How to Setup Error Reporting in Stackdriver From Kubernetes Pods?
Setting up error reporting in Google Cloud’s Stackdriver (now part of Google Cloud Operations Suite) from Kubernetes pods involves configuring your application to send errors to Google Cloud Error Reporting. This process includes setting up a Google Cloud project, enabling the required APIs, configuring your Kubernetes cluster, and modifying your application code or logging setup to report errors.
Step-by-Step Guide to Set Up Error Reporting in Stackdriver from Kubernetes Pods
Step 1: Set Up Your Google Cloud Project
- Create a Google Cloud Project if you haven't already: Create Project.
- Enable the Error Reporting API:
- Go to the APIs & Services Dashboard.
- Search for "Error Reporting API" and enable it.
Step 2: Set Up Authentication with Google Cloud
To allow your Kubernetes pods to report errors, you need to provide authentication credentials.
- Create a Service Account:
- Go to the Service Accounts page.
- Click on "Create Service Account".
- Name your service account (e.g.,
stackdriver-error-reporter
). - Assign it the role Error Reporting Writer (or Logs Writer for logging errors).
- Generate and Download a Key:
- Once the service account is created, click on it.
- Go to the "Keys" tab, click "Add Key," and select "JSON".
- Download the key file; this will be used to authenticate from your Kubernetes cluster.
Step 3: Add the Service Account Key to Kubernetes as a Secret
Upload the key to your Kubernetes cluster as a secret so that your pods can access it.
Create a Kubernetes secret with the downloaded JSON key file:
kubectl create secret generic stackdriver-key --from-file=key.json=/path/to/your/service-account-key.json
Mount the Secret in Your Pods: Update your Kubernetes pod or deployment YAML to mount the secret:
apiVersion: apps/v1 kind: Deployment metadata: name: your-app spec: replicas: 1 template: metadata: labels: app: your-app spec: containers: - name: your-app-container image: your-app-image env: - name: GOOGLE_APPLICATION_CREDENTIALS value: /secrets/google/key.json volumeMounts: - name: gcp-credentials mountPath: /secrets/google readOnly: true volumes: - name: gcp-credentials secret: secretName: stackdriver-key
Step 4: Modify Your Application to Report Errors
Your application must be configured to report errors to Stackdriver. Depending on your language and framework, you might use Stackdriver libraries or rely on logging agents.
Using Google Cloud Error Reporting Libraries
For Node.js:
Install the library:
npm install @google-cloud/error-reporting
- Use the Error Reporting client in your application:
```jsx
const { ErrorReporting } = require('@google-cloud/error-reporting');
const errors = new ErrorReporting();
// Report an error
try {
// Your code here
} catch (err) {
errors.report(err);
}
```
For Python:
Install the library:
pip install google-cloud-error-reporting
- Use the Error Reporting client in your application:
```python
from google.cloud import error_reporting
client = error_reporting.Client()
try:
# Your code here
except Exception as e:
client.report_exception()
```
For Java:
Add the dependency to your
pom.xml
:<dependency> <groupId>com.google.cloud</groupId> <artifactId>google-cloud-errorreporting</artifactId> <version>0.119.1-alpha</version> </dependency>
- Use the client in your code:
```java
ErrorReportingClient errorClient = ErrorReportingClient.create();
try {
// Your code here
} catch (Exception e) {
errorClient.reportErrorEvent(
ReportedErrorEvent.newBuilder()
.setMessage(e.getMessage())
.build()
);
}
```
Step 5: View Errors in Google Cloud Error Reporting
- Go to the Error Reporting page in Google Cloud Console.
- You should see errors reported from your application.
Troubleshooting Tips
- Ensure Service Account Permissions: Make sure the service account has appropriate permissions (Error Reporting Writer).
- Check Environment Variables: Ensure
GOOGLE_APPLICATION_CREDENTIALS
points to the correct path of your service account key. - Network Access: Your Kubernetes pods must be able to reach Google Cloud APIs (ensure there are no network restrictions).
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us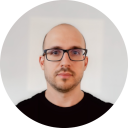
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github