What is the difference between Factory and Seeder in Laravel?
Both Factory and Seeder are used to generate testing data for your application. But there are some differences:
Factory
By using factories you can easily create test data for your Laravel application based on your Model. In Factory, we are using other classes or libraries like fzaninotto/faker
to generate fake data easily.
In Factory, we can also generate data related to the relationship while in DB Seeder we cannot do that.
// generating data based on model
factory(App\User::class, 50)->create()->each(function ($user) {
$user->posts()->save(factory(App\Post::class)->make());
});
Or different example:
use Illuminate\Support\Str;
use Faker\Generator as Faker;
// generating data using Faker
$factory->define(App\User::class, function (Faker $faker) {
return [
'name' => $faker->name,
'email' => $faker->unique()->safeEmail,
'email_verified_at' => now(),
'password' => '$2y$10$TKh8H1.PfQx37YgCzwiKb.KjNyWgaHb9cbcoQgdIVFlYg7B77UdFm', // secret
'remember_token' => Str::random(10),
];
});
Seeder
Laravel includes the ability to seed your database with data using seed classes. All seed classes are stored in the database/seeders
directory. By default, a DatabaseSeeder
class is defined for you. From this class, you may use the call
method to run other seed classes, allowing you to control the seeding order.
use Illuminate\Support\Str;
use Illuminate\Database\Seeder;
use Illuminate\Support\Facades\DB;
use App\Models\User;
class DatabaseSeeder extends Seeder
{
public function run(){
User::factory()
->count(50)
->hasPosts(1)
->create();
}
}
-
What is the difference between collection and query builder in Laravel?
Query Builder Builder is a layer of abstraction between your application and the database. Typically it's used to provide a common API for you to build platform-agnostic database queries. $users = ...
Questions -
How to Get the Query Executed in Laravel 5
By default, the query log is disabled in Laravel 5: Don’t worry, you can enable the query log by running the following: // enable query log DB::enableQueryLog(); Then you can display the log like t...
Questions -
How to log data in JSON in Laravel
Logging data in JSON format in Laravel can be done using the built-in Monolog logging library that Laravel uses under the hood. Here are the steps to configure Laravel to log data in JSON format: O...
Questions -
Unit Testing in Laravel
Testing is an important phase in the software development life cycle. It ensures that the code you've written is working as designed before you move on to the next part of your project. Every time ...
Guides
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us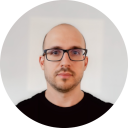
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github