How to Read Package Version in node.js Code?
To read the package version in Node.js code, you can use the require
function to import the package.json
file and access the version
property. Here's an example:
Assuming your project structure looks like this:
project-root
│ package.json
│ yourScript.js
Modify your
package.json
file to include aversion
field:{ "name": "your-project", "version": "1.0.0", "description": "Your project description", // other fields... }
In your Node.js script (
yourScript.js
), read the package version:const packageInfo = require('./package.json'); // Access the version property const version = packageInfo.version; console.log('Package version:', version);
Make sure to adjust the path in the
require
statement if your script is in a different location relative to thepackage.json
file.
Alternatively, you can use the fs
(file system) module to read the contents of the package.json
file and then parse the JSON data to retrieve the version:
const fs = require('fs');
// Read the content of package.json
const packageJsonContent = fs.readFileSync('./package.json', 'utf8');
// Parse the JSON data
const packageInfo = JSON.parse(packageJsonContent);
// Access the version property
const version = packageInfo.version;
console.log('Package version:', version);
Using require
is a more convenient and common approach, but reading the file manually gives you more flexibility in case you need to perform additional operations on the package.json
data.
-
How to install a previous exact version of a NPM package?
To install a specific version of an npm package, you can use the npm install command along with the package name and the desired version number. Here's the syntax: npm install <package_name>@<versi...
Questions -
How to find the version of an installed npm package?
To find the version of an installed npm package, you can use the following commands: To see the version of an installed Node.js or npm package, run npm list <package-name>. To see the latest versio...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us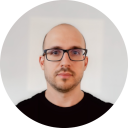
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github