Writing Log Data to Syslog Using Log4j
To write log data to syslog using Log4j, you need to configure Log4j to send log messages to a syslog server. Log4j supports syslog through appenders, which are components responsible for sending log data to different outputs.
Here's how you can configure Log4j to send log messages to syslog:
1. Add Log4j Dependency
Ensure that you have the Log4j library in your project. For Maven projects, include the dependency in your pom.xml
:
<dependency>
<groupId>org.apache.logging.log4j</groupId>
<artifactId>log4j-core</artifactId>
<version>2.x.x</version> <!-- Use the latest version -->
</dependency>
<dependency>
<groupId>org.apache.logging.log4j</groupId>
<artifactId>log4j-api</artifactId>
<version>2.x.x</version> <!-- Use the latest version -->
</dependency>
For Gradle, add the dependency to your build.gradle
:
implementation 'org.apache.logging.log4j:log4j-core:2.x.x' // Use the latest version
implementation 'org.apache.logging.log4j:log4j-api:2.x.x' // Use the latest version
2. Configure Log4j to Use Syslog Appender
You need to configure the SyslogAppender
in your Log4j configuration file. Log4j supports XML, JSON, and properties formats for configuration. Below are examples for XML and properties configurations.
XML Configuration
Create or edit the Log4j configuration file (log4j2.xml
) to include a SyslogAppender
:
<?xml version="1.0" encoding="UTF-8"?>
<Configuration status="WARN">
<Appenders>
<Syslog name="SyslogAppender" host="localhost" port="514" protocol="UDP">
<PatternLayout pattern="%d{yyyy-MM-dd HH:mm:ss} %-5level %logger{36} - %msg%n"/>
</Syslog>
</Appenders>
<Loggers>
<Root level="info">
<AppenderRef ref="SyslogAppender"/>
</Root>
</Loggers>
</Configuration>
In this configuration:
host
: The address of the syslog server. Uselocalhost
if running locally.port
: The port on which the syslog server is listening (default is514
).protocol
: The protocol used for sending logs. CommonlyUDP
, butTCP
can also be used.
Properties Configuration
For a properties-based configuration, use log4j2.properties
:
status = warn
name = PropertiesConfig
# Configure Syslog appender
appender.syslog.type = Syslog
appender.syslog.name = SyslogAppender
appender.syslog.host = localhost
appender.syslog.port = 514
appender.syslog.protocol = UDP
appender.syslog.layout.type = PatternLayout
appender.syslog.layout.pattern = %d{yyyy-MM-dd HH:mm:ss} %-5level %logger{36} - %msg%n
# Configure root logger
rootLogger.level = info
rootLogger.appenderRefs = syslog
rootLogger.appenderRef.syslog.ref = SyslogAppender
3. Log Messages to Syslog
With the configuration in place, you can log messages using Log4j in your Java application:
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
public class Example {
private static final Logger logger = LogManager.getLogger(Example.class);
public static void main(String[] args) {
logger.info("This is an informational message.");
logger.error("This is an error message.");
}
}
4. Verify Logs
On the Syslog Server: Check that your syslog server is receiving messages. For example, you can use
tail
to view the log file:tail -f /var/log/syslog
Log4j Debugging: If messages are not appearing in the syslog, enable Log4j internal debugging to troubleshoot issues:
<Configuration status="debug"> <!-- Your configuration --> </Configuration>
Additional Tips
- Syslog Server Configuration: Ensure that your syslog server is correctly configured to accept and handle incoming log messages on the specified port.
- Firewall Rules: Check that any firewalls between the application and the syslog server allow traffic on the syslog port.
- Protocol Choice: Choose between
UDP
andTCP
based on your requirements.UDP
is simpler but less reliable, whileTCP
provides guaranteed delivery.
This setup should allow you to route Log4j log messages to your syslog server, facilitating centralized log management and monitoring.
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us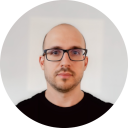
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github