environment elsewhere
pip install -r requirements.txt
# Deactivate when finished
deactivate
This separation of concerns keeps each tool focused but requires learning multiple commands and patterns. The requirements.txt
file becomes the key to reproducing environments, though it only lists Python packages, not the Python version or system dependencies.
Conda integrates environment management directly, treating environments as first-class concepts:
# Create environment with specific Python version
conda create --name projectB_env python=3.9
# Activate the environment
conda activate projectB_env
# Install packages
conda install scikit-learn pandas
# Save environment definition
conda env export > environment.yml
# Recreate environment from file
conda env create -f environment.yml
# Deactivate environment
conda deactivate
Conda's integrated approach simplifies the workflow with consistent commands. The environment.yml
file captures not just the packages but also channels, Python version, and platform-specific dependencies, making environment reproduction more reliable across different systems.
Here's an example environment.yml
file:
name: machine_learning
channels:
- conda-forge
- defaults
dependencies:
- python=3.9
- numpy=1.21
- pandas=1.3
- scikit-learn=1.0
- matplotlib=3.4
- pip:
- tensorflow==2.6.0
Conda's unified approach to environment management provides a smoother experience, especially for projects with complex dependencies across multiple languages.
Package installation and dependency resolution
The heart of any package manager is how it installs software and resolves dependencies. This is where Conda and Pip reveal their most significant philosophical differences.
Pip uses a relatively straightforward dependency resolver to install Python packages from PyPI. It processes dependencies sequentially, installing each package and its dependencies in turn:
# Basic package installation
pip install requests
# Install specific version
pip install numpy==1.21.0
# Install with version constraints
pip install "pandas>=1.3.0,<1.4.0"
# Upgrade a package
pip install --upgrade matplotlib
# Install development dependencies
pip install -e .
Pip's approach is direct and works well for many Python projects. However, it can sometimes struggle with complex dependency networks, potentially leading to conflicts. Recent versions have improved the resolver, but challenges remain, especially with packages that have complex version constraints.
For large projects, a requirements.txt
file manages dependencies:
# requirements.txt
numpy==1.21.0
pandas>=1.3.0,<1.4.0
matplotlib>=3.4.0
scikit-learn==1.0.0
Conda uses a more sophisticated constraint solver (a SAT solver) to determine a compatible set of packages before any installation begins. This approach handles complex dependency networks more reliably:
# Basic package installation
conda install requests
# Install from specific channel
conda install -c conda-forge opencv
# Install multiple packages
conda install numpy pandas matplotlib
# Install specific version
conda install python=3.8 numpy=1.21
# Update all packages
conda update --all
Conda's dependency resolution considers the entire environment at once, including non-Python dependencies and packages from different languages. This comprehensive approach is particularly valuable for scientific computing, where Python packages often depend on compiled C/C++ libraries.
A key strength of Conda is its ability to install pre-compiled binary packages for multiple platforms, avoiding the need to compile from source:
# Install a package with complex dependencies
conda install pytorch cudatoolkit=11.3 -c pytorch
This command installs PyTorch with the appropriate CUDA dependencies—something that would be considerably more complex with Pip, potentially requiring manual installation of system libraries.
Package sources and availability
Where packages come from and what types are available significantly influences which package manager best suits your needs.
Pip installs packages exclusively from the Python Package Index (PyPI), a vast repository of Python packages maintained by the Python community. This focused approach means virtually every pure Python package is available:
# Search for packages
pip search tensorflow # Note: search functionality is currently disabled in pip
# Show package information
pip show numpy
# List installed packages
pip list
# Install from alternative sources
pip install git+https://github.com/user/project.git
pip install https://example.com/packages/some-package.tar.gz
pip install -e /path/to/local/project/
Pip's integration with PyPI provides access to over 350,000 Python packages, covering nearly every Python use case. However, Pip primarily handles Python-specific packages and relies on wheels (pre-compiled binaries) when available or falls back to building from source.
Conda pulls packages from its own repositories, primarily Anaconda's default repository and community channels like conda-forge:
# List available channels
conda config --show channels
# Add a channel
conda config --add channels conda-forge
# Search for a package
conda search numpy
# Install from a specific channel
conda install -c bioconda biopython
# List installed packages
conda list
Conda repositories contain fewer packages than PyPI overall but include many non-Python dependencies crucial for scientific computing. The conda-forge channel has significantly expanded package availability, though some newer or niche Python packages might still only be available on PyPI.
