In Node.js, how do I "include" functions from my other files?
In Node.js, you can include (import) functions from other files using the require
statement. Here's an example of how to include functions from another file:
Suppose you have a file named mathFunctions.js
with some functions:
// mathFunctions.js
function add(a, b) {
return a + b;
}
function subtract(a, b) {
return a - b;
}
module.exports = {
add,
subtract
};
Now, in another file (let's call it app.js
), you can include and use these functions:
// app.js
// Include (require) the mathFunctions module
const mathFunctions = require('./mathFunctions');
// Use the functions from mathFunctions module
const resultAdd = mathFunctions.add(5, 3);
const resultSubtract = mathFunctions.subtract(8, 3);
// Log the results
console.log('Addition:', resultAdd);
console.log('Subtraction:', resultSubtract);
In the example above:
require('./mathFunctions')
is used to import the module defined inmathFunctions.js
. The./
indicates that the module is in the same directory.- The imported module is assigned to the variable
mathFunctions
. - You can then use the functions from
mathFunctions
as if they were defined in the current file.
When you run app.js
, it will output:
Addition: 8
Subtraction: 5
Remember to replace the file paths with the correct paths in your actual project. The module.exports
statement in mathFunctions.js
is used to export the functions from that file, making them accessible in other files that require it.
Note: With the introduction of ECMAScript modules (ESM) in newer versions of Node.js (v13.2.0 and later), you can also use import
and export
syntax. However, the CommonJS require
and module.exports
syntax is still widely used in many Node.js projects.
-
How do you get a list of the names of all files present in a directory in Node.js?
In Node.js, you can use the fs (file system) module to get a list of file names in a directory. Here's an example using the fs.readdir function: const fs = require('fs'); const directoryPath = '/pa...
Questions -
How to read environment variables in Node.js
In Node.js, you can read environmental variables using the process.env object. This object provides access to the user environment, including environment variables. Here's a simple example of how y...
Questions -
How can I update Node.js and NPM to their latest versions?
There are several ways to update Node.js to its latest version. Here are three methods: Updating Node.js Using NPM You can use NPM to update Node.js by installing the n package, which will be used ...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us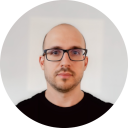
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github