What is init.py for?
In Python, the __init__.py
file is used to mark a directory as a Python package. It is used to initialize the package when it is imported.
The __init__.py
file can contain code that will be executed when the package is imported, as well as function definitions and variable assignments. It is a good place to put any code that you want to run when the package is first imported.
For example, suppose you have a package called mypackage
with the following structure:
mypackage/
__init__.py
module1.py
module2.py
...
If you want to import module1
from mypackage
, you can do so using the following import statement:
import mypackage.module1
When you run this import statement, Python will execute the code in __init__.py
before it executes the import statement for module1
. This can be useful if you want to do some initialization or setup before the other modules in the package are imported.
It is also possible to have multiple levels of packages, with __init__.py
files at each level. For example:
mypackage/
__init__.py
subpackage1/
__init__.py
module1.py
module2.py
...
subpackage2/
__init__.py
module1.py
module2.py
...
In this case, you would import a module from subpackage1
using the following import statement:
import mypackage.subpackage1.module1
Again, the code in the __init__.py
files for mypackage
and subpackage1
would be executed before the import statement for module1
is executed.
-
How to access the index in for loops in Python?
In python, if you are enumerating over a list using the for loop, you can access the index of the current value by using enumerate function. my_list = [1,2,3,4,5,6,7,8,9,10] for index, value in enu...
Questions -
How to check if a string contains a substring in Python?
There are multiple ways to check if a string contains a specific substring. Using in keyword You can use in keyword to check if a string contains a specific substring. The expression will return bo...
Questions -
How to flatten a list in Python?
You can flatten a list in python using the following one-liner: flat_list = [item for sublist in l for item in sublist] In the example above, l is the list of lists that is to be flattened. The pre...
Questions -
What Does if name == “main”: Do in Python?
The if __name__ == "main" is a guarding block that is used to contain the code that should only run went the file in which this block is defined is run as a script. What it means is that if you run...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us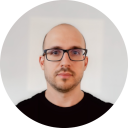
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github