How to Run Nginx Within a Docker Container Without Halting?
Running Nginx within a Docker container is a common practice, and ensuring that it continues running smoothly without halting is crucial for maintaining a stable service. Here’s a comprehensive guide to run Nginx within a Docker container effectively:
1. Create a Dockerfile for Nginx
To set up Nginx in a Docker container, you need to create a Dockerfile. This file defines the environment and commands needed to build your Docker image.
Basic Dockerfile Example:
# Use the official Nginx image from Docker Hub
FROM nginx:latest
# Copy custom configuration file (optional)
COPY nginx.conf /etc/nginx/nginx.conf
# Copy static files (optional)
COPY html/ /usr/share/nginx/html/
# Expose ports (default is 80 for HTTP and 443 for HTTPS)
EXPOSE 80
EXPOSE 443
Explanation:
FROM nginx:latest
: Uses the latest official Nginx image from Docker Hub.COPY nginx.conf /etc/nginx/nginx.conf
: (Optional) Copies a custom Nginx configuration file into the container.COPY html/ /usr/share/nginx/html/
: (Optional) Copies static files into the container's web root directory.EXPOSE 80 443
: Exposes ports 80 and 443 for HTTP and HTTPS traffic.
2. Build the Docker Image
After creating the Dockerfile, build your Docker image using the docker build
command.
Build Command:
docker build -t my-nginx-image .
Explanation:
t my-nginx-image
: Tags the image with the namemy-nginx-image
..
: Specifies the build context (current directory).
3. Run the Docker Container
Run your Docker container with the docker run
command. Ensure that it runs in the foreground to keep it active.
Run Command:
docker run -d \\
--name my-nginx-container \\
-p 80:80 \\
-p 443:443 \\
my-nginx-image
Explanation:
d
: Runs the container in detached mode (in the background).-name my-nginx-container
: Names the containermy-nginx-container
.p 80:80
: Maps port 80 on the host to port 80 in the container.p 443:443
: Maps port 443 on the host to port 443 in the container.my-nginx-image
: Specifies the Docker image to use.
4. Verify Container Status
Check if your container is running correctly.
Verify Command:
docker ps
Explanation:
- Lists all running containers and their statuses.
5. View Logs
Monitor the logs to ensure Nginx is operating correctly and troubleshoot any issues.
View Logs Command:
docker logs my-nginx-container
Explanation:
- Shows the logs for the specified container.
6. Keep the Container Running
If your container stops unexpectedly, consider the following strategies:
A. Use a Health Check
Define a health check in your Dockerfile to ensure the container is running properly.
Add to Dockerfile:
HEALTHCHECK --interval=30s --timeout=10s \\
CMD curl -f <http://localhost/> || exit 1
Explanation:
-interval=30s
: Check every 30 seconds.-timeout=10s
: Timeout after 10 seconds.CMD curl -f <http://localhost/> || exit 1
: Executes a command to check the health of the Nginx server.
B. Use Docker Restart Policies
Set a restart policy to automatically restart the container if it stops.
Run Command with Restart Policy:
docker run -d \\
--name my-nginx-container \\
--restart unless-stopped \\
-p 80:80 \\
-p 443:443 \\
my-nginx-image
Explanation:
-restart unless-stopped
: Automatically restarts the container unless it is explicitly stopped.
7. Ensure Proper Configuration
Make sure that your Nginx configuration is correct. Incorrect configurations can lead to issues. Verify the configuration inside the container:
Access Container Shell:
docker exec -it my-nginx-container /bin/bash
Check Nginx Configuration:
nginx -t
Explanation:
nginx -t
: Tests the Nginx configuration for syntax errors.
8. Monitor and Manage Resources
Ensure your container has sufficient resources. Resource constraints can lead to issues.
Check Container Resource Usage:
docker stats my-nginx-container
Explanation:
- Monitors the resource usage (CPU, memory) of your container.
Summary
To run Nginx within a Docker container without halting:
- Create a Dockerfile: Define the environment and commands for building the image.
- Build the Docker Image: Use
docker build
to create your image. - Run the Docker Container: Use
docker run
with thed
option to keep it running. - Verify Container Status: Check if the container is running using
docker ps
. - View Logs: Monitor logs with
docker logs
to troubleshoot issues. - Keep the Container Running: Use health checks and restart policies to ensure uptime.
- Ensure Proper Configuration: Verify and test Nginx configuration inside the container.
- Monitor Resources: Use
docker stats
to monitor resource usage.
By following these steps, you can effectively run and manage Nginx within a Docker container, ensuring it operates continuously and reliably.
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us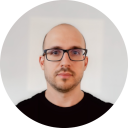
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github