User authentication in Laravel
Many web applications provide a way for their users to authenticate with the application and "login". Implementing this feature in web applications can be a complex and potentially risky endeavor. For this reason, Laravel strives to give you the tools you need to implement authentication quickly, securely, and easily.
Starter kits
First, you should install a Laravel application starter kit . Our current starter kits, Laravel Breeze and Laravel Jetstream, offer beautifully designed starting points for incorporating authentication into your fresh Laravel application.
Laravel Breeze is a minimal, simple implementation of all of Laravel's authentication features, including login, registration, password reset, email verification, and password confirmation. Laravel Breeze's view layer is made up of simple Blade templates styled with Tailwind CSS. Breeze also offers an Inertia based scaffolding option using Vue or React.
Laravel Jetstream is a more robust application starter kit that includes support for scaffolding your application with Livewire or Inertia and Vue. In addition, Jetstream features optional support for two-factor authentication, teams, profile management, browser session management, API support via Laravel Sanctum, account deletion, and more.
Retrieving authenticated user
You will often need to interact with the currently authenticated user. While handling an incoming request, you may access the authenticated user via the Auth
facade's user
method:
use Illuminate\Support\Facades\Auth;
// Retrieve the currently authenticated user...
$user = Auth::user();
// Retrieve the currently authenticated user's ID...
$id = Auth::id();
Alternatively, once a user is authenticated, you may access the authenticated user via an Illuminate\Http\Request
instance. Remember, type-hinted classes will automatically be injected into your controller methods. By type-hinting the Illuminate\Http\Request
object, you may gain convenient access to the authenticated user from any controller method in your application via the request's user
method:
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class FlightController extends Controller
{
/**
* Update the flight information for an existing flight.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function update(Request $request)
{
// $request->user()
}
}
Determining If The Current User Is Authenticated
To determine if the user making the incoming HTTP request is authenticated, you may use the check
method on the Auth
facade. This method will return true
if the user is authenticated:
use Illuminate\Support\Facades\Auth;
if (Auth::check()) {
// The user is logged in...
}
Protecting routes
Route middleware can be used to only allow authenticated users to access a given route. Laravel ships with an auth
middleware, which references the Illuminate\Auth\Middleware\Authenticate
class. Since this middleware is already registered in your application's HTTP kernel, all you need to do is attach the middleware to a route definition:
Route::get('/flights', function () {
// Only authenticated users may access this route...
})->middleware('auth');
When attaching the auth
middleware to a route, you may also specify which "guard" should be used to authenticate the user. The guard specified should correspond to one of the keys in the guards
array of your auth.php
configuration file:
Route::get('/flights', function () {
// Only authenticated users may access this route...
})->middleware('auth:admin');
Redirecting unauthenticated users
When the auth
middleware detects an unauthenticated user, it will redirect the user to the login
named route. You may modify this behavior by updating the redirectTo
function in your application's app/Http/Middleware/Authenticate.php
file:
/**
* Get the path the user should be redirected to.
*
* @param \Illuminate\Http\Request $request
* @return string
*/
protected function redirectTo($request)
{
return route('login');
}
-
What is the difference between collection and query builder in Laravel?
Query Builder Builder is a layer of abstraction between your application and the database. Typically it's used to provide a common API for you to build platform-agnostic database queries. $users = ...
Questions -
How to log data in JSON in Laravel
Logging data in JSON format in Laravel can be done using the built-in Monolog logging library that Laravel uses under the hood. Here are the steps to configure Laravel to log data in JSON format: O...
Questions -
How to populate databases in Laravel
Laravel includes the ability to seed your database with data using seed classes. All seed classes are stored in the database/seeders directory. By default, a DatabaseSeeder class is defined for you...
Questions -
What is the difference between Factory and Seeder in Laravel?
Both Factory and Seeder are used to generate testing data for your application. But there are some differences: Factory By using factories you can easily create test data for your Laravel applicati...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us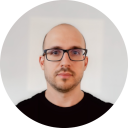
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github