Conda can also install Pip packages when needed, providing access to both ecosystems:
# Install pip within conda environment
conda install pip
# Use pip within the conda environment
pip install some-package-only-on-pypi
This hybrid approach allows Conda to manage the core environment while still accessing PyPI-only packages, though mixing package managers can occasionally lead to conflicts.
Configuration and reproducibility
Reliable configuration and environment reproducibility are critical for team collaboration and deployment. Conda and Pip offer different approaches to these challenges.
Pip relies on requirements.txt
files to capture dependencies, a simple but effective approach for Python-only projects:
# Generate requirements from current environment
pip freeze > requirements.txt
# Install from requirements
pip install -r requirements.txt
# Create dev vs. production requirements
pip freeze > requirements-dev.txt
pip install -r requirements-prod.txt
A typical requirements.txt
file looks like this:
# requirements.txt
numpy==1.21.0
pandas==1.3.3
scikit-learn==1.0.0
matplotlib==3.4.3
For more complex projects, you might use additional tools like pip-tools to manage dependencies more effectively:
# Using pip-compile from pip-tools
pip-compile requirements.in
# Install compiled requirements
pip-sync requirements.txt
This approach works well for Python-centric projects but doesn't capture the Python version or system dependencies.
Conda uses environment.yml
files, which provide a more comprehensive environment definition:
# Export environment
conda env export > environment.yml
# Create environment from file
conda env create -f environment.yml
# Export without build numbers for better cross-platform compatibility
conda env export --no-builds > environment.yml
A conda environment file captures channels, Python version, and platform-specific details:
name: data_analysis
channels:
- conda-forge
- defaults
dependencies:
- python=3.9
- pandas=1.3
- numpy=1.21
- matplotlib=3.4
- scikit-learn=1.0
- jupyterlab=3.1
- pip:
- awscli==1.20.0
You can also create simplified environment files for better cross-platform compatibility:
# Create a more portable environment file
conda env export --from-history > environment-portable.yml
Conda's approach provides more comprehensive environment capture, particularly valuable for complex scientific applications or cross-platform development.
Practical workflows
The day-to-day usage patterns of these package managers reveal how they fit into different development workflows.
Pip excels in Python-focused development with straightforward dependency requirements. It integrates seamlessly with Python development tools and workflows:
# Common Python development workflow with pip
python -m venv venv
source venv/bin/activate
# Install development tools
pip install black pytest mypy
# Install your project in development mode
pip install -e .
# Install production dependencies
pip install -r requirements.txt
# Run tests
pytest
# Format code
black .
# Check types
mypy .
This lightweight approach works well for web development, scripting, and many application types where Python is the primary language. When integrated with tools like tox or nox, you can test across multiple Python versions:
# Testing across Python versions with tox
pip install tox
tox
Conda shines in data science, scientific computing, and cross-language projects, providing an integrated environment for complex dependencies:
# Data science workflow with conda
conda create -n data_project python=3.9
conda activate data_project
# Install scientific stack
conda install numpy pandas matplotlib scikit-learn
# Install Jupyter
conda install jupyterlab
# Install R in the same environment
conda install r-base r-essentials
# Launch Jupyter
jupyter lab
For machine learning workflows, Conda simplifies GPU integration:
# Deep learning with GPU
conda create -n tensorflow_gpu
conda activate tensorflow_gpu
# Install TensorFlow with GPU support
conda install tensorflow-gpu cudatoolkit cudnn
# Verify installation
python -c "import tensorflow as tf; print(tf.config.list_physical_devices('GPU'))"
Conda's integrated environment management is particularly valuable for reproducible research and computational notebooks:
# Reproducible research workflow
conda env create -f environment.yml
conda activate research_project
# Launch notebook server
jupyter notebook
The ability to share a single environment.yml
file that captures all dependencies, including the correct Python version and non-Python libraries, simplifies collaboration in research settings.
Final thoughts
This article compared Conda and Pip to help you choose the best package manager for your Python projects.
Pip is a lightweight, Python-focused tool perfect for web and app development. It integrates with PyPI, giving access to a vast collection of Python packages and is easy to use.
Conda, with its broader environment management and multi-language support, is ideal for data science and scientific computing. It handles complex dependencies, especially for packages with C/C++ or GPU support.
For simple Python projects, Pip with virtual environments is a good choice. For projects with complex dependencies, like data science, Conda is a stronger option. Many developers use both: Pip for Python projects and Conda for data science or scientific work. Some even combine them to manage environments with Conda and Python-specific packages with Pip. Ultimately, both are excellent tools—choose based on your specific project requirements, team expertise, and whether you need to manage dependencies beyond the Python ecosystem.
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us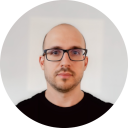
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github