How can I make a Python dictionary from separate lists of keys and values?
You can create a Python dictionary from two separate lists, where the first list contains keys and the second list contains values using the zip
function.
Here is an example of this in action:
# List of keys
keys = ['a', 'b', 'c']
# List of values
values = [1, 2, 3]
# Zip the keys and values and create a dictionary
dictionary = dict(zip(keys, values))
print(dictionary)
# Output: {'a': 1, 'b': 2, 'c': 3}
-
Finding the index of an item in a Python list?
To find an index of the first occurrence of an element in a given list, you can use index method of List class with the element passed as an argument. The syntax is the following: my_list = [1,2,0,...
Questions -
How do I list all files in a directory using Python?
To list all files in a directory in Python, you can use the os module and its listdir() function. This function returns a list of all the files and directories in the specified directory. Here's an...
Questions -
How do I concatenate two lists in Python?
You can concatenate two lists in Python by using the + operator or the extend() method. Here is an example using the + operator: list1 = [1, 2, 3] list2 = [4, 5, 6] list3 = list1 + list2 print(list...
Questions -
How to flatten a list in Python?
You can flatten a list in python using the following one-liner: flat_list = [item for sublist in l for item in sublist] In the example above, l is the list of lists that is to be flattened. The pre...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us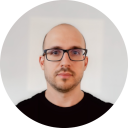
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github