How can I uninstall npm modules in Node.js?
To uninstall npm modules (packages) in Node.js, you can use the npm uninstall
command followed by the name of the module you want to remove. Here are a few examples:
Uninstalling a Local Module:
To remove a module from your project's node_modules
directory and update your package.json
:
npm uninstall <module_name>
Replace <module_name>
with the name of the module you want to uninstall.
Uninstalling a Global Module:
To remove a globally installed module:
npm uninstall -g <module_name>
Uninstalling Multiple Modules:
To uninstall multiple modules at once:
npm uninstall <module1> <module2> <module3>
Uninstalling and Saving to devDependencies
:
If you want to uninstall a module and remove it from devDependencies
in your package.json
:
npm uninstall --save-dev <module_name>
Uninstalling and Saving to dependencies
:
If you want to uninstall a module and remove it from dependencies
in your package.json
:
npm uninstall --save <module_name>
Uninstalling and Updating package.json
:
In some cases, npm might not automatically remove the entry from your package.json
file. If that happens, you can use the --save
or --save-dev
flag to ensure it is removed:
npm uninstall --save <module_name>
or
npm uninstall --save-dev <module_name>
After running any of these commands, npm will remove the specified module from your project or global installation.
Make sure to check your package.json
file and verify that the entry for the uninstalled module has been removed. If it hasn't, you may need to remove it manually.
-
How to decide when to use Node.js?
Node.js is a powerful, JavaScript-based runtime environment that has shaped the modern web landscape, enabling developers to build fast, scalable, and efficient web applications. To Node.js or not ...
Questions -
How do you get a list of the names of all files present in a directory in Node.js?
In Node.js, you can use the fs (file system) module to get a list of file names in a directory. Here's an example using the fs.readdir function: const fs = require('fs'); const directoryPath = '/pa...
Questions -
How to use multiple node versions on the same machine?
There are several ways to manage multiple Node.js versions on the same machine. Here are two popular tools for achieving this: NVM (Node Version Manager): NVM is a widely used tool for managing mul...
Questions -
What is the purpose of Node.js module.exports and how do you use it?
In Node.js, module.exports is a special object that is used to define what a module exports as its public interface. It is used to expose functionality from one module (file) to another module, all...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us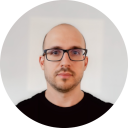
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github