Installing specific package version with pip
To install a specific version of a package with pip, you can use the ==
operator followed by the desired version number. For example:
pip install package_name==1.0.4
This will install version 1.0.4 of the package. If you want to install a version that is compatible with a certain version of Python, you can specify the Python version using the python
keyword followed by the version number. For example:
pip install package_name==1.0.4 python==3.6
This will install version 1.0.4 of the package and ensure that it is compatible with Python 3.6.
You can also use the >
and <
operators to specify a minimum or maximum version number, respectively. For example:
pip install package_name>=1.0.4
This will install the latest version of the package that is greater than or equal to 1.0.4.
Finally, you can use the ~=
operator to specify a minimum and maximum version number. For example:
pip install package_name~=1.0.4
This will install the latest version of the package that is compatible with version 1.0.4 (i.e., the package should have the same major and minor version numbers as 1.0.4, but the patch number can be different).
-
How do I uppercase or lowercase a string in Python?
To uppercase a string in Python, you can use the upper() method of the string. For example: string = "hello" uppercasestring = string.upper() print(uppercasestring) # prints "HELLO" To lowercase a...
Questions -
How do I check if a list is empty in Python?
You can check if a list is empty by using the len() function to check the length of the list. If the length of the list is 0, then it is empty. Here's an example: my_list = [] if len(my_list) == 0:...
Questions -
How to access the index in for loops in Python?
In python, if you are enumerating over a list using the for loop, you can access the index of the current value by using enumerate function. my_list = [1,2,3,4,5,6,7,8,9,10] for index, value in enu...
Questions -
How to upgrade all Python packages with pip?
You can use the pip install command with the --upgrade option to upgrade all packages in your Python environment. Here's the basic syntax: pip install --upgrade [package1] [package2] ... To upgrade...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us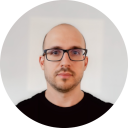
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github