What is the difference between collection and query builder in Laravel?
Query Builder
Builder is a layer of abstraction between your application and the database. Typically it's used to provide a common API for you to build platform-agnostic database queries.
$users = DB::table('users')
->where('votes', '>=', 100)
->get();
Collection
A Collection is a data structure that encapsulates a standard PHP array. It provides a chainable API for standard array_*
type PHP functions, together with other useful functions for manipulating a collection of data.
$collection = collect(str_split('AABBCCCD'));
$chunks = $collection->chunkWhile(function ($value, $key, $chunk) {
return $value === $chunk->last();
});
$chunks->all();
// [['A', 'A'], ['B', 'B'], ['C', 'C', 'C'], ['D']]
-
How to Get the Query Executed in Laravel 5
By default, the query log is disabled in Laravel 5: Don’t worry, you can enable the query log by running the following: // enable query log DB::enableQueryLog(); Then you can display the log like t...
Questions -
How to log data in JSON in Laravel
Logging data in JSON format in Laravel can be done using the built-in Monolog logging library that Laravel uses under the hood. Here are the steps to configure Laravel to log data in JSON format: O...
Questions -
Task scheduling in Laravel
Learn how to create and monitor scheduled tasks in a Laravel application
Guides -
Unit Testing in Laravel
Testing is an important phase in the software development life cycle. It ensures that the code you've written is working as designed before you move on to the next part of your project. Every time ...
Guides
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us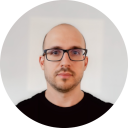
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github