In Nginx, handling and understanding the response headers from upstream servers (like application servers, APIs, etc.) is crucial for debugging, optimizing performance, and ensuring proper functionality. Here’s a detailed guide on how to manage, inspect, and troubleshoot response headers from upstream servers.
1. Understanding Response Headers
Response headers are sent by the upstream server in the HTTP response. They contain metadata about the response, such as content type, length, caching policies, and server information.
Common Response Headers:
Content-Type
: Specifies the MIME type of the content.Content-Length
: Indicates the size of the response body.Cache-Control
: Directs how the response should be cached.Expires
: Provides an expiration date for the cached response.Server
: Identifies the server software handling the request.
2. Configuring Nginx to Log Response Headers
To capture and log response headers from upstream servers, you can use Nginx’s log_format
directive to include header information in the access logs.
Example Configuration:
1. Edit Nginx Configuration File:
Open the Nginx configuration file (usually /etc/nginx/nginx.conf
or your specific server block file):
sudo nano /etc/nginx/nginx.conf
2. Define a Custom Log Format:
Add a custom log format that includes relevant response headers. You can use variables like $upstream_http_<header_name>
to log specific headers.
Example Custom Log Format:
http {
log_format main '$remote_addr - $remote_user [$time_local] "$request" '
'$status $body_bytes_sent "$http_referer" '
'"$http_user_agent" "$http_x_forwarded_for" '
'"$upstream_http_content_type" '
'"$upstream_http_content_length" '
'"$upstream_http_cache_control"';
access_log /var/log/nginx/access.log main;
# Other configurations...
}
3. Test Configuration:
Check the Nginx configuration for syntax errors:
sudo nginx -t
4. Reload Nginx:
Apply the new configuration by reloading Nginx:
sudo systemctl reload nginx
3. Viewing Response Headers
Once configured, you can view the response headers in the access logs.
Example Log Entry:
192.168.1.1 - - [26/Aug/2024:13:45:23 +0000] "GET /api/resource HTTP/1.1" 200 1234 "<http://example.com>" "Mozilla/5.0" "192.168.1.2" "application/json" "1234" "no-cache"
In this example:
application/json
is theContent-Type
header.1234
is theContent-Length
header.no-cache
is theCache-Control
header.
4. Troubleshooting Response Header Issues
If you encounter issues with response headers, consider the following steps:
- 1. *Verify Upstream Server Configuration:**
Ensure that the upstream server is correctly configured to send the desired headers.
- 2. *Inspect Headers Directly:**
Use tools like curl
, wget
, or browser developer tools to inspect the response headers directly.
Example with curl
:
curl -I <http://example.com/api/resource>
- 3. *Check Nginx Logs:**
Review Nginx’s error logs for any issues related to proxying or upstream server communication:
sudo tail -f /var/log/nginx/error.log
- 4. *Review Upstream Logs:**
Inspect the logs of the upstream server to ensure it is handling requests and generating responses as expected.
5. Advanced Header Manipulation
Nginx can also be configured to modify response headers before sending them to the client using directives like add_header
and more_set_headers
(requires the ngx_http_headers_module
).
Example: Adding Headers:
location / {
proxy_pass <http://myapp>;
add_header X-Response-Time $upstream_response_time;
}
Example: Removing Headers:
location / {
proxy_pass <http://myapp>;
more_set_headers 'X-Server-Name:';
}
Summary
- Log Response Headers: Configure
log_format
to include response headers in access logs. - View Logs: Check access logs to view response headers.
- Troubleshoot: Verify upstream server configuration, inspect headers directly, and check logs for issues.
- Advanced Manipulation: Use directives like
add_header
andmore_set_headers
for advanced header management.
By configuring Nginx to log and inspect response headers, you can gain insights into upstream server responses and troubleshoot issues effectively.
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us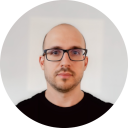
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github