How to remove an element from a list by index in Python?
To remove an element from a list by index in Python, you can use the del
statement. Here is an example:
a = [1, 2, 3, 4, 5]
del a[2]
print(a) # [1, 2, 4, 5]
The del
statement removes the element at the specified index, 2 in this case, from the list.
Keep in mind that this operation modifies the list in place and does not return a new list. If you want to remove an element from a list and get a new list with the element removed, you can use the pop()
method instead.
For example:
a = [1, 2, 3, 4, 5]
b = a.pop(2)
print(a) # [1, 2, 3, 4, 5]
print(b) # 3
The pop()
method removes the element at the specified index and returns the element. It also modifies the list in place. If you don't specify an index, it removes and returns the last element of the list by default.
-
How do I split Python list into equally-sized chunks?
To split a list into equally sized chunks, you can use the grouper function from the itertools module. Here's an example of how you can use it: from itertools import zip_longest def grouper(iterabl...
Questions -
How to flatten a list in Python?
You can flatten a list in python using the following one-liner: flat_list = [item for sublist in l for item in sublist] In the example above, l is the list of lists that is to be flattened. The pre...
Questions -
Finding the index of an item in a Python list?
To find an index of the first occurrence of an element in a given list, you can use index method of List class with the element passed as an argument. The syntax is the following: my_list = [1,2,0,...
Questions -
How do I check if a list is empty in Python?
You can check if a list is empty by using the len() function to check the length of the list. If the length of the list is 0, then it is empty. Here's an example: my_list = [] if len(my_list) == 0:...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us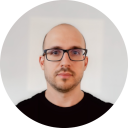
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github