Removing Old Indices in Elasticsearch
Removing old indices in Elasticsearch is important for managing disk space and maintaining optimal performance. Here are several methods to delete old indices in Elasticsearch:
1. Using the Elasticsearch REST API
Delete a Specific Index
To delete a specific index, you can use the DELETE HTTP method with the index name.
DELETE /index_name
Example using curl
:
curl -X DELETE "localhost:9200/index_name?pretty"
Replace index_name
with the name of the index you want to delete.
Delete Indices with a Date Pattern
If you have indices with a date pattern (e.g., logs-2023-01-01
), you can delete indices that match a specific pattern.
To delete indices that match a pattern, you can use wildcards:
DELETE /logs-2023-01-*
Example using curl
:
curl -X DELETE "localhost:9200/logs-2023-01-*?pretty"
Delete Indices Based on Age or Size Using Index Lifecycle Management (ILM)
You can automate index deletion based on age or size using Index Lifecycle Management (ILM). This is useful for managing large volumes of data.
Define an ILM Policy: Create an ILM policy that specifies when to delete indices.
PUT /_ilm/policy/delete-old-indices { "phases": { "hot": { "actions": {} }, "delete": { "min_age": "30d", // Age after which to delete indices "actions": { "delete": {} } } } }
Apply the ILM Policy to Indices: Set the ILM policy for your indices.
PUT /index_name/_settings { "index.lifecycle.name": "delete-old-indices" }
Verify ILM Policy: Check if the ILM policy is applied and review its status.
GET /_ilm/policy
2. Using Kibana Dev Tools
- Open Kibana: Go to the Dev Tools section in Kibana.
Execute DELETE Command: Run the DELETE command for the indices you want to remove.
DELETE /index_name
To delete multiple indices matching a pattern:
DELETE /logs-2023-01-*
Confirm Deletion: Check the status to ensure indices have been deleted.
GET /_cat/indices?v
3. Using Curator (Optional)
Elasticsearch Curator is a command-line tool for managing indices and snapshots. It is useful for automating tasks like deleting old indices.
- Install Curator: Follow the installation instructions from the Elasticsearch Curator documentation.
Create a Curator Configuration File: Define your Curator actions in a YAML file (e.g.,
delete_old_indices.yml
).actions: 1: action: delete_indices description: "Delete indices older than 30 days" options: ignore_empty_list: True allow_ilm_indices: False filters: - filtertype: age source: creation_date direction: older unit: days value: 30
Run Curator: Execute Curator with the configuration file.
curator --config /path/to/curator.yml /path/to/delete_old_indices.yml
4. Manual Deletion (CLI or Script)
You can write scripts in languages like Python using the elasticsearch-py
library to programmatically delete old indices.
Example with Python:
from elasticsearch import Elasticsearch
es = Elasticsearch(["<http://localhost:9200>"])
# Define index pattern
index_pattern = "logs-2023-01-*"
# List indices matching pattern
indices = es.cat.indices(index=index_pattern, format="json")
# Delete each index
for index in indices:
index_name = index['index']
es.indices.delete(index=index_name)
print(f"Deleted index: {index_name}")
Summary
- Direct Deletion: Use the Elasticsearch REST API to delete specific or pattern-matching indices.
- ILM: Automate index management, including deletion, based on age or size.
- Kibana Dev Tools: Perform index deletion through Kibana's built-in Dev Tools console.
- Curator: Use Curator for advanced index management and automation.
- Scripted Solutions: Write scripts in programming languages for custom deletion logic.
Choose the method that best fits your needs based on your Elasticsearch setup and data management requirements.
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us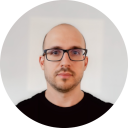
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github