How to log uncaught exceptions in C#
In C#, you can log uncaught exceptions using the AppDomain.UnhandledException
event and a logging framework like log4net or NLog. The AppDomain.UnhandledException
event allows you to handle uncaught exceptions that occur in your application's primary application domain. Here's a step-by-step guide on how to set up logging for uncaught exceptions in C#:
- Install a Logging Framework: Choose a logging framework like log4net or NLog and add it to your project using NuGet. For this example, let's use log4net.
- Configure the Logging Framework: Set up the configuration for your chosen logging framework. For log4net, you typically configure it through an XML file. Consult the documentation of the logging framework for specific configuration details.
- Subscribe to the
AppDomain.UnhandledException
event and log exceptions: In your application's entry point (usually theMain
method), subscribe to theAppDomain.UnhandledException
event. This event is triggered when an unhandled exception occurs in the application domain.
Here's an example using log4net for logging unhandled exceptions:
using System;
using System.Reflection;
using log4net;
namespace UnhandledExceptionLoggingExample
{
class Program
{
// Define a logger instance
private static readonly ILog Log = LogManager.GetLogger(MethodBase.GetCurrentMethod().DeclaringType);
static void Main(string[] args)
{
// Configure log4net (load configuration from an XML file, etc.)
log4net.Config.XmlConfigurator.Configure();
// Subscribe to the UnhandledException event
AppDomain.CurrentDomain.UnhandledException += CurrentDomain_UnhandledException;
try
{
// Your application code here...
// For testing, let's throw an exception
throw new Exception("An unhandled exception occurred!");
}
catch (Exception ex)
{
// Handle exceptions that you catch within your code
Log.Error("Caught exception:", ex);
}
}
// Event handler for unhandled exceptions
private static void CurrentDomain_UnhandledException(object sender, UnhandledExceptionEventArgs e)
{
// Log unhandled exceptions
if (e.ExceptionObject is Exception ex)
{
Log.Fatal("Unhandled exception:", ex);
}
else
{
Log.Fatal("Unhandled non-exception object:", e.ExceptionObject);
}
}
}
}
In this example, we use log4net for logging unhandled exceptions. The UnhandledException
event handler logs the unhandled exception using the logger. Additionally, we catch exceptions within the try-catch
block in the Main
method, so they are also logged. This approach ensures that both unhandled and handled exceptions are logged.
Don't forget to configure log4net according to your needs. Make sure to handle the exception carefully in the CurrentDomain_UnhandledException
event handler, as it runs in a potentially unstable state.
To learn more about logging, visit Better Stack Community.
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us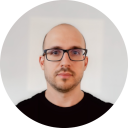
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github