What Are The 4 Types Of Metrics In Prometheus
Prometheus supports four main metric types, each suited for specific use cases. These types help capture various aspects of system performance and behavior.
1. Counter
A counter is a cumulative metric that only increases over time or resets to zero. It is ideal for tracking counts, such as the number of requests or errors.
Characteristics:
- Monotonically increasing.
- Resets only when the application restarts.
Use cases:
- Total number of HTTP requests.
- Count of failed operations.
- Number of tasks completed.
Example:
from prometheus_client import Counter
http_requests = Counter('http_requests_total', 'Total HTTP requests')
http_requests.inc() # Increment by 1
http_requests.inc(5) # Increment by 5
2. Gauge
A gauge represents a value that can go up or down. It measures instantaneous values, such as memory usage or active connections.
Characteristics:
- Can increase or decrease.
- Tracks current state at a specific moment.
Use cases:
- CPU or memory usage.
- Number of in-progress requests.
- Size of a queue.
Example:
from prometheus_client import Gauge
memory_usage = Gauge('memory_usage_bytes', 'Current memory usage in bytes')
memory_usage.set(512) # Set value
memory_usage.inc(128) # Increment
memory_usage.dec(64) # Decrement
3. Histogram
A histogram samples observations and categorizes them into predefined buckets. It is used to measure the distribution of values, such as request durations or response sizes.
Characteristics:
- Buckets define ranges for observations.
- Provides cumulative counts for each bucket and a
+Inf
bucket for all values. - Also tracks the total sum and count of observations.
Use cases:
- Measuring request latency.
- Analyzing response size distribution.
- Tracking database query durations.
Example:
from prometheus_client import Histogram
request_latency = Histogram(
'http_request_latency_seconds', 'Request latency in seconds',
buckets=[0.1, 0.5, 1, 2.5, 5, 10]
)
request_latency.observe(1.2) # Record an observation
Metrics exposed:
http_request_latency_seconds_bucket{le="0.5"} 15
http_request_latency_seconds_sum 25
http_request_latency_seconds_count 30
4. Summary
A summary is similar to a histogram but focuses on providing quantiles (e.g., 95th percentile). It tracks the total number of observations, their sum, and configurable quantile values.
Characteristics:
- Quantiles are configurable per summary.
- Does not use buckets.
- Best for small-scale scenarios where exact quantiles are needed.
Use cases:
- Measuring request latency with specific percentiles.
- Monitoring exact error rates.
Example:
from prometheus_client import Summary
request_latency = Summary('http_request_latency_seconds', 'Request latency in seconds')
request_latency.observe(1.2) # Record an observation
Comparison of metric types
Metric Type | Tracks | Can decrease | Aggregation support (e.g., rate() , sum() ) |
---|---|---|---|
Counter | Cumulative values | No | Yes |
Gauge | Instantaneous values | Yes | Yes |
Histogram | Value distributions | No | Yes |
Summary | Value distributions | No | Limited (quantiles are pre-computed) |
-
What Is A Bucket In Prometheus?
In Prometheus, a bucket is a concept used in histograms to organize observed values into predefined ranges. Buckets are critical for tracking and analyzing the distribution of values, such as respo...
Questions -
Limitations of Prometheus Labels
Prometheus labels are a powerful feature used to add dimensional data to metrics. However, improper use or lack of understanding of their limitations can lead to inefficiencies, high resource consu...
Questions -
How To Manage Prometheus Counters
Prometheus counters are metrics that only increase or reset to zero. They are ideal for tracking values like requests, errors, or completed tasks. Managing counters effectively ensures accurate and...
Questions -
What is the Difference Between a Gauge and a Counter?
Gauges and counters are two core metric types in Prometheus. They serve different purposes and are used to track different kinds of data. 1. Counter A counter is a metric that only increases over t...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us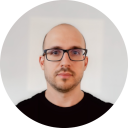
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github