How do you get a list of the names of all files present in a directory in Node.js?
In Node.js, you can use the fs
(file system) module to get a list of file names in a directory. Here's an example using the fs.readdir
function:
const fs = require('fs');
const directoryPath = '/path/to/your/directory';
// Read the contents of the directory
fs.readdir(directoryPath, (err, files) => {
if (err) {
console.error('Error reading directory:', err);
return;
}
// Log the list of file names
console.log('Files in the directory:');
files.forEach(file => {
console.log(file);
});
});
Replace '/path/to/your/directory'
with the actual path of the directory you want to list files from.
In this example:
fs.readdir
is used to read the contents of the specified directory.- The callback function receives an array of file names (
files
) or an error (err
). - If there is an error, it's logged to the console. Otherwise, it logs each file name in the directory.
Note that the file names returned by readdir
include both files and directories. If you want to filter only files, you can use the fs.stat
method to check the type of each entry.
Here's an updated example that filters only files:
const fs = require('fs');
const path = require('path');
const directoryPath = '/path/to/your/directory';
fs.readdir(directoryPath, (err, files) => {
if (err) {
console.error('Error reading directory:', err);
return;
}
// Filter only files
const fileNames = files.filter(file => {
const filePath = path.join(directoryPath, file);
return fs.statSync(filePath).isFile();
});
console.log('Files in the directory:');
fileNames.forEach(fileName => {
console.log(fileName);
});
});
In this updated example, fs.statSync(filePath).isFile()
is used to check if each entry is a file. The path.join
method is used to construct the full path to each file.
-
How do I pass command line arguments to a Node.js program and receive them?
In Node.js, you can pass command line arguments to your script the same as you would for any other command line application. Simply type your arguments after the script path separated with a space ...
Questions -
How can I update Node.js and NPM to their latest versions?
There are several ways to update Node.js to its latest version. Here are three methods: Updating Node.js Using NPM You can use NPM to update Node.js by installing the n package, which will be used ...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us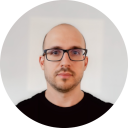
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github