How to Process POST Data in node.js?
In Node.js, you can process POST data in different ways depending on the framework or library you are using. I'll provide examples for both native HTTP and using the popular Express.js framework.
Native HTTP Module
To process POST data in a native Node.js HTTP server, you need to listen for the data
and end
events on the request
object. Here's a simple example:
const http = require('http');
const server = http.createServer((req, res) => {
if (req.method === 'POST') {
let data = '';
req.on('data', (chunk) => {
data += chunk;
});
req.on('end', () => {
// Process the POST data
console.log('Received POST data:', data);
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end('Data received successfully');
});
} else {
res.writeHead(404, { 'Content-Type': 'text/plain' });
res.end('Not Found');
}
});
const PORT = 3000;
server.listen(PORT, () => {
console.log(`Server listening on port ${PORT}`);
});
Express.js
If you're using Express.js, you can use the body-parser
middleware to parse POST data. Install it using:
npm install body-parser
Then, use it in your Express app:
const express = require('express');
const bodyParser = require('body-parser');
const app = express();
// Parse JSON and url-encoded data
app.use(bodyParser.json());
app.use(bodyParser.urlencoded({ extended: true }));
// Handle POST requests
app.post('/process-post', (req, res) => {
// Access POST data
const postData = req.body;
// Process the data
console.log('Received POST data:', postData);
res.send('Data received successfully');
});
const PORT = 3000;
app.listen(PORT, () => {
console.log(`Server listening on port ${PORT}`);
});
In this example, the body-parser
middleware is used to parse JSON and URL-encoded data sent in POST requests. The processed data is available in req.body
.
Choose the approach that fits your use case and preferences. The native approach is lightweight, while using Express.js provides a more convenient and feature-rich way to handle HTTP requests.
-
How to Read Package Version in node.js Code?
To read the package version in Node.js code, you can use the require function to import the package.json file and access the version property. Here's an example: Assuming your project structure loo...
Questions -
How is an HTTP POST request made in node.js?
In Node.js, you can make an HTTP POST request using the http or https modules that come with Node.js, or you can use a more user-friendly library like axios or node-fetch. Here, I'll provide exampl...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us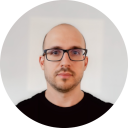
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github