When sending logs from Serilog to Logstash, you'll generally want to use a sink that can format the logs in a way that Logstash can process efficiently. For this purpose, the Serilog.Sinks.Network
package is commonly used, specifically the Tcp
or Udp
sinks, depending on your needs.
Here's how you can set up Serilog to send logs to Logstash:
1. Using Serilog.Sinks.Network
Setup for TCP
Install the Required Package:
Install the
Serilog.Sinks.Network
NuGet package in your project:dotnet add package Serilog.Sinks.Network
Configure Serilog in Your Application:
Set up Serilog to send logs via TCP to Logstash:
using Serilog; var logger = new LoggerConfiguration() .WriteTo.Console() .WriteTo.Tcp("localhost", 5044, new Serilog.Formatting.Json.JsonFormatter()) .CreateLogger(); Log.Logger = logger; Log.Information("This is a test log message");
- **`localhost`**: Replace with the IP address or hostname of your Logstash instance.
- **`5044`**: Replace with the port number on which Logstash is listening for TCP input.
- **`JsonFormatter`**: Formats the log events as JSON, which is suitable for Logstash.
Setup for UDP
If you prefer using UDP:
using Serilog;
var logger = new LoggerConfiguration()
.WriteTo.Console()
.WriteTo.Udp("localhost", 5044, new Serilog.Formatting.Json.JsonFormatter())
.CreateLogger();
Log.Logger = logger;
Log.Information("This is a test log message");
Udp
: Sends logs via UDP. Make sure that Logstash is configured to receive UDP input.
2. Logstash Configuration
Make sure Logstash is set up to receive logs via TCP or UDP. Here's a basic example configuration for TCP input:
input {
tcp {
port => 5044
codec => json_lines
}
}
output {
elasticsearch {
hosts => ["<http://localhost:9200>"]
index => "serilog-logs"
}
}
port
: Should match the port you configured in Serilog (e.g., 5044).codec
: Usejson_lines
to handle JSON-formatted log entries.
For UDP, the configuration would be:
input {
udp {
port => 5044
codec => json_lines
}
}
output {
elasticsearch {
hosts => ["<http://localhost:9200>"]
index => "serilog-logs"
}
}
Summary
Serilog.Sinks.Network
: ProvidesTcp
andUdp
sinks suitable for sending logs to Logstash.- Configuration: Ensure that Serilog and Logstash configurations match, particularly regarding the port and data format.
- Logstash Input: Configure Logstash to handle TCP or UDP input with appropriate codec settings (e.g.,
json_lines
).
By using the Serilog.Sinks.Network
package, you can efficiently stream log data from Serilog to Logstash, where it can then be processed and forwarded to Elasticsearch or other outputs.
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us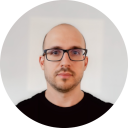
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github