How to create a singleton in Python?
The singleton pattern is a software design pattern that restricts the instantiation of a class to a singular instance.
Using metaclass
In Python, there are multiple ways to do that but the most effective is using metaclasses.
class Singleton(type):
_instances = {}
def __call__(cls, *args, **kwargs):
if cls not in cls._instances:
cls._instances[cls] = super(Singleton, cls).__call__(*args, **kwargs)
return cls._instances[cls]
class Logger(metaclass=Singleton):
pass
In the example above, the Logger class is a singleton - every instance of the Logger will be the same. There will always be only one Logger and all Logger objects will refer to that one Logger.
-
Understanding Python super() with init() methods
The super() function is used to call a method from a parent class. When used with the __init__ method, it allows you to initialize the attributes of the parent class, in addition to any attributes ...
Questions -
How Do I Measure Elapsed Time in Python?
There are several ways to measure elapsed time in Python. Here are a few options: You can use the time module to get the current time in seconds since the epoch (the epoch is a predefined point in ...
Questions -
What are metaclasses in Python?
In Python, a metaclass is the class of a class. It defines how a class behaves, including how it is created and how it manages its instances. A metaclass is defined by inheriting from the built-in ...
Questions -
How to convert string into datetime in Python?
To convert a string into a datetime object in Python, you can use the datetime.strptime() function. This function allows you to specify the format of the input string, and it will return a datetime...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us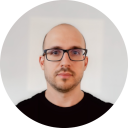
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